Java算法移动开发:算法在移动开发中的应用,提升用户体验
发布时间: 2024-08-28 03:24:37 阅读量: 28 订阅数: 37 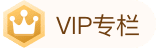
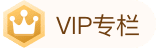
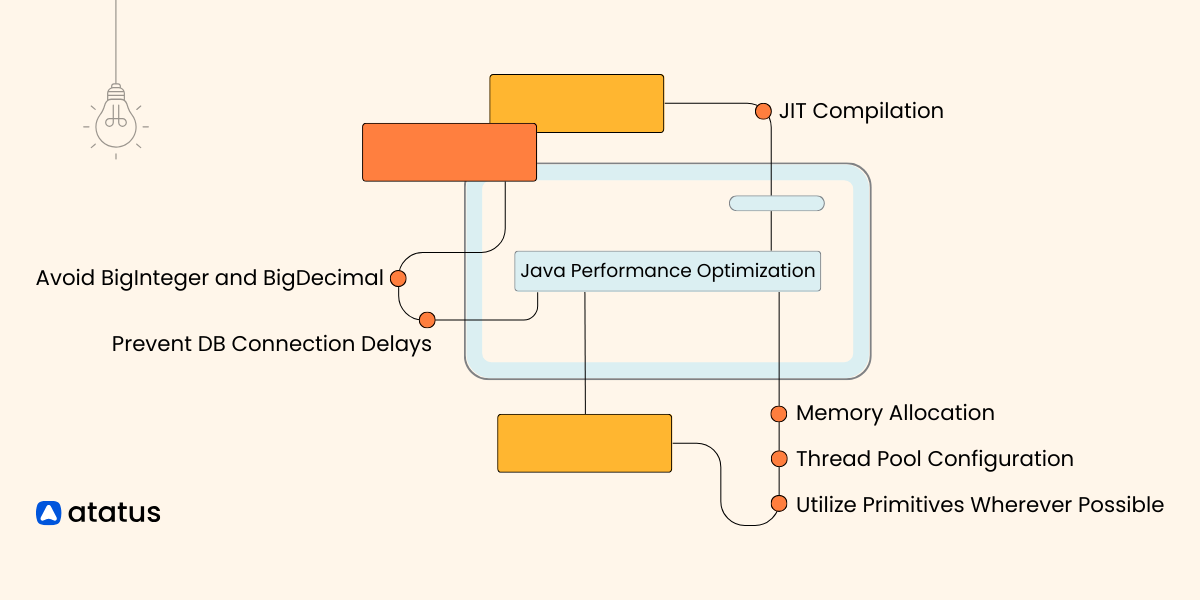
# 1. 移动开发中的算法基础**
算法是计算机科学的基础,它提供了解决问题和执行任务的步骤。在移动开发中,算法被广泛用于优化性能、增强用户体验和实现复杂功能。
理解算法的基础对于移动开发人员至关重要。这包括了解算法的复杂度、数据结构和算法设计模式。复杂度分析有助于确定算法在不同输入规模下的性能。数据结构提供了组织和存储数据的有效方法,而算法设计模式提供了一组可重用的解决方案来解决常见问题。
# 2. 算法在移动开发中的应用
算法在移动开发中发挥着至关重要的作用,为用户提供更流畅、更直观、更个性化的体验。本章节将深入探讨算法在移动开发中的广泛应用,从路径规划到数据结构、图像处理等方面进行详细分析。
### 2.1 路径规划算法
路径规划算法是移动开发中必不可少的工具,它可以帮助用户在复杂环境中找到最佳路径。最常用的路径规划算法包括:
#### 2.1.1 Dijkstra算法
Dijkstra算法是一种贪婪算法,用于寻找从给定起点到所有其他节点的最短路径。算法从起点开始,逐步扩展到相邻节点,并更新每个节点的最小距离。
```java
// Dijkstra算法实现
public class Dijkstra {
private final List<Vertex> vertices;
private final List<Edge> edges;
public Dijkstra(List<Vertex> vertices, List<Edge> edges) {
this.vertices = vertices;
this.edges = edges;
}
public Map<Vertex, Integer> computeShortestPaths(Vertex start) {
// 初始化距离表
Map<Vertex, Integer> distances = new HashMap<>();
for (Vertex vertex : vertices) {
distances.put(vertex, Integer.MAX_VALUE);
}
distances.put(start, 0);
// 优先队列,按距离排序
PriorityQueue<Vertex> queue = new PriorityQueue<>(Comparator.comparing(distances::get));
queue.add(start);
while (!queue.isEmpty()) {
Vertex current = queue.poll();
// 遍历当前节点的所有相邻节点
for (Edge edge : current.getEdges()) {
Vertex neighbor = edge.getDestination();
int newDistance = distances.get(current) + edge.getWeight();
// 如果新距离更短,则更新距离表
if (newDistance < distances.get(neighbor)) {
distances.put(neighbor, newDistance);
queue.add(neighbor);
}
}
}
return distances;
}
}
```
**逻辑分析:**
* 算法首先初始化距离表,将所有节点的距离设置为无穷大,并设置起点距离为0。
* 然后使用优先队列对节点进行排序,按距离从小到大排列。
* 算法从优先队列中取出距离最小的节点,并更新其相邻节点的距离。
* 如果新距离更短,则更新距离表并将其相邻节点添加到优先队列中。
* 算法重复此过程,直到优先队列为空。
#### 2.1.2 A*算法
A*算法是一种启发式搜索算法,它结合了 Dijkstra算法和贪婪搜索算法。A*算法使用启发函数来估计从当前节点到目标节点的剩余距离,从而提高搜索效率。
```java
// A*算法实现
public class AStar {
private final List<Vertex> vertices;
private final List<Edge> edges;
private final HeuristicFunction heuristicFunction;
public AStar(List<Vertex> vertices, List<Edge> edges, HeuristicFunction heuristicFunction) {
this.vertices = vertices;
this.edges = edges;
this.heuristicFunction = heuristicFunction;
}
public Map<Vertex, Integer> computeShortestPaths(Vertex start, Vertex goal) {
// 初始化距离表
Map<Vertex, Integer> distances = new HashMap<>();
for (Vertex vertex : vertices) {
distances.put(vertex, Integer.MAX_VALUE);
}
distances.put(start, 0);
// 优先队列,按 f(n) = g(n) + h(n) 排序
PriorityQueue<Vertex> queue = new PriorityQueue<>(Comparator.comparing(vertex -> distances.get(vertex) + heuristicFunction.estimate(vertex, goal)));
queue.add(start);
while (!queue.isEmpty()) {
Vertex current = queue.poll();
// 如果当前节点为目标节点,则返回距离表
if (current.equals(goal)) {
return distances;
}
// 遍历当前节点的所有相邻节点
for (Edge edge :
```
0
0
相关推荐







