Java算法设计模式:巧用模式,算法设计更优雅
发布时间: 2024-08-28 03:01:00 阅读量: 50 订阅数: 31 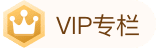
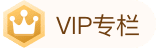
# 1. 算法设计模式概述
算法设计模式是一组可重用的解决方案,用于解决软件设计中常见的算法问题。它们提供了可扩展、可维护和高效的代码结构,使开发人员能够专注于解决特定问题,而不是重复解决通用算法问题。
设计模式根据其目的和结构分为三类:创建型模式、结构型模式和行为型模式。创建型模式专注于对象创建,结构型模式处理对象之间的关系,而行为型模式定义对象之间的通信和交互。
# 2. Java算法设计模式的分类与应用
算法设计模式是软件开发中常用的最佳实践,它提供了一种可重用的解决方案,用于解决常见软件设计问题。Java算法设计模式根据其用途和解决的问题类型分为三类:创建型模式、结构型模式和行为型模式。
### 2.1 创建型模式
创建型模式用于创建对象,它们提供了一种灵活的方式来实例化对象,而无需指定具体的类。
#### 2.1.1 工厂模式
工厂模式提供了一个接口,用于创建对象,而不必指定具体的类。它允许您在不修改客户端代码的情况下更改创建对象的类。
**代码块:**
```java
// 工厂接口
interface Factory {
Product createProduct();
}
// 具体工厂
class ConcreteFactory1 implements Factory {
@Override
public Product createProduct() {
return new Product1();
}
}
class ConcreteFactory2 implements Factory {
@Override
public Product createProduct() {
return new Product2();
}
}
// 产品接口
interface Product {
void doSomething();
}
// 具体产品
class Product1 implements Product {
@Override
public void doSomething() {
System.out.println("Product1");
}
}
class Product2 implements Product {
@Override
public void doSomething() {
System.out.println("Product2");
}
}
// 客户端代码
class Client {
private Factory factory;
public Client(Factory factory) {
this.factory = factory;
}
public Product createProduct() {
return factory.createProduct();
}
}
public class Main {
public static void main(String[] args) {
Factory factory1 = new ConcreteFactory1();
Client client1 = new Client(factory1);
Product product1 = client1.createProduct();
product1.doSomething(); // 输出:Product1
Factory factory2 = new ConcreteFactory2();
Client client2 = new Client(factory2);
Product product2 = client2.createProduct();
product2.doSomething(); // 输出:Product2
}
}
```
**逻辑分析:**
* 工厂接口定义了一个创建产品的方法。
* 具体工厂类实现了工厂接口,并创建特定类型的产品。
* 产品接口定义了产品的方法。
* 具体产品类实现了产品接口,并提供了实际的实现。
* 客户端代码通过工厂接口创建产品,而无需指定具体的类。
#### 2.1.2 建造者模式
建造者模式将一个复杂对象的构建与它的表示分离。它允许您使用不同的表示构建同一对象。
**代码块:**
```java
// 建造者接口
interface Builder {
void buildPartA();
void buildPartB();
void buildPartC();
Product getResult();
}
// 具体建造者
class ConcreteBuilder implements Builder {
private Product product = new Product();
@Override
public void buildPartA() {
product.addPart("PartA");
}
@Override
public void buildPartB() {
product.addPart("PartB");
}
@Override
public void buildPartC() {
product.addPart("PartC");
}
@Override
public Product getResult() {
return product;
}
}
// 指导者类
class Director {
private Builder builder;
public Director(Builder builder) {
this.builder = builder;
}
public Product construct() {
builder.buildPartA();
builder.buildPartB();
builder.buildPartC();
return builder.getResult();
}
}
// 产品类
class Product {
private List<String> parts = new ArrayList<>();
public void addPart(String part) {
parts.add(part);
}
@Override
public String toString() {
return "Product{" +
"parts=" + parts +
'}';
}
}
public class Main {
public static void main(String[] args) {
Builder builder = new ConcreteBuilder();
Director director = new Director(builder);
Product product = director.construct();
System.out.println(product); // 输出:Product{parts=[PartA, PartB, PartC]}
}
}
```
**逻辑分析:**
* 建造者接口定义了构建产品的方法。
* 具体建造者类实现了建造者接口,并创建特定类型的产品。
* 指导者类负责协调建造过程。
* 产品类表示最终构建的产品。
* 客户端代码通过指导者类构建产品,而无需直接与具体建造者交互。
#### 2.1.3 单例模式
单例模式确保一个类只有一个实例,并提供一个全局访问点。它用于创建单例对象,如配置对象、日志记录器或缓存。
**代码块:**
```java
public class Singleton {
private static volatile Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
```
**逻辑分析:**
* 单例类定义了一个私有构造函数,以防止直接实例化。
* getInstance()方法返回单例实例。如果实例不存在,它将使用双重检查锁定机制创建实例。
* 双重检查锁定机制确保线程安全,同时避免不必要的同步。
# 3.1.1 快速排序
**快速排序**是一种基于分治思想的排序算法,其基本思想是:
1. 从数组中选择一个元素作为枢纽元素。
2. 将数组中所有小于枢纽元素的元素放在枢纽元素的左边,所有大于枢纽元素的元素放在枢纽元素的右边。
3. 对枢纽元素左边的子数组和右边的子数组分别进行快速排序。
**代码实现:**
```java
public static void quickSort(int[] arr, int left, int right) {
if (left >= right) {
return;
}
int pivot = arr[right];
int i = left - 1;
for (int j = left; j < right; j++) {
if (arr[j] < pivot) {
i++;
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[i + 1];
arr[i + 1] = arr[right];
arr[right] = temp;
quickSort(arr, left, i);
quickSort(arr, i + 2, right);
}
```
**逻辑分析:**
* `quickSort`方法接收三个参数:数组`arr`、左边界`left`和右边界`right`。
* 如果`left`大于等于`right`,说明数组只有一个元素或为空,直接返回。
* 选择数组最右边的元素作为枢纽元素`pivot`。
* 初始化一个指针`i`为`left`- 1。
* 遍历数组,将所有小于`pivot`的元素移动到`i`的左边。
* 将`pivot`元素移动到`i + 1`的位置。
* 对`pivot`元素左边的子数组和右边的子数组分别进行快速排序。
**参数说明:**
* `arr`:需要排序的数组。
* `left`:数组的左边界。
* `right`:数组的右边界。
### 3.1.2 归并排序
**归并排序**也是一种基于分治思想的排序算法,其基本思想是:
1. 将数组分成两个大小相等的子数组。
2. 对这两个子数组分别进行归并排序。
3. 将排好序的两个子数组合并成一个排好序的数组。
**代码实现:**
```java
public static void mergeSort(int[] arr, int left, int right) {
if (left >= right) {
return;
}
int mid = (left + right) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
private static void merge(int[] arr, int left, int mid, int right) {
int[] temp = new int[right - left + 1];
int i = left;
int j = mid + 1;
int k = 0;
while (i <= mid && j <= right) {
if (arr[i] < arr[j]) {
temp[k++] = arr[i++];
} else {
temp[k++] = arr[j++];
}
}
while (i <= mid) {
temp[k++] = arr[i++];
}
while (j <= right) {
temp[k++] = arr[j++];
}
for (int m = 0; m < temp.length; m++) {
arr[left + m] = temp[m];
}
}
```
**逻辑分析:**
* `mergeSort`方法接收三个参数:数组`arr`、左边界`left`和右边界`right`。
* 如果`left`大于等于`right`,说明数组只有一个元素或为空,直接返回。
* 计算数组的中间位置`mid`。
* 对`arr`数组的左半部分和右半部分分别进行归并排序。
* 调用`merge`方法将排好序的两个子数组合并成一个排好序的数组。
**参数说明:**
* `arr`:需要排序的数组。
* `left`:数组的左边界。
* `right`:数组的右边界。
### 3.1.3 堆排序
**堆排序**是一种基于堆数据结构的排序算法,其基本思想是:
1. 将数组构建成一个最大堆(或最小堆)。
2. 将堆顶元素与最后一个元素交换,然后重新调整堆。
3. 重复步骤2,直到堆中只剩下一个元素。
**代码实现:**
```java
public static void heapSort(int[] arr) {
int len = arr.length;
buildMaxHeap(arr, len);
for (int i = len - 1; i >= 1; i--) {
int temp = arr[0];
arr[0] = arr[i];
arr[i] = temp;
heapify(arr, i, 0);
}
}
private static void buildMaxHeap(int[] arr, int len) {
for (int i = len / 2 - 1; i >= 0; i--) {
heapify(arr, len, i);
}
}
private static void heapify(int[] arr, int len, int i) {
int largest = i;
int left = 2 * i + 1;
int right = 2 * i + 2;
if (left < len && arr[left] > arr[largest]) {
largest = left;
}
if (right < len && arr[right] > arr[largest]) {
largest = right;
}
if (largest != i) {
int temp = arr[i];
arr[i] = arr[largest];
arr[largest] = temp;
heapify(arr, len, largest);
}
}
```
**逻辑分析:**
* `heapSort`方法接收一个数组`arr`作为参数。
* 调用`buildMaxHeap`方法将数组构建成一个最大堆。
* 遍历数组,将堆顶元素与最后一个元素交换,然后重新调整堆。
* 重复步骤2,直到堆中只剩下一个元素。
**参数说明:**
* `arr`:需要排序的数组。
**时间复杂度:**
* 平均时间复杂度:O(n log n)
* 最坏时间复杂度:O(n log n)
* 最好时间复杂度:O(n)
# 4. Java算法设计模式的性能优化
### 4.1 时间复杂度分析
#### 4.1.1 大O表示法
大O表示法是一种用于描述算法时间复杂度的渐近分析方法。它表示算法在输入规模趋于无穷大时,执行时间的上界。大O表示法使用以下符号:
* **O(f(n)):**算法的时间复杂度为f(n)的渐近上界。这意味着算法在最坏情况下执行时间不会超过f(n)。
* **Ω(f(n)):**算法的时间复杂度为f(n)的渐近下界。这意味着算法在最好情况下执行时间不会低于f(n)。
* **Θ(f(n)):**算法的时间复杂度为f(n)的渐近紧确界。这意味着算法在最坏和最好情况下执行时间都为f(n)。
#### 4.1.2 常见算法的时间复杂度
下表列出了几种常见算法的时间复杂度:
| 算法 | 时间复杂度 |
|---|---|
| 顺序查找 | O(n) |
| 二分查找 | O(log n) |
| 插入排序 | O(n^2) |
| 快速排序 | O(n log n) |
| 归并排序 | O(n log n) |
| 堆排序 | O(n log n) |
### 4.2 空间复杂度分析
#### 4.2.1 空间复杂度的概念
空间复杂度表示算法在执行过程中所需的内存空间量。它通常表示为算法在最坏情况下所需的最大内存空间。空间复杂度受以下因素影响:
* **数据结构:**算法使用的数据结构会影响其空间复杂度。例如,数组和链表的空间复杂度不同。
* **递归调用:**递归算法可能会导致堆栈空间消耗过大,从而增加空间复杂度。
* **临时变量:**算法中使用的临时变量也会增加空间复杂度。
#### 4.2.2 常见算法的空间复杂度
下表列出了几种常见算法的空间复杂度:
| 算法 | 空间复杂度 |
|---|---|
| 顺序查找 | O(1) |
| 二分查找 | O(1) |
| 插入排序 | O(n) |
| 快速排序 | O(log n) |
| 归并排序 | O(n) |
| 堆排序 | O(n) |
### 优化算法性能
#### 时间复杂度优化
* **选择合适的算法:**对于给定的问题,选择时间复杂度最优的算法。例如,对于有序数组,使用二分查找比顺序查找更有效率。
* **减少循环次数:**优化循环条件和步长,以减少循环次数。
* **使用数据结构:**使用合适的データ结构,例如哈希表或树,可以提高查找和插入操作的效率。
* **并行化:**对于某些算法,可以通过并行化来提高执行速度。
#### 空间复杂度优化
* **避免不必要的变量:**仅声明和使用必要的变量,以减少内存消耗。
* **使用引用而不是复制:**对于大对象,使用引用而不是复制可以节省内存空间。
* **使用内存池:**对于频繁创建和销毁的对象,使用内存池可以减少内存分配和释放的开销。
* **优化数据结构:**选择空间复杂度最优的数据结构,例如使用稀疏数组或跳表。
### 性能分析工具
* **Java Profiler:**Java Profiler是一种用于分析Java应用程序性能的工具。它可以显示代码执行时间、内存使用情况和线程活动。
* **JMH(Java Microbenchmark Harness):**JMH是一个用于进行微基准测试的框架。它可以帮助识别代码中的性能瓶颈。
* **YourKit Java Profiler:**YourKit Java Profiler是一个商业工具,提供高级性能分析功能,例如内存泄漏检测和线程分析。
# 5.1 算法设计模式的组合与扩展
### 5.1.1 模式组合的原则
算法设计模式的组合是一种将多个设计模式组合在一起,以创建更复杂和可重用的解决方案的技术。模式组合遵循以下原则:
- **独立性:**每个模式应该保持独立,以便可以单独使用或与其他模式组合。
- **协同性:**组合的模式应该协同工作,以实现比单独使用时更强大的功能。
- **可扩展性:**模式组合应该允许轻松添加或删除模式,以适应不断变化的需求。
### 5.1.2 模式扩展的案例
模式扩展是指修改或创建新的设计模式,以满足特定需求。以下是模式扩展的一个案例:
**装饰器模式的扩展:**
装饰器模式可以用来在不修改原始对象的情况下向对象添加功能。然而,在某些情况下,我们需要向原始对象添加不可逆或永久性的功能。为此,我们可以扩展装饰器模式,创建一个**不可变装饰器**。
不可变装饰器通过创建一个原始对象的副本并向副本添加功能来工作。这确保了原始对象不受修改,同时允许我们向对象添加永久性功能。
```java
public class ImmutableDecorator implements Shape {
private final Shape decoratedShape;
private final String additionalFunctionality;
public ImmutableDecorator(Shape decoratedShape, String additionalFunctionality) {
this.decoratedShape = decoratedShape;
this.additionalFunctionality = additionalFunctionality;
}
@Override
public void draw() {
decoratedShape.draw();
System.out.println("Additional functionality: " + additionalFunctionality);
}
}
```
在上面的示例中,`ImmutableDecorator`类扩展了`Shape`接口,并通过向`decoratedShape`对象添加`additionalFunctionality`来提供不可变的装饰。
## 5.2 算法设计模式的创新与应用
### 5.2.1 新型算法设计模式的探索
算法设计模式是一个不断发展的领域,新的模式不断被创建和探索。以下是一些新型算法设计模式:
- **管道模式:**管道模式将多个处理阶段连接成一个流水线,允许数据从一个阶段流向另一个阶段,同时保持松散耦合。
- **反应模式:**反应模式允许对象对事件做出反应,而无需显式地轮询事件。这在构建响应式和异步应用程序时非常有用。
- **责任链模式:**责任链模式将请求传递给一系列处理程序,每个处理程序都有机会处理请求或将其传递给下一个处理程序。这在构建可扩展和可维护的事件处理系统时非常有用。
### 5.2.2 算法设计模式在不同领域的应用
算法设计模式不仅在软件开发中有用,而且还在其他领域有广泛的应用,例如:
- **机器学习:**算法设计模式用于构建机器学习模型,如决策树、支持向量机和神经网络。
- **数据分析:**算法设计模式用于处理和分析大型数据集,如MapReduce和Spark。
- **金融:**算法设计模式用于构建金融模型,如风险管理和投资优化。
# 6. Java算法设计模式的学习与应用指南
### 6.1 算法设计模式的学习方法
#### 6.1.1 理论基础的掌握
- 理解设计模式的定义、分类和应用场景。
- 熟练掌握面向对象编程(OOP)概念,如封装、继承和多态。
- 了解数据结构和算法的基本原理,如数组、链表、栈和队列。
#### 6.1.2 实践案例的分析
- 通过分析真实世界的代码示例,理解算法设计模式的实际应用。
- 尝试自己实现算法设计模式,加深对概念的理解。
- 参加在线课程或研讨会,向经验丰富的开发者学习。
### 6.2 算法设计模式的应用场景
#### 6.2.1 复杂算法的抽象与重用
- 使用算法设计模式将复杂算法抽象为可重用的模块。
- 避免重复编写相同的代码,提高开发效率。
- 例如,使用策略模式将不同的排序算法封装为可互换的策略。
#### 6.2.2 代码的可读性与可维护性提升
- 算法设计模式有助于组织和结构代码,使其更易于理解和维护。
- 通过将相关代码分组到不同的类或模块中,提高代码的可读性。
- 例如,使用建造者模式创建复杂对象,避免创建嵌套或难以理解的代码。
0
0
相关推荐
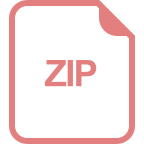
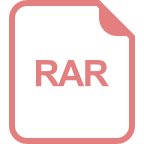
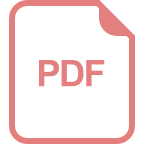
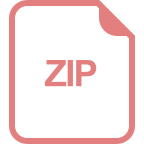
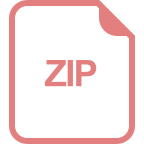
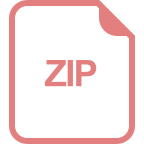
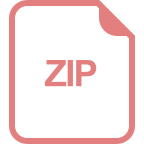
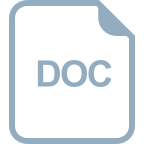
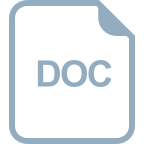