【单片机排序算法优化指南】:揭秘从冒泡排序到快速排序的性能提升秘诀
发布时间: 2024-07-11 05:59:57 阅读量: 45 订阅数: 38 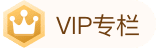
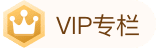
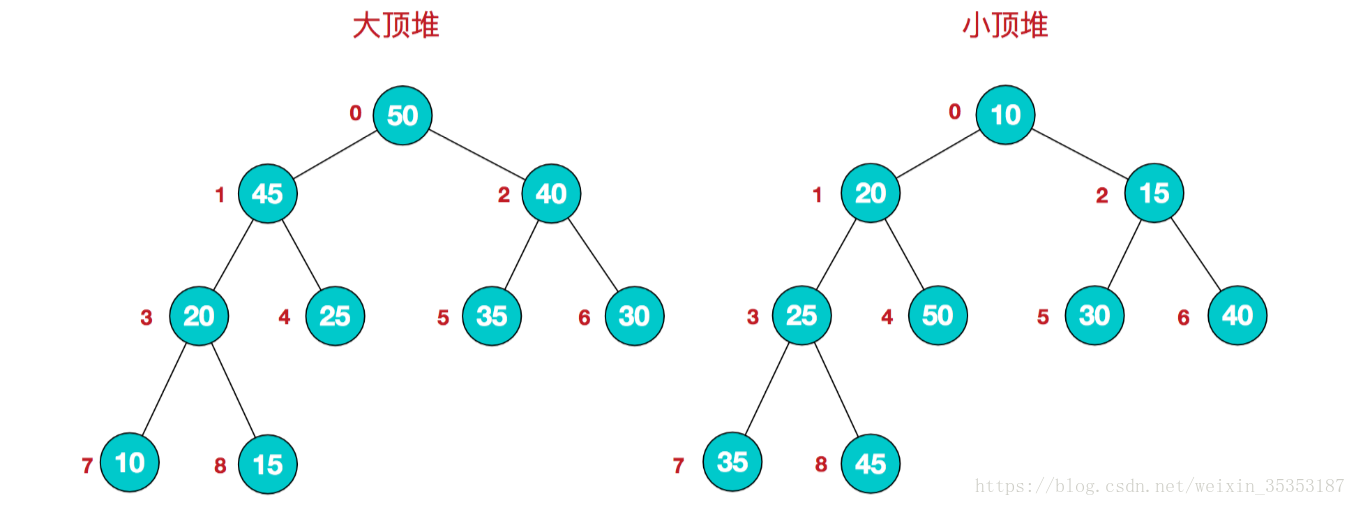
# 1. 单片机排序算法基础**
单片机排序算法是一种用于对单片机中的数据进行排序的算法。排序算法的目的是将数据元素按照特定的顺序排列,例如升序或降序。排序算法在单片机系统中广泛应用于数据管理、控制系统和通信协议优化等领域。
排序算法的性能至关重要,因为它影响着单片机系统的整体效率。排序算法的性能主要由时间复杂度和空间复杂度决定。时间复杂度衡量算法执行所需的时间,而空间复杂度衡量算法执行所需的空间。
# 2. 排序算法性能优化原理
排序算法的性能优化是算法设计中的关键问题,因为它直接影响到算法在实际应用中的效率。本章节将深入分析排序算法的性能优化原理,包括算法复杂度分析和优化策略。
### 2.1 算法复杂度分析
算法复杂度分析是评估算法性能的重要指标,它描述了算法在不同输入规模下的时间和空间开销。
#### 2.1.1 时间复杂度
时间复杂度表示算法执行所花费的时间,通常用大 O 符号表示。常见的时间复杂度包括:
* O(1):常数时间,与输入规模无关
* O(log n):对数时间,随着输入规模的增加,时间开销以对数形式增长
* O(n):线性时间,时间开销与输入规模成正比
* O(n^2):平方时间,时间开销与输入规模的平方成正比
#### 2.1.2 空间复杂度
空间复杂度表示算法执行所需要的内存空间,通常也用大 O 符号表示。常见的空间复杂度包括:
* O(1):常数空间,与输入规模无关
* O(n):线性空间,空间开销与输入规模成正比
* O(n^2):平方空间,空间开销与输入规模的平方成正比
### 2.2 优化策略
排序算法的优化策略主要包括数据结构优化和算法改进。
#### 2.2.1 数据结构优化
数据结构优化通过选择合适的存储结构来提高算法的性能。例如:
* 使用数组或链表存储数据,可以优化空间开销
* 使用二叉树或哈希表存储数据,可以优化查询时间
#### 2.2.2 算法改进
算法改进通过修改算法的执行逻辑来提高性能。例如:
* 使用快速排序或归并排序等分治算法,可以降低时间复杂度
* 使用插入排序或选择排序等简单算法,可以优化空间复杂度
### 代码示例
以下代码示例展示了冒泡排序算法的性能优化:
```c
// 原版冒泡排序
void bubble_sort(int *arr, int len) {
for (int i = 0; i < len - 1; i++) {
for (int j = 0; j < len - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
// 优化后的冒泡排序
void bubble_sort_optimized(int *arr, int len) {
int swapped = 1;
while (swapped) {
swapped = 0;
for (int j = 0; j < len - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
swapped = 1;
}
}
}
}
```
优化后的冒泡排序通过引入 `swapped` 变量来记录是否发生交换,如果未发生交换,则说明数组已排序,可以提前终止排序过程,从而降低时间复杂度。
### 性能对比
下表对比了原版冒泡排序和优化后的冒泡排序的性能:
| 算法 | 时间复杂度 | 空间复杂度 |
|---|---|---|
| 原版冒泡排序 | O(n^2) | O(1) |
| 优化后的冒泡排序 | O(n) | O(1) |
从表中可以看出,优化后的冒泡排序在时间复杂度上得到了显著提升,从 O(n^2) 降低到了 O(n)。
# 3. 经典排序算法实践**
### 3.1 冒泡排序
#### 3.1.1 原理和实现
冒泡排序是一种简单的排序算法,它通过不断比较相邻元素,将较大的元素“冒泡”到数组末尾,直到整个数组有序。算法步骤如下:
```python
def bubble_sort(arr):
for i in range(len(arr) - 1):
for j in range(len(arr) - 1 - i):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
```
#### 3.1.2 性能优化
冒泡排序的时间复杂度为 O(n^2),其中 n 为数组长度。为了优化性能,可以采用以下策略:
- **提前退出:**如果某次遍历没有发生交换,说明数组已经有序,可以提前退出循环。
- **优化比较:**在每次比较前,先判断是否需要比较,例如相邻元素已经有序。
### 3.2 选择排序
#### 3.2.1 原理和实现
选择排序通过在未排序部分中找到最小元素,并将其与未排序部分的第一个元素交换,以此类推,直到整个数组有序。算法步骤如下:
```python
def selection_sort(arr):
for i in range(len(arr) - 1):
min_index = i
for j in range(i + 1, len(arr)):
if arr[j] < arr[min_index]:
min_index = j
arr[i], arr[min_index] = arr[min_index], arr[i]
```
#### 3.2.2 性能优化
选择排序的时间复杂度也为 O(n^2)。优化策略与冒泡排序类似:
- **提前退出:**如果某次遍历没有找到更小的元素,说明数组已经有序,可以提前退出循环。
- **优化比较:**在每次比较前,先判断是否需要比较,例如相邻元素已经有序。
### 3.3 插入排序
#### 3.3.1 原理和实现
插入排序通过将未排序部分的元素逐个插入到已排序部分中,保持已排序部分有序。算法步骤如下:
```python
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and arr[j] > key:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
```
#### 3.3.2 性能优化
插入排序的时间复杂度为 O(n^2),但对于已经部分有序的数组,其性能可以显著提高。优化策略如下:
- **二分查找:**在已排序部分中使用二分查找来找到插入位置,减少比较次数。
- **哨兵元素:**在已排序部分开头添加一个哨兵元素,避免边界检查。
# 4.1 归并排序
### 4.1.1 原理和实现
归并排序是一种分治算法,它将待排序的数组分成较小的子数组,对子数组进行排序,然后合并这些有序的子数组以得到最终的排序结果。
归并排序的实现步骤如下:
1. **递归分解:**将待排序的数组分成大小相等的两个子数组,直到每个子数组只有一个元素或为空。
2. **递归排序:**对每个子数组递归地应用归并排序算法。
3. **合并:**将排序好的子数组合并成一个有序的数组。
```python
def merge_sort(arr):
"""
归并排序算法
参数:
arr: 待排序的数组
返回:
排序后的数组
"""
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left_half = merge_sort(arr[:mid])
right_half = merge_sort(arr[mid:])
return merge(left_half, right_half)
def merge(left, right):
"""
合并两个有序数组
参数:
left: 有序的左半数组
right: 有序的右半数组
返回:
合并后的有序数组
"""
merged = []
left_index = 0
right_index = 0
while left_index < len(left) and right_index < len(right):
if left[left_index] <= right[right_index]:
merged.append(left[left_index])
left_index += 1
else:
merged.append(right[right_index])
right_index += 1
merged.extend(left[left_index:])
merged.extend(right[right_index:])
return merged
```
### 4.1.2 性能优化
归并排序的平均时间复杂度为 O(n log n),最坏时间复杂度也为 O(n log n),空间复杂度为 O(n)。
以下是一些优化归并排序性能的方法:
* **使用哨兵元素:**在合并过程中,添加一个哨兵元素到每个子数组的末尾,哨兵元素的值大于所有其他元素。这可以简化合并过程,因为不需要再检查数组是否越界。
* **自底向上归并:**而不是递归地调用归并排序,可以自底向上地进行合并,从较小的子数组开始合并,逐步合并成更大的子数组。
* **并行归并:**如果可用,可以使用多线程或多进程来并行执行归并操作,从而提高性能。
# 5. 单片机排序算法应用
排序算法在单片机系统中有着广泛的应用,可以有效地优化数据处理效率和系统性能。以下列举几个典型应用场景:
### 5.1 数据采集和处理
在单片机系统中,经常需要采集和处理大量数据,例如传感器数据、通信数据等。排序算法可以对这些数据进行排序,以便于后续的分析和处理。例如,可以对传感器数据进行排序,找出最大值或最小值,从而判断传感器状态。
```c
// 数据采集和排序
uint16_t sensor_data[100];
// 冒泡排序
for (int i = 0; i < 100; i++) {
for (int j = 0; j < 99 - i; j++) {
if (sensor_data[j] > sensor_data[j + 1]) {
uint16_t temp = sensor_data[j];
sensor_data[j] = sensor_data[j + 1];
sensor_data[j + 1] = temp;
}
}
}
// 获取最大值
uint16_t max_value = sensor_data[99];
```
### 5.2 控制系统优化
在单片机控制系统中,排序算法可以用于优化控制策略。例如,在电机控制系统中,可以对电机转速数据进行排序,找出最优转速,从而提高控制精度。
```c
// 电机转速数据排序
uint16_t motor_speed[100];
// 快速排序
int partition(uint16_t arr[], int low, int high) {
uint16_t pivot = arr[high];
int i = (low - 1);
for (int j = low; j <= high - 1; j++) {
if (arr[j] < pivot) {
i++;
uint16_t temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
uint16_t temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return (i + 1);
}
void quick_sort(uint16_t arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quick_sort(arr, low, pi - 1);
quick_sort(arr, pi + 1, high);
}
}
// 获取最优转速
uint16_t optimal_speed = motor_speed[50]; // 假设最优转速位于数组中间
```
### 5.3 通信协议优化
在单片机通信系统中,排序算法可以用于优化通信协议。例如,在数据包传输中,可以对数据包大小进行排序,优先传输较小的数据包,从而提高传输效率。
```c
// 数据包大小排序
uint16_t packet_size[100];
// 归并排序
void merge(uint16_t arr[], int l, int m, int r) {
int i, j, k;
int n1 = m - l + 1;
int n2 = r - m;
uint16_t L[n1], R[n2];
for (i = 0; i < n1; i++)
L[i] = arr[l + i];
for (j = 0; j < n2; j++)
R[j] = arr[m + 1 + j];
i = 0;
j = 0;
k = l;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
void merge_sort(uint16_t arr[], int l, int r) {
if (l < r) {
int m = l + (r - l) / 2;
merge_sort(arr, l, m);
merge_sort(arr, m + 1, r);
merge(arr, l, m, r);
}
}
// 获取最小的数据包大小
uint16_t min_packet_size = packet_size[0];
```
0
0
相关推荐
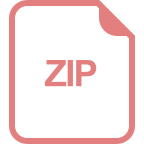
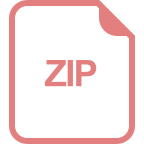
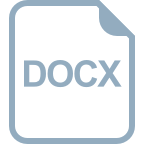
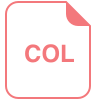
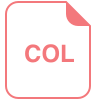
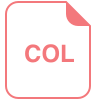
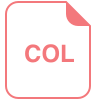

