近似算法在运筹学中的应用:提升决策效率与优化,助你做出明智的决策
发布时间: 2024-08-25 01:41:05 阅读量: 64 订阅数: 45 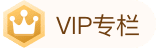
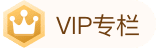
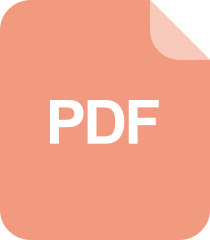
C语言实现运筹学中的马氏决策算法实例
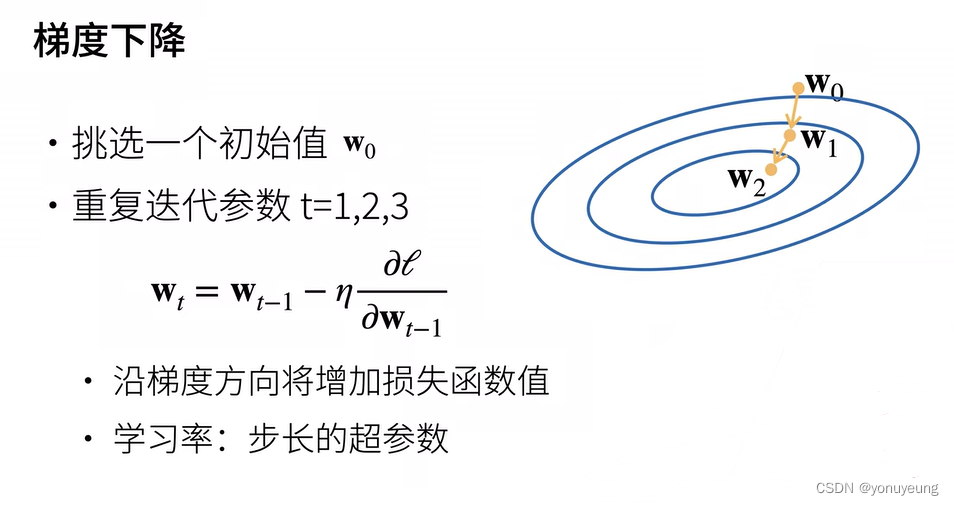
# 1. 近似算法在运筹学中的概述**
近似算法是一种在多项式时间内求解 NP 困难问题的算法,它不能保证找到最优解,但可以找到一个近似最优解,且近似比(近似解与最优解之比)有界。
近似算法在运筹学中广泛应用,尤其是在解决组合优化问题和连续优化问题时。组合优化问题包括旅行商问题和背包问题,而连续优化问题包括线性规划和非线性规划。
# 2. 近似算法的理论基础**
近似算法是运筹学中用于解决复杂优化问题的有效工具。本章将深入探讨近似算法的理论基础,包括其定义、分类、分析方法和证明近似比。
## 2.1 近似算法的定义和分类
### 2.1.1 近似比和近似因子
近似算法是一种不能保证找到最优解的算法,但它能提供一个近似解,该近似解与最优解之间的误差在可接受范围内。近似比定义为近似解与最优解之比,它衡量了近似算法的性能。
近似因子是一个常数,它表示近似比的上界。例如,一个近似因子为 2 的算法保证找到的解与最优解之间的误差不会超过 100%。
### 2.1.2 贪心算法和启发式算法
贪心算法是一种在每一步都做出局部最优选择的算法。虽然贪心算法通常不能保证找到最优解,但它们通常可以提供良好的近似解。
启发式算法是一种基于经验和直觉设计的算法。它们通常不能提供近似比的保证,但它们可以在实践中有效地解决复杂问题。
## 2.2 近似算法的分析方法
### 2.2.1 性能分析和复杂度分析
近似算法的性能分析涉及评估其近似比和时间复杂度。近似比衡量算法的近似质量,而时间复杂度衡量算法运行所需的时间。
### 2.2.2 证明近似比
证明近似比是证明近似算法性能的关键步骤。有几种技术可以用于证明近似比,包括:
- **竞争分析:**将近似算法与一个简单的算法进行比较,该算法始终提供一个已知近似比的解。
- **线性规划松弛:**将优化问题松弛为一个线性规划问题,并证明近似算法的解与松弛解之间的误差。
- **对偶分析:**使用对偶性理论来证明近似算法的解与最优解之间的误差。
## 代码示例:
```python
def greedy_tsp(graph):
"""
使用贪心算法求解旅行商问题。
参数:
graph: 图的邻接矩阵。
返回:
一个近似旅行商路径。
"""
# 初始化未访问的顶点集合。
unvisited = set(range(len(graph)))
# 选择一个起始顶点。
current = unvisited.pop()
# 循环访问所有顶点。
while unvisited:
# 选择当前顶点到未访问顶点的最短边。
next = min(unvisited, key=lambda v: graph[current][v])
# 访问下一个顶点。
current = next
unvisited.remove(next)
# 返回旅行商路径。
return [current] + greedy_tsp(graph[current:])
```
**代码逻辑分析:**
该代码实现了贪心算法来求解旅行商问题。它从一个起始顶点开始,每次选择当前顶点到未访问顶点的最短边,并访问下一个顶点。这个过程一直持续到所有顶点都被访问。
**参数说明:**
* `graph`:图的邻接矩阵,其中 `graph[i][j]` 表示顶点 `i` 和 `j` 之间的距离。
# 3. 近似算法在运筹学中的实践应用
### 3.1 组合优化问题
组合优化问题是指在有限集合中找到满足特定目标函数的最佳解。近似算法在解决组合优化问题中发挥着重要作用,因为它可以在有限时间内找到接近最优解的解。
#### 3.1.1 旅行商问题
旅行商问题(TSP)是一个经典的组合优化问题,其目标是在一组城市中找到一条最短的路径,该路径访问每个城市一次并返回出发城市。
**贪心算法:**
一种常见的 TSP 近似算法是贪心算法,它在每次迭代中选择当前城市到未访问城市的最短路径。该算法简单易于实现,但不能保证找到最优解。
**代码块:**
```python
def greedy_tsp(cities):
"""
贪心算法求解旅行商问题。
参数:
cities: 城市列表。
返回:
最短路径。
"""
visited = set()
current_city = cities[0]
path = [current_city]
visited.add(current_city)
while len(visited) < len(cities):
min_distance = float('inf')
next_city = None
for city in cities:
if city not in visited and distance(current_city, city) < min_distance:
min_distance = distance(current_city, city)
next_city = city
path.append(next_city)
current_city = next_city
visited.add(current_city)
return path
```
**逻辑分析:**
该贪心算法首先将第一个城市标记为已访问,然后在每次迭代中选择当前城市到未访问城市的最短路径。该算法不断更新当前城市和已访问城市集合,直到所有城市都被访问。
#### 3.1.2 背包问题
背包问题是另一个常见的组合优化问题,其目标是在给定容量的背包中装入最多价值的物品。
**动态规划:**
一种解决背包问题的近似算法是动态规划,它将问题分解成较小的子问题,并使用表格存储子问题的最优解。
**代码块:**
```python
def knapsack_dp(items, capacity):
"""
动态规划求解背包问题。
参数:
items: 物品列表,每个物品有价值和重量。
capacity: 背包容量。
返回:
```
0
0
相关推荐






