【数据可视化基础】:用Python勾勒清晰故事线的7大技巧
发布时间: 2024-08-31 09:38:11 阅读量: 192 订阅数: 97 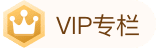
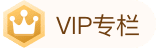
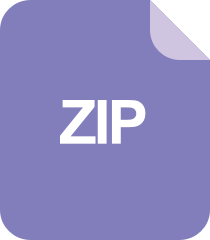
python爬虫数据可视化分析大作业.zip


# 1. 数据可视化的基础概念
数据可视化是将复杂的数据集转换成图形图像,以便观察者能够识别模式、趋势和关联,这些模式在原始数据中可能不是那么明显。它涉及到统计图形学、图形设计、信息可视化、科学可视化等多个领域。一个优秀的数据可视化不仅应该具备传达信息的功能,还应该具备吸引观众注意力的能力,并且要简洁明了,避免过度装饰导致的误导。
数据可视化的目的是为了更有效地传达信息,它可以通过以下方式实现:
- **简化复杂数据**:帮助人们理解庞大的数据集。
- **识别模式和趋势**:通过图形展示发现数据中隐藏的模式。
- **有效沟通**:将数据转化为容易理解的图表或图形,以便快速交流信息。
- **增强记忆**:图形信息比文本信息更容易被大脑处理和记忆。
接下来的章节将深入探讨数据可视化的设计原则、适用图表类型的选择、高级技巧以及如何将理论应用于实际项目中。
# 2. Python数据可视化工具简介
## 2.1 Matplotlib库的基础与应用
Matplotlib是Python中最古老且广泛使用的数据可视化库之一。它允许用户从简单的线图到复杂的3D图形进行广泛的定制。本节将探讨Matplotlib的安装、基本使用和一些高级特性。
### 2.1.1 安装Matplotlib
首先,你需要在你的Python环境中安装Matplotlib。可以通过pip安装它:
```bash
pip install matplotlib
```
### 2.1.2 Matplotlib的基本绘图
Matplotlib提供了`pyplot`模块,该模块提供了一个类似MATLAB的绘图系统,允许用户快速地绘制数据。
```python
import matplotlib.pyplot as plt
# 示例数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.plot(x, y)
plt.title('Simple Line Chart')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
plt.show()
```
在这个例子中,我们绘制了一个简单的线图,其中x和y是数据列表。`plot`函数创建了线图,`title`、`xlabel`和`ylabel`分别添加了图表的标题和坐标轴标签。`show()`函数用来显示图表。
### 2.1.3 Matplotlib的高级特性
Matplotlib不仅仅支持基本的绘图。它还可以定制图表的外观和行为。例如,你可以改变线条的颜色和样式,添加图例,改变坐标轴的刻度,等等。
```python
plt.plot(x, y, 'r--', label='Sample Data')
plt.plot([1, 5], [2, 14], 'b.-', label='Linear Fit')
plt.title('Advanced Line Chart')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
plt.legend()
plt.grid(True)
plt.show()
```
在这个例子中,我们使用了不同的颜色和线条样式来绘制数据,并添加了图例和网格线。`r--`表示红色虚线,而`b.-`表示蓝色点线。
Matplotlib还支持子图绘制,它允许在一个图窗口中创建多个图表。这对于比较多个数据集非常有用。
```python
fig, ax = plt.subplots(2, 1) # 创建两个子图
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
y2 = [4, 6, 9, 14, 18]
ax[0].plot(x, y)
ax[1].plot(x, y2)
ax[0].set_title('First Subplot')
ax[1].set_title('Second Subplot')
plt.tight_layout()
plt.show()
```
这里,`subplots(2, 1)`创建了一个2行1列的子图布局。每个子图都可以独立地进行绘制和定制。
## 2.2 Seaborn库的高级特性
虽然Matplotlib提供了强大的绘图功能,但Seaborn库在某些方面进一步简化了高级可视化类型,比如统计图表。Seaborn构建在Matplotlib之上,提供了额外的主题和视觉设置。
### 2.2.1 安装Seaborn
安装Seaborn跟安装Matplotlib类似:
```bash
pip install seaborn
```
### 2.2.2 Seaborn的统计可视化
Seaborn最著名的特性之一是它创建高级统计可视化的能力。
```python
import seaborn as sns
tips = sns.load_dataset('tips')
sns.barplot(x="day", y="total_bill", data=tips)
plt.title('Bar Plot of Total Bills by Day')
plt.xlabel('Day of the Week')
plt.ylabel('Total Bill')
plt.show()
```
在这个例子中,我们使用Seaborn的`barplot`函数来创建一个条形图,展示了餐馆小费数据按天的分布。
Seaborn也支持更复杂的统计图表,例如分布图和回归图。
```python
sns.jointplot(x='total_bill', y='tip', data=tips, kind='reg')
plt.suptitle('Joint Plot of Total Bill and Tip')
plt.show()
```
`jointplot`函数创建了一个联合图,展示了两个变量的关系,并附有各自的分布图。`kind='reg'`参数表示添加了线性回归线。
### 2.2.3 Seaborn的自定义和主题
Seaborn通过内置的主题和调色板支持快速的视觉定制,提高了美观程度。
```python
sns.set_theme(style="darkgrid")
sns.lineplot(x=x, y=y, data=tips)
plt.title('Line Plot with Seaborn')
plt.show()
```
在上述代码中,我们使用了`set_theme`函数来应用一个默认的Seaborn主题,这里的`style="darkgrid"`给图表带来了深色的网格背景。
总结来说,Matplotlib和Seaborn都是Python中强大的数据可视化工具。Matplotlib提供了底层的绘图功能,而Seaborn则在Matplotlib的基础上提供了一些额外的高级可视化和美观性定制。通过两者的组合使用,可以创建出既功能强大又视觉吸引人的数据可视化项目。
# 3. 选择合适的图表类型
在数据可视化中,选择恰当的图表类型对于有效传达信息至关重要。不同的数据类型和分析目标将指导我们选择最适合的可视化方式。本章节将详细介绍几种常见图表类型的应用场景,包括柱状图和条形图、折线图和面积图,以及散点图和气泡图。通过深入探讨它们的特点和使用方法,我们将帮助读者更好地选择和应用这些图表。
## 3.1 柱状图和条形图的应用
柱状图和条形图是数据可视化中最常见的图表类型,它们以条形的方式直观地展示不同类别的数值大小。尽管它们的外观相似,但二者在方向上有所区别:柱状图的条形是垂直的,而条形图的条形则是水平的。这两种图表通常用于比较不同类别的数据。
### 3.1.1 对比数据项
柱状图非常适合于展示和对比不同类别的数据项。通过条形的高度或长度,我们可以快速比较各个类别之间的数值差异。
```python
import matplotlib.pyplot as plt
# 示例数据
categories = ['Category A', 'Category B', 'Category C', 'Category D']
values = [10, 20, 30, 40]
# 创建柱状图
plt.figure(figsize=(8, 4))
plt.bar(categories, values)
# 添加图表标题和轴标签
plt.title('Comparison of Data Items')
plt.xlabel('Categories')
plt.ylabel('Values')
# 显示图表
plt.show()
```
在上述代码中,我们使用了Python的matplotlib库创建了一个柱状图。通过`plt.bar()`函数,我们定义了类别的列表和对应的数值。图表的`title`、`xlabel`和`ylabel`分别定义了图表标题和轴标签。
### 3.1.2 时间序列数据展示
柱状图也常用于展示时间序列数据,尤其是当你需要比较不同时期的数据量时。
```python
import pandas as pd
import matplotlib.dates as mdates
# 示例时间序列数据
dates = pd.date_range(start='2021-01-01', periods=4, freq='M')
values = [15, 25, 35, 45]
# 创建时间序列柱状图
plt.figure(figsize=(8, 4))
plt.bar(dates, values)
# 设置日期格式
plt.gca().xaxis.set_major_formatter(mdates.DateFormatter('%Y-%m'))
plt.gca().xaxis.set_major_locator(mdates.Mon
```
0
0
相关推荐
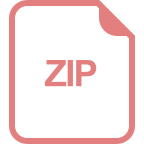
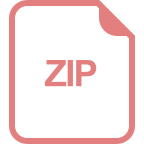
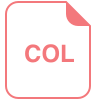
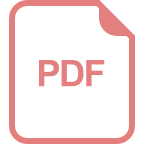
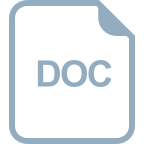
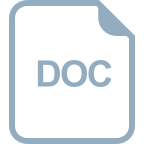
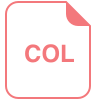
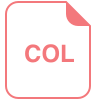