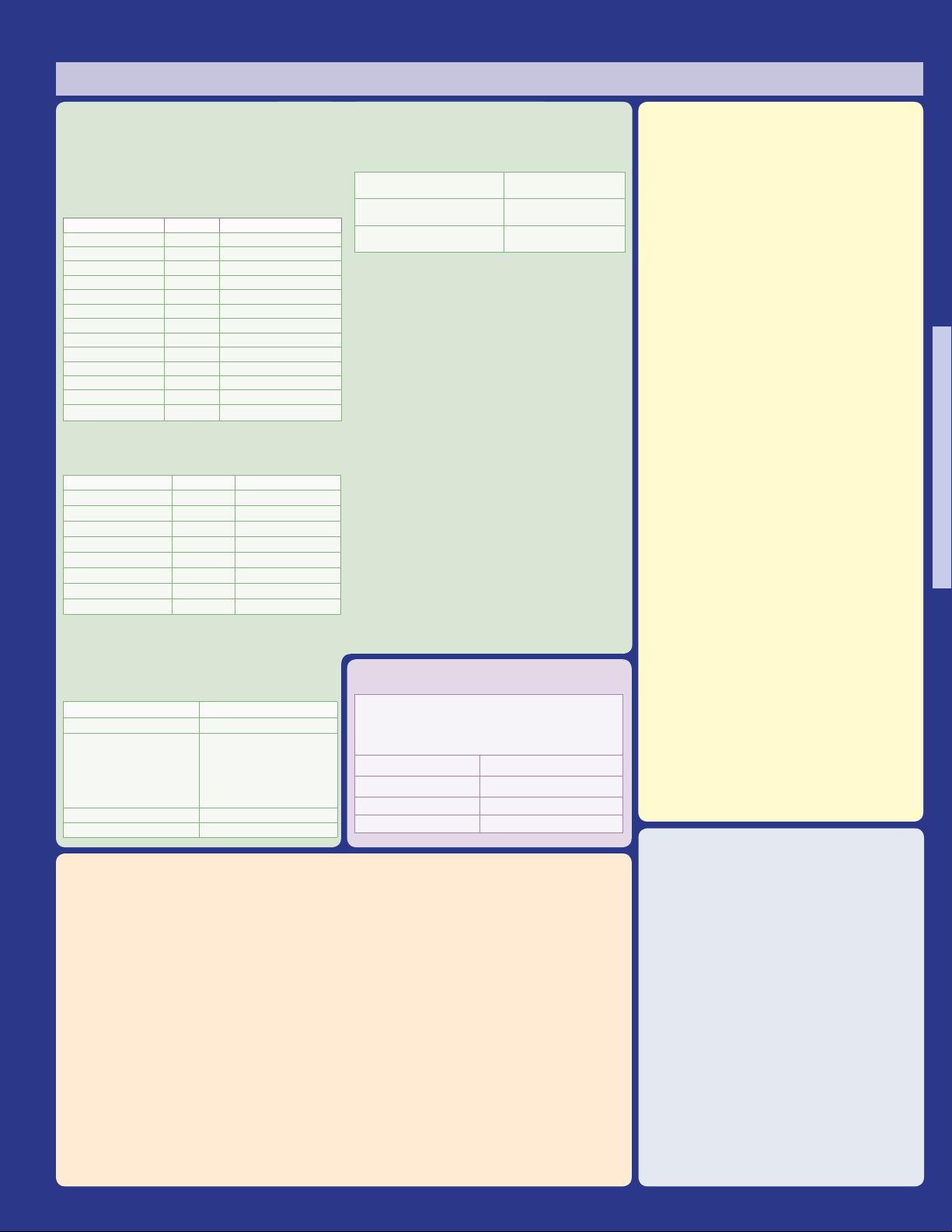
OpenCL 2.2 Reference Guide Page 5
©2017 Khronos Group - Rev. 0817 www.khronos.org/opencl
OpenCL C++ Language
OpenCL C++ Language
OpenCL C++ and C++ 14
The OpenCL C++ programming language is based on the
ISO/IEC JTC1 SC22 WG21 N3690 language (a.k.a. C++14)
specicaon with specic restricons and excepons.
Secon numbers denoted here with § refer to the C++ 14
specicaon.
• Implicit conversions for pointer types follow the rules
described in the C++ 14 specicaon.
• Conversions between integer types follow the conversion
rules specied in the C++14 specicaon except for
specic out-of-range behavior and saturated conversions.
• The preprocessing direcves dened by the C++14
specicaon are supported.
• Macro names dened by the C++14 specicaon but not
currently supported by OpenCL are reserved for future
use.
• OpenCL C++ standard library implements modied version
of the C++ 14 numeric limits library.
• OpenCL C++ implements the following parts of the C++ 14
iterator library: Primives, iterator operaons, predened
iterators, and range access.
• The OpenCL C++ kernel language doesn’t support variadic
funcons and variable length arrays.
• OpenCL C++ library implements most of the C++14 tuples
except for allocator related traits (§ 20.4.2.8).
• OpenCL C++ supports type traits dened in the C++ 14
specicaon with addions and changes to the following:
UnaryTypeTraits (§ 3.15.1)
BinaryTypeTraits (§ 3.15.2)
TransformaonTraits (§ 3.15.3)
• OpenCL C++ standard library implements most C++ 14
tuples excluding allocator related traits.
• C++14 features not supported by OpenCL C++:
the dynamic_cast operator (§ 5.2.7)
type idencaon (§ 5.2.8)
recursive funcon calls (§ 5.2.2, item 9) unless they are a
compile-me constant expression
non-placement new and delete operators (§ 5.3.4, 5.3.5)
goto statement (§ 6.6)
register and thread_local storage qualiers (§ 7.1.1)
virtual funcon qualier (§ 7.1.2)
funcon pointers (§ 8.3.5, 8.5.3) unless they are a
compile-me constant expression
virtual funcons and abstract classes (§ 10.3, 10.4)
excepon handling (§ 15)
the C++ standard library (§ 17 … 30)
asm declaraon (§ 7.4)
no implicit lambda to funcon pointer conversion (§ 5.1.2)
OpenCL C++ Language Reference
Secon and table references are to the OpenCL 2.2 C++ Language specicaon.
Qualiers and Oponal Aributes
Funcon Qualier [2.6.1]
__kernel, kernel
Type and Variable Aributes [2.8]
[[cl::aligned(X)]] [[cl::aligned]]
Species a minimum alignment (in bytes) for variables of the
specied type.
[[cl::packed]]
Species that each member of the structure or union is placed to
minimize the memory required.
Kernel Funcon Aributes [2.8.3]
[[cl::work_group_size_hint(X, Y, Z)]]
A hint to the compiler to specify the value most likely
to be specied by the local_work_size argument to
clEnqueueNDRangeKernel.
[[cl::required_work_group_size(X, Y, Z)]]
The work-group size that must be used as the local_work_size
argument to clEnqueueNDRangeKernel.
[[cl::required_num_sub_groups(X)]]
The number of sub-groups that must be generated by a kernel
launch.
[[cl::vec_type_hint(<type>)]]
A hint to the compiler as a representaon of the computaonal
width of the kernel.
Kernel Parameter Aribute [2.8.4]
[[cl::max_size(n)]]
The value of the aribute species the maximum size in bytes of
the corresponding memory object.
Loop Aributes [2.8.5]
[[cl::unroll_hint(n)]] [[cl::unroll_hint]]
Used to specify that a loop (for, while, and do loops) can be
unrolled.
[[cl::ivdep(len)]] [[cl::ivdep]]
A hint to indicate that the compiler may assume there are
no memory dependencies across loop iteraons in order to
autovectorize consecuve iteraons of loop.
Conversions and Reinterpretaon
Header <opencl_convert>
Conversion types [3.2]
Conversions are available for the scalar types bool, char,
uchar, short, ushort, int, uint, long, ulong, half (if cl_khr_fp16
extension is enabled), oat, double (if cl_khr_fp64 is enabled),
and derived vector types.
template <class T, rounding_mode rmode, class U>
T convert_cast(U const& arg);
template <class T, rounding_mode rmode>
T convert_cast(T const& arg);
// and more...
Rounding modes [3.2.3]
::rte to nearest even ::rtz toward zero
::rtp toward + innity ::rtn toward - innity
Reinterpreng types [3.3]
Header <opencl_reinterpret>
Supported data types except bool and void may be
reinterpreted as another data type of the same size using the
as_type funcon for scalar and vector data types.
template <class T, class U>
T as_type(U const& arg);
Preprocessor Direcves & Macros [2.7]
#pragma OPENCL FP_CONTRACT on-o-switch
on-o-switch: ON, OFF, or DEFAULT
#pragma OPENCL EXTENSION extensionname : behavior
#pragma OPENCL EXTENSION all : behavior
__FILE__ Current source le
__LINE__ Integer line number
__OPENCL_CPP_VERSION__ Integer version number, e.g: 100
__func__ Current funcon name
Supported Data Types [3.1]
Header <opencl_def>
cl_* types have exactly the same size as their host counterparts
dened in <cl_plaorm.h> le. Half types require cl_khr_fp16.
Double types require that cl_khr_fp64 be enabled and that
CL_DEVICE_DOUBLE_FP_CONFIG is not zero.
Built-in scalar data types
OpenCL Type API Type Descripon
bool -- true (1) or false (0)
char cl_char 8-bit signed
unsigned char, uchar cl_uchar 8-bit unsigned
short cl_short 16-bit signed
unsigned short, ushort cl_ushort 16-bit unsigned
int cl_int 32-bit signed
unsigned int, uint cl_uint 32-bit unsigned
long cl_long 64-bit signed
unsigned long, ulong cl_ulong 64-bit unsigned
oat cl_oat 32-bit oat
double cl_double 64-bit IEEE 754
half cl_half 16-bit oat (storage only)
void void empty set of values
Built-in vector data types
n is 2, 3, 4, 8, or 16. The halfn vector data type is required to be
supported as a data storage format.
OpenCL Type API Type Descripon
bool
n
[u]char
n
cl_[u]charn 8-bit [un]signed
[u]short
n
cl_ [u]shortn 16-bit [un]signed
[u]int
n
cl_ [u]intn 32-bit [un]signed
[u]long
n
cl_ [u]longn 64-bit [un]signed
oat
n
cl_oatn 32-bit oat
double
n
cl_doublen 64-bit oat
half
n
cl_ halfn 16-bit oat
Other types
[3.7.1, 3.8.1]
Header <opencl_image>
Image and sampler types require CL_DEVICE_IMAGE_SUPPORT
is CL_TRUE. See header <opencl_pipe> for pipe type. See
header <opencl_device_queue> for device_queue type.
Type in OpenCL C++ API type for applicaon
cl::sampler cl_sampler
cl::image[1d, 2d, 3d]
cl::image1d_[buer, array]
cl::image2d_ms
cl::image2d_array[_ms]
cl::image2d_depth[_ms]
cl::image2d_array_depth[_ms]
cl_image
cl::pipe cl_pipe
cl::device_queue cl_queue
half wrapper [3.6.1]
Header <opencl_half> OpenCL C++ implements a wrapper
class for the built-in half data type. The class methods perform
implicit vload_half and vstore_half operaons from Vector Data
Load and Store Funcons secon.
fp16(const half &r) noexcept;
Constructs an object with a
half built-in type.
fp16(const oat &r) noexcept;
Constructs an object with a
oat built-in type.
fp16(const double &r) noexcept;
Constructs an object with a
double built-in type.
ndrange [3.13.6]
Header <opencl_device_queue> The ndrange type is used to
represent the size of the enqueued workload with a dimension
from 1 to 3.
struct ndrange {
explicit ndrange(size_t global_work_size) noexcept;
ndrange(size_t global_work_size, size_t local_work_size
noexcept;
ndrange(size_t global_work_oset, size_t global_work_size,
size_t local_work_size) noexcept;
template <size_t N>
ndrange(const size_t (&global_work_size)[N]) noexcept;
template <size_t N>
ndrange(const size_t (&global_work_size)[N],
const size_t (&global_work_size)[N]) noexcept;
template <size_t N>
ndrange(const size_t (&global_work_oset)[N],
const size_t (&global_work_size)[N],
const size_t (&global_work_size)[N]) noexcept;
};
Example
#include <opencl_device_queue>
#include <opencl_work_item>
using namespace cl;
kernel void foo(device_queue q) {
q.enqueue_kernel(cl::enqueue_policy::no_wait, cl::ndrange( 1 ),
[](){ uint d = get_global_id(0); } );
}