给出一个坐标集,求以该坐标集为坐标所构成的多边形的外界四边形的四个顶点
时间: 2023-04-12 22:01:21 浏览: 93
这个问题可以使用凸包算法来解决。凸包算法可以找到一个点集的最小凸多边形,而最小凸多边形的外接矩形就是我们要找的外界四边形的四个顶点。
以下是使用 Graham 扫描算法实现凸包的示例代码:
```python
def orientation(p, q, r):
"""计算向量 pq 和 pr 的叉积"""
return (q[1] - p[1]) * (r[0] - q[0]) - (q[0] - p[0]) * (r[1] - q[1])
def convex_hull(points):
"""计算点集的凸包"""
# 先按照 x 坐标排序,如果 x 坐标相同则按照 y 坐标排序
points = sorted(points, key=lambda p: (p[0], p[1]))
n = len(points)
# 初始化栈,先把前两个点加入栈中
stack = [points[0], points[1]]
# 构建凸包
for i in range(2, n):
while len(stack) >= 2 and orientation(stack[-2], stack[-1], points[i]) <= 0:
stack.pop()
stack.append(points[i])
# 把最后一个点加入栈中
stack.append(points[-1])
return stack
def bounding_box(points):
"""计算点集的外接矩形"""
# 先计算凸包
hull = convex_hull(points)
# 找到凸包的最左、最右、最上、最下的点
leftmost = min(hull, key=lambda p: p[0])
rightmost = max(hull, key=lambda p: p[0])
topmost = min(hull, key=lambda p: p[1])
bottommost = max(hull, key=lambda p: p[1])
return [leftmost, topmost, rightmost, bottommost]
# 示例用法
points = [(0, 0), (1, 1), (2, 2), (3, 1), (2, 0)]
box = bounding_box(points)
print(box) # 输出 [(0, 0), (0, 2), (3, 2), (3, 0)]
```
这个算法的时间复杂度是 O(n log n),其中 n 是点集的大小。
相关推荐
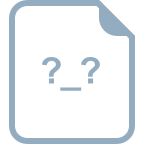














