unity Action
时间: 2023-06-21 14:09:32 浏览: 154
Unity中的Action是一种委托(Delegate),它可以用来传递方法,使得我们可以在代码中动态地指定方法。Action委托可以用来代替没有返回值(void)的方法。它定义在System命名空间中,其定义如下:
```csharp
public delegate void Action();
```
Action委托可以有0个到16个参数,但是不包括返回值。例如:
```csharp
public delegate void Action<T>(T obj);
public delegate void Action<T1, T2>(T1 arg1, T2 arg2);
// ...
```
使用Action委托可以方便地将方法作为参数传递给其他方法,或者在事件的处理程序中使用。例如,我们可以这样定义一个方法:
```csharp
public void DoSomething()
{
Debug.Log("Do something");
}
```
然后将它作为参数传递给另一个方法:
```csharp
public void DoSomethingElse(Action action)
{
action();
}
```
这样当我们调用`DoSomethingElse(DoSomething)`时,就会输出"Do something"。
相关问题
unity action
Unity Action is a programming construct in Unity game engine that allows developers to define a set of actions or behaviors that can be triggered by certain events or inputs. Actions can be anything from playing an animation, moving an object, or executing a script.
Unity Actions are commonly used to create gameplay mechanics, such as shooting a weapon or jumping, as well as for user interface interactions, such as pressing a button or selecting an option from a menu.
Developers can define their own custom actions or use pre-built ones provided by Unity, such as the built-in UnityEvent system. Unity Actions are typically set up in the Unity Editor, using the Inspector window or scripting.
Overall, Unity Actions provide a flexible and powerful way to create interactive experiences in Unity games and applications.
unity Action传参
Unity中的Action是一种委托类型,可以用于定义不带参数和返回值的方法。如果需要在Action中传递参数,可以使用lambda表达式或者匿名方法来实现。
例如,定义一个Action类型的方法:
```csharp
public void DoSomething(Action<int> callback)
{
// 在这里调用callback,将一个int类型的参数传递给它
callback(42);
}
```
然后,在调用这个方法时,可以传递一个lambda表达式或者匿名方法:
```csharp
DoSomething((int result) =>
{
Debug.Log("The result is: " + result);
});
```
其中,lambda表达式 `(int result) => { Debug.Log("The result is: " + result); }` 接收一个int类型的参数,并在控制台输出结果。在方法内部,调用 `callback(42)` 时,会将参数 42 传递给lambda表达式,从而执行输出结果的操作。
阅读全文
相关推荐
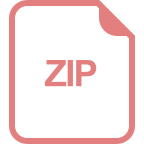












