my code:Z = np.random.standard_normal((M, N)) S = np.zeros((M, N+1)) S[:, 0] = S0 for i in range(N): S[:, i+1] = S[:, i] * np.exp((r-rf - 0.5*sigma**2)*dt + sigma*np.sqrt(dt)*Z[:, i]) error:if np.any((S[i, 21:126] > U) | (S[i, 201:231] < L) | (S[i, -1] < 1.3) | (S[i, -1] > 1.8)): ValueError: operands could not be broadcast together with shapes (105,) (30,)
时间: 2023-10-31 21:06:32 浏览: 70
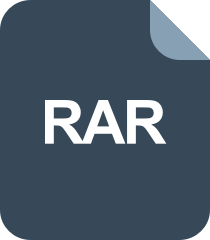
mseq.rar_matlab ms_mseq_mseq.m_m序列
这个错误是因为 `(S[i, 21:126] > U)` 和 `(S[i, 201:231] < L)` 的形状不兼容,导致无法进行逻辑运算。
根据你的代码,`S` 是一个 `(M, N+1)` 的数组,所以 `S[i, 21:126]` 的形状是 `(105,)`,而 `S[i, 201:231]` 的形状是 `(30,)`。所以在进行 `|` 运算时,两个形状不兼容,导致了这个错误。
你需要检查你的切片操作是否正确,并且确保你的数组形状是一致的。如果你需要使用不同的切片操作,可以考虑使用 `np.concatenate()` 等函数来将不同的数组拼接起来,以保证它们的形状一致。
如果你无法解决这个问题,可以将你的代码和具体错误信息提供给我,我会帮助你更好地解决这个问题。
阅读全文
相关推荐
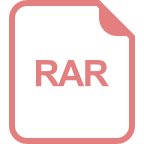
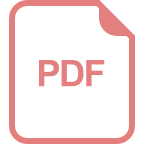
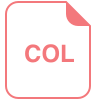
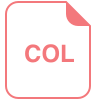
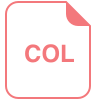
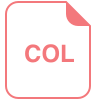
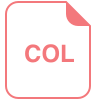
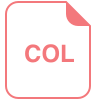
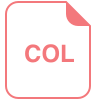
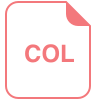



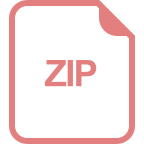
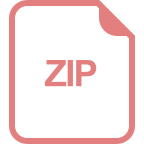
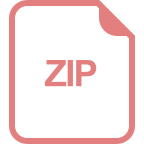
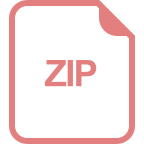
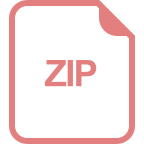
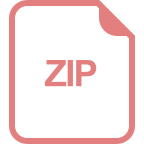