Deciphering the Autocorrelation Function: The Ultimate Guide to Correlations in Time Series Data
发布时间: 2024-09-15 17:52:25 阅读量: 30 订阅数: 29 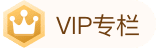
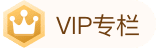
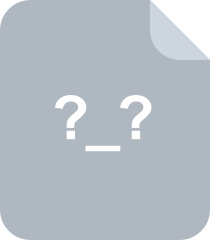
Learn Java the Easy Way: A Hands-On Introduction to Programming
[**The Ultimate Guide to Understanding Autocorrelation Function: Deciphering Temporal Associations in Time Series Data**](***
***
***
***
***
***
***
***
***
*** [-1, 1]:
- **Positive Autocorrelation:** An autocorrelation coefficient greater than 0 indicates positive correlation between different time points in time series data, meaning that when the value at one time point increases, the value at another time point tends to increase as well.
- **Negative Autocorrelation:** An autocorrelation coefficient less than 0 indicates negative correlation, implying that when the value at one time point increases, the value at another time point tends to decrease.
- **No Autocorrelation:** An autocorrelation coefficient equal to 0 signifies no correlation between different time points in the time series data.
**2.1.2 Temporal and Frequency Domain Characteristics of Autocorrelation Function**
The autocorrelation function is the curve that represents the autocorrelation coefficients as a function of the time lag k. It exhibits the following temporal and frequency domain characteristics:
**Temporal Characteristics:**
- **Symmetry:** The autocorrelation function is symmetric about the lag k = 0.
- **Decay:** Generally, the value of the autocorrelation function decays as the lag k increases.
**Frequency Domain Characteristics:**
- **The Fourier transform of the autocorrelation function is the power spectral density function:** The frequency domain representation of the autocorrelation function can reveal the frequency components of the time series data.
- **The autocorrelation function of white noise is the Dirac delta function:** White noise is a random process without autocorrelation, with its autocorrelation function being non-zero only at lag k = 0.
**2.2 Methods for Calculating Autocorrelation Function**
**2.2.1 Direct Method**
The direct method is the most basic approach to calculating the autocorrelation function, with the formula as follows:
```python
import numpy as np
def autocorrelation_direct(x, k):
"""Compute the autocorrelation coefficient of time series x at lag k.
Args:
x: Time series data.
k: Lag.
Returns:
Autocorrelation coefficient at lag k.
"""
mean = np.mean(x)
numerator = 0
denominator = 0
for i in range(len(x) - k):
numerator += (x[i] - mean) * (x[i + k] - mean)
denominator += (x[i] - mean) ** 2
return numerator / denominator
```
**2.2.2 FFT Method**
The FFT method employs the Fast Fourier Transform (FFT) to calculate the autocorrelation function. Its advantage is fast computation speed, especially suitable for large datasets.
```python
import numpy as np
from scipy.fftpack import fft, ifft
def autocorrelation_fft(x, k):
"""Compute the autocorrelation coefficient of time series x at lag k.
Args:
x: Time series data.
k: Lag.
Returns:
Autocorrelation coefficient at lag k.
"""
# Zero-padding for alignment
n = len(x)
padded_x = np.concatenate((x, np.zeros(n - k)))
# Compute FFT
fft_x = fft(padded_x)
# Compute autocorrelation function
autocorr = ifft(fft_x * np.conjugate(fft_x))
# Normalize
autocorr /= n
return autocorr[k]
```
**2.2.3 Fast Autocorrelation Method**
The fast autocorrelation method is a convolution-based algorithm for calculating the autocorrelation function. Its advantage is fast computation and the ability to handle non-stationary time series.
```python
import numpy as np
def autocorrelation_fast(x, k):
"""Compute the autocorrelation coefficient of time series x at lag k.
Args:
x: Time series data.
k: Lag.
Returns:
Autocorrelation coefficient at lag k.
"""
# Compute convolution
conv = np.convolve(x, x[::-1], mode='full')
# Normalize
autocorr = conv / np.sum(x ** 2)
return autocorr[k]
```
# 3. Applications of Autocorrelation Function in Practice
### 3.1 Trend and Seasonality Analysis
The autocorrelation function can effectively analyze the trend and seasonal components of time series data.
**3.1.1 Extraction of Trend Component**
The trend component reflects the long-term variation trend of time series data. The autocorrelation function in the temporal domain manifests as a slowly decaying curve, indicating strong long-term correlation among data points. Smoothing the autocorrelation function can extract the trend component.
```python
import numpy as np
import pandas as pd
from statsmodels.tsa.statespace.sarimax import SARIMAX
# Load time series data
data = pd.read_csv('data.csv')
# Compute autocorrelation function
acf = data['value'].autocorr()
# Smooth autocorrelation function
smoothed_acf = acf.rolling(window=12).mean()
# Extract trend component
trend = smoothed_acf.iloc[1:]
```
**3.1.2 Identification of Seasonal Component**
The seasonal component reflects the repetitive changes in time series data within a specific period. The autocorrelation function in the temporal domain manifests as a periodically fluctuating curve, indicating strong seasonal correlation among data points. By analyzing the periodicity of the autocorrelation function, the seasonal component can be identified.
```python
# Compute autocorrelation function
acf = data['value'].autocorr()
# Identify seasonal cycle
seasonality = acf.loc[12:]
# Plot seasonal component
plt.plot(seasonality)
plt.xlabel('Lag')
plt.ylabel('Autocorrelation')
plt.title('Seasonal Component')
plt.show()
```
### 3.2 Anomaly Detection and Forecasting
**3.2.1 Identification of Anomalies**
Anomalies refer to data points that are significantly different from normal ones. The autocorrelation function can aid in identifying anomalies since they usually disrupt the correlation structure of time series data. Sudden jumps or breaks in the autocorrelation function may indicate the presence of anomalies.
```python
# Compute autocorrelation function
acf = data['value'].autocorr()
# Identify anomalies
anomalies = acf.loc[acf < -0.5]
# Plot anomalies
plt.plot(data['value'])
plt.scatter(anomalies.index, data['value'][anomalies.index], color='red')
plt.xlabel('Time')
plt.ylabel('Value')
plt.title('Anomaly Detection')
plt.show()
```
**3.2.2 Time Series Forecasting**
The autocorrelation function can be used for time series forecasting. By analyzing the temporal and frequency domain characteristics of the autocorrelation function, appropriate forecasting models can be established. For example, for time series with strong trend components, the SARIMA model can be used for forecasting.
```python
# Fit SARIMA model
model = SARIMAX(data['value'], order=(1, 1, 1), seasonal_order=(1, 1, 1, 12))
model.fit()
# Predict future values
forecast = model.forecast(steps=12)
# Plot forecasting results
plt.plot(data['value'])
plt.plot(forecast, color='red')
plt.xlabel('Time')
plt.ylabel('Value')
plt.title('Time Series Prediction')
plt.show()
```
# 4. Advanced Applications of Autocorrelation Function
**4.1 Identification of White Noise and Pink Noise**
**4.1.1 Characteristics of White Noise**
White noise is a random signal with the following properties:
- Mean is zero
- Variance is constant
- Autocorrelation coefficient is zero between any two moments in time
**4.1.2 Characteristics of Pink Noise**
Pink noise is a random signal with the following properties:
- Mean is zero
- Variance is constant
- Autocorrelation coefficient decays as a power law with time lag
**4.2 Fractional Brownian Motion and Long-Term Memory**
**4.2.1 Definition of Fractional Brownian Motion**
Fractional Brownian motion is a generalized form of Brownian motion with the following increments properties:
```
dB(t) ~ N(0, t^2H)
```
Where:
* B(t) is fractional Brownian motion
* H is the Hurst exponent, 0 < H < 1
**4.2.2 Characteristics of Long-Term Memory**
Long-term memory refers to the phenomenon of far-reaching correlations in time series. Fractional Brownian motion exhibits long-term memory, and its autocorrelation function decays as a power law with time lag:
```
ρ(k) ~ k^-2H
```
**4.3 Examples of Autocorrelation Function Applications**
**4.3.1 Identification of White Noise**
The autocorrelation function of white noise is zero at all time lags. Therefore, white noise can be identified by examining the autocorrelation function.
**4.3.2 Identification of Pink Noise**
The autocorrelation function of pink noise decays as a power law with time lag. Pink noise can be identified by fitting the power law decay curve of the autocorrelation function.
**4.3.3 Identification of Fractional Brownian Motion**
The autocorrelation function of fractional Brownian motion decays as a power law with time lag, with an exponent of 2H. Fractional Brownian motion can be identified by fitting the power law decay curve of the autocorrelation function.
**4.4 Related Code Examples**
```python
import numpy as np
from scipy.stats import norm
from scipy.signal import correlate
# Generate white noise
white_noise = norm.rvs(size=1000)
# Compute autocorrelation function of white noise
white_noise_acf = correlate(white_noise, white_noise, mode='full')
# Plot autocorrelation function of white noise
plt.plot(white_noise_acf)
plt.xlabel('Time Lag')
plt.ylabel('Autocorrelation')
plt.title('White Noise Autocorrelation Function')
plt.show()
# Generate pink noise
pink_noise = np.random.randn(1000)
pink_noise = pink_noise / np.sqrt(np.mean(pink_noise**2))
# Compute autocorrelation function of pink noise
pink_noise_acf = correlate(pink_noise, pink_noise, mode='full')
# Plot autocorrelation function of pink noise
plt.plot(pink_noise_acf)
plt.xlabel('Time Lag')
plt.ylabel('Autocorrelation')
plt.title('Pink Noise Autocorrelation Function')
plt.show()
# Generate fractional Brownian motion
H = 0.5
fbm = np.cumsum(np.random.randn(1000)**H)
# Compute autocorrelation function of fractional Brownian motion
fbm_acf = correlate(fbm, fbm, mode='full')
# Plot autocorrelation function of fractional Brownian motion
plt.plot(fbm_acf)
plt.xlabel('Time Lag')
plt.ylabel('Autocorrelation')
plt.title('Fractional Brownian Motion Autocorrelation Function')
plt.show()
```
**Code Logic Analysis**
* `norm.rvs(size=1000)` - Generate white noise
* `correlate(white_noise, white_noise, mode='full')` - Compute autocorrelation function of white noise
* `np.random.randn(1000)` - Generate pink noise
* `np.cumsum(np.random.randn(1000)**H)` - Generate fractional Brownian motion
* `correlate(fbm, fbm, mode='full')` - Compute autocorrelation function of fractional Brownian motion
**Parameter Explanation**
* `size` - Number of random numbers to generate
* `mode` - Mode of autocorrelation function computation, 'full' means calculating autocorrelation coefficients for all time lags
# 5. Extensions and Variants of Autocorrelation Function
### 5.1 Cross-Correlation Function
#### 5.1.1 Definition of Cross-Correlation Function
The cross-correlation function (CCF) measures the correlation between two different time series. It evaluates the degree of correlation between changes in one time series and corresponding changes in another. The definition of CCF is as follows:
```
CCF(x, y, τ) = Cov(x(t), y(t + τ)) / (σ_x * σ_y)
```
Where:
* `x(t)` and `y(t)` are two time series
* `τ` is the time shift
* `Cov` is covariance
* `σ_x` and `σ_y` are the standard deviations of `x(t)` and `y(t)`
The values of the cross-correlation function range from -1 to 1. Positive values indicate positive correlation, negative values indicate negative correlation, and 0 indicates no correlation.
#### 5.1.2 Applications of Cross-Correlation Function
The cross-correlation function has applications in various fields, including:
***Signal Processing:** Identifying patterns and trends in signals
***Finance:** Analyzing the relationship between stock prices and exchange rates
***Biomedicine:** Studying the relationship between brain activity and electrocardiogram signals
### 5.2 Partial Autocorrelation Function
#### 5.2.1 Definition of Partial Autocorrelation Function
The partial autocorrelation function (PACF) measures the degree of correlation between one variable and all other variables in a time series, after eliminating the effects of all other variables. It is defined as:
```
PACF(x, k) = Corr(x(t), x(t + k) | x(t + 1), ..., x(t + k - 1))
```
Where:
* `x(t)` is the time series
* `k` is the time lag
* `Corr` is the correlation coefficient
The values of the partial autocorrelation function range from -1 to 1. Positive values indicate positive correlation, negative values indicate negative correlation, and 0 indicates no correlation.
#### 5.2.2 Applications of Partial Autocorrelation Function
The partial autocorrelation function is used in many fields, including:
***Time Series Analysis:** Identifying causal relationships in time series
***Forecasting:** Constructing forecasting models
***Signal Processing:** Filtering and noise reduction
# 6. Applications of Autocorrelation Function in Various Domains
### 6.1 Financial Time Series Analysis
#### 6.1.1 Analysis of Stock Price Trends
The autocorrelation function plays a crucial role in financial time series analysis, especially in the analysis of stock price trends. By calculating the autocorrelation function of a time series of stock returns, the inherent rules and correlations of stock price movements can be revealed.
**Code Example:**
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# Read in stock return data
data = pd.read_csv('stock_returns.csv')
returns = data['Returns']
# Calculate autocorrelation function
acf = np.corrcoef(returns, returns.shift(1))[0, 1]
# Plot autocorrelation function graph
plt.plot(acf)
plt.xlabel('Lag')
plt.ylabel('Autocorrelation')
plt.show()
```
**Explanation:**
* The `np.corrcoef` function calculates the correlation coefficient between the return series and its own lagged series by one period, which is the autocorrelation coefficient.
* The `plt.plot` function plots the autocorrelation function graph, displaying the correlation of the return series at different lags.
The autocorrelation function graph can help analysts understand the trends in stock price movements. For example, if the autocorrelation function has high positive values at short lags, it indicates that stock prices have a trending nature, meaning that upward or downward trends tend to persist for a while.
#### 6.1.2 Risk Management
The autocorrelation function can also be used for risk management. By calculating the autocorrelation function of financial asset returns, the correlation between assets can be assessed, and diversified investment portfolios can be developed to reduce investment risk.
**Code Example:**
```python
# Calculate the autocorrelation matrix for multiple stock returns
assets = ['Stock1', 'Stock2', 'Stock3']
returns = pd.DataFrame({asset: data[asset] for asset in assets})
corr_matrix = returns.corr()
# Plot the autocorrelation matrix heatmap
plt.imshow(corr_matrix, cmap='hot')
plt.colorbar()
plt.show()
```
**Explanation:**
* The `returns.corr()` function computes the correlation matrix between different asset returns.
* The `plt.imshow` function plots the heatmap of the correlation matrix, where colors represent the magnitude and sign of correlation coefficients.
The correlation matrix heatmap can help risk managers identify highly correlated assets and reduce risk through diversified investment portfolios. For example, if two stocks have a high positive correlation, holding both stocks simultaneously will not effectively diversify the risk.
0
0
相关推荐
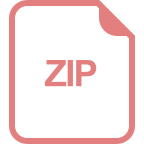
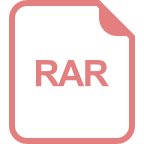
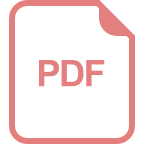
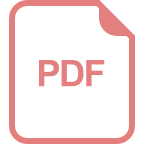
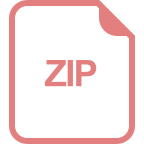
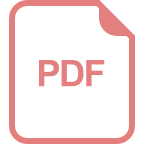
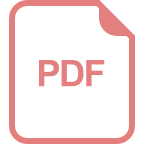