Matlab Autocorrelation Function Optimization Secrets: Enhancing Efficiency and Accuracy
发布时间: 2024-09-15 17:58:09 阅读量: 51 订阅数: 32 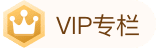
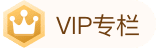
# The Secrets of Optimizing Matlab's Autocorrelation Function: Enhancing Efficiency and Accuracy
## 1. The Basics of Matlab's Autocorrelation Function
The autocorrelation function is a mathematical tool for measuring the similarity of a signal or data sequence with itself. In Matlab, the autocorrelation function can be calculated using the `xcorr` function.
The basic syntax of the `xcorr` function is:
```matlab
[c, lags] = xcorr(x, y, maxlags)
```
Where:
* `x` and `y` are the input signals or data sequences.
* `maxlags` specifies the maximum number of lags for which to compute the autocorrelation coefficients.
## 2. Optimization Tips for Matlab's Autocorrelation Function
The autocorrelation function is widely used in practical applications, but its computation can be quite extensive, especially for long sequences of data. To improve the computational efficiency of the autocorrelation function, optimization techniques are necessary. This chapter introduces two commonly used optimization algorithms: the Fourier Transform method and the Circular Convolution method, as well as data preprocessing techniques.
### 2.1 Selection of Optimization Algorithms
#### 2.1.1 The Fourier Transform Method
The Fourier Transform method is an autocorrelation function calculation method based on the Fourier Transform. Its fundamental principle is to convert the time-domain signal into a frequency-domain signal, use the simple and fast properties of the autocorrelation function of the frequency-domain signal, and then convert the result back to the time domain.
```
X_fft = fft(x);
autocorr_fft = ifft(X_fft .* conj(X_fft));
```
**Code Logic Analysis:**
1. `fft(x)`: Converts the time-domain signal `x` into the frequency-domain signal `X_fft`.
2. `conj(X_fft)`: Finds the complex conjugate of the frequency-domain signal `X_fft`.
3. `X_fft .* conj(X_fft)`: Multiplies the frequency-domain signal `X_fft` and its complex conjugate element by element, resulting in the frequency-domain representation of the autocorrelation function.
4. `ifft()`: Converts the frequency-domain representation of the autocorrelation function back into the time-domain representation, obtaining the autocorrelation function `autocorr_fft`.
**Parameter Description:**
- `x`: Time-domain signal.
#### 2.1.2 The Circular Convolution Method
The Circular Convolution method is an autocorrelation function calculation method based on circular convolution. Its basic principle is to perform circular convolution of the time-domain signal with itself to obtain the autocorrelation function.
```
autocorr_conv = conv(x, x, 'same');
```
**Code Logic Analysis:**
1. `conv(x, x, 'same')`: Performs circular convolution of the time-domain signal `x` with itself, resulting in the autocorrelation function `autocorr_conv`.
2. The `'same'` parameter specifies the use of a convolution kernel of the same length, i.e., the same as the length of the input signal.
**Parameter Description:**
- `x`: Time-domain signal.
### 2.2 Data Preprocessing
Data preprocessing can effectively improve the computational efficiency and accuracy of the autocorrelation function.
#### 2.2.1 Mean Removal
The mean removal operation can eliminate the DC component of the signal, improving the computational accuracy of the autocorrelation function.
```
x_demeaned = x - mean(x);
```
**Code Logic Analysis:**
1. `mean(x)`: Calculates the mean of the time-domain signal `x`.
2. `x - mean(x)`: Subtracts the mean from the time-domain signal `x`, resulting in the mean-removed signal `x_demeaned`.
**Parameter Description:**
- `x`: Time-domain signal.
#### 2.2.2 Normalization
Normalization can limit the amplitude of the signal to a certain range, improving the computational stability of the autocorrelation function.
```
x_normalized = x / max(abs(x));
```
**Code Logic Analysis:**
1. `max(abs(x))`: Calculates the maximum absolute value of the time-domain signal `x`.
2. `x / max(abs(x))`: Normalizes the time-domain signal `x` to the range [0, 1], resulting in the normalized signal `x_normalized`.
**Parameter Description:**
- `x`: Time-domain signal.
## 3. Practical Applications of Matlab's Autocorrelation Function
### 3.1 Signal Processing
The autocorrelation function has extensive applications in the field of signal processing, mainly used to analyze the periodicity, similarity, and noise characteristics of signals.
#### 3.1.1 Noise Reduction
The autocorrelation function can effectively eliminate noise
0
0