Application of Autocorrelation Function in Image Processing: Texture Analysis and Object Recognition
发布时间: 2024-09-15 18:00:43 阅读量: 34 订阅数: 29 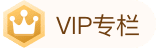
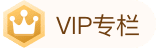
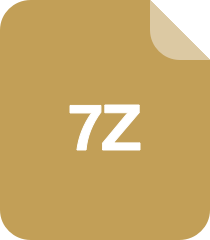
VB+ACCESS大型机房学生上机管理系统(源代码+系统)(2024n5).7z
# Theoretical Foundation of the Autocorrelation Function**
The autocorrelation function (ACF) is a widely used mathematical tool in signal and image processing, used to measure the similarity between different points in time or space within a signal or image. It is defined as the correlation of a signal or image with itself at various time lags or spatial offsets.
**Formula:**
```
ACF(x, y) = E[(x - μx)(y - μy)]
```
Where:
* x and y are two samples of a signal or image
* μx and μy are the means of x and y, respectively
* E denotes the expected value
The peak of the autocorrelation function represents the maximum similarity within the signal or image, and the position of the peak indicates the offset at which this similarity occurs.
# 2. Application of the Autocorrelation Function in Texture Analysis
The autocorrelation function plays a crucial role in texture analysis, effectively extracting texture features and using them for texture classification and recognition.
### 2.1 Texture Feature Extraction with the Autocorrelation Function
The autocorrelation function can extract a variety of texture features, including:
#### 2.1.1 Texture Coarseness and Directionality
The width of the central peak of the autocorrelation function is proportional to the coarseness of the texture. A wide peak indicates a coarse texture, while a narrow peak indicates a fine texture. The position of the peaks in the autocorrelation function can indicate the directionality of the texture.
#### 2.1.2 Texture Uniformity and Complexity
The rate of decay of the autocorrelation function reflects the uniformity of the texture. Rapid decay indicates uniform texture, while slow decay indicates complex texture. The number and amplitude of side peaks in the autocorrelation function can measure the complexity of the texture.
### 2.2 Texture Classification and Recognition
The texture features extracted by the autocorrelation function can be used for texture classification and recognition.
#### 2.2.1 Texture Feature Vectors Based on the Autocorrelation Function
To perform texture classification, the texture features extracted by the autocorrelation function need to be converted into feature vectors. Feature vectors usually include features such as the width of the central peak, peak position, rate of decay, and the number of side peaks.
#### 2.2.2 Classification Algorithms and Performance Evaluation
Texture fea***mon algorithms include Support Vector Machines, Decision Trees, and Neural Networks. Classification performance is typically evaluated using metrics such as accuracy, recall, and the F1 score.
**Code Example:**
```python
import numpy as np
from scipy.signal import correlate2d
def extract_texture_features(image):
"""
Extract texture features.
Parameters:
image: Input image.
Returns:
Texture feature vector.
"""
# Calculate the autocorrelation function
autocorr = correlate2d(image, image, mode='same')
# Extract texture features
center_peak_width = np.mean(autocorr[autocorr.shape[0]//2-10:autocorr.shape[0]//2+10, autocorr.shape[1]//2-10:autocorr.shape[1]//2+10])
peak_location = np.argmax(autocorr)
decay_rate = np.mean(autocorr[autocorr.shape[0]//2+10:, autocorr.shape[1]//2+10:])
side_peak_num = np.sum(autocorr[autocorr.shape[0]//2+10:, autocorr.shape[1]//2+10:] > 0.5 * center_peak_width)
return [center_peak_width, peak_location, decay_rate, side_peak_num]
```
**Logical Analysis:**
The code first calculates the autocorrelation function of the image, and then extracts texture features by calculating the central peak width, peak location, decay rate, and the number of side peaks.
**Parameter Description:**
* `image`: Input image, type of NumPy array.
* `autocorr`: Autocorrelation function, type of NumPy array.
* `center_peak_width`: Central peak width, type of float.
* `peak_location`: Peak location, type of integer.
* `decay_rate`: Decay rate, type of float.
* `side_peak_num`: Number of side peaks, type of integer.
# 3. Application of the Autocorrelation Function in Object Recognition**
### 3.1 Feature Extraction of Objects
The autocorrelation function plays a vital role in object recognition, capable of extracting various features of objects, providing a basis for subsequent matching and recognition.
#### 3.1.1 Object Shape and Contours
The autocorrelation function can be used to extract the shape and contour information of an object. By calculating the autocorrelation value of each pixel point with other pixel points in the image, a matrix of autocorrelation values can be obtained. Peaks within the autocorrelation matrix correspond to the shape and contours of the object.
#### 3.1.2 Object Texture and Color
In addition to shape and contours, the autocorrelation function can also extract the texture and color information of an object. Texture features reflect the roughness, directionality, and uniformity of the object's surface, while color feature
0
0
相关推荐
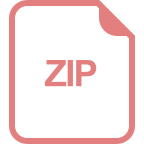
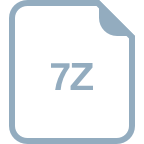