一份坦克大战的java代码
时间: 2023-10-05 18:05:12 浏览: 89
以下是一个简单的坦克大战游戏的 Java 代码,希望能对你有所帮助:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.Rectangle;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.awt.image.BufferedImage;
import java.util.ArrayList;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class TankWar extends JPanel implements KeyListener, Runnable {
private static final long serialVersionUID = 1L;
private JFrame frame;
private Tank playerTank;
private ArrayList<EnemyTank> enemyTanks;
private ArrayList<Explosion> explosions;
private BufferedImage offScreenImage;
private Graphics offScreenGraphics;
private boolean[] keys;
public TankWar() {
frame = new JFrame("Tank War");
frame.setSize(800, 600);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
playerTank = new Tank(400, 500, Tank.Direction.UP, true);
enemyTanks = new ArrayList<EnemyTank>();
explosions = new ArrayList<Explosion>();
for (int i = 0; i < 5; i++) {
enemyTanks.add(new EnemyTank(50 + i * 150, 50, Tank.Direction.DOWN));
}
offScreenImage = new BufferedImage(800, 600, BufferedImage.TYPE_INT_ARGB);
offScreenGraphics = offScreenImage.getGraphics();
keys = new boolean[256];
frame.addKeyListener(this);
frame.add(this);
frame.setVisible(true);
new Thread(this).start();
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
offScreenGraphics.setColor(Color.WHITE);
offScreenGraphics.fillRect(0, 0, 800, 600);
playerTank.draw(offScreenGraphics);
for (EnemyTank enemyTank : enemyTanks) {
enemyTank.draw(offScreenGraphics);
}
for (Explosion explosion : explosions) {
explosion.draw(offScreenGraphics);
}
g.drawImage(offScreenImage, 0, 0, null);
}
@Override
public void keyPressed(KeyEvent e) {
keys[e.getKeyCode()] = true;
if (keys[KeyEvent.VK_SPACE]) {
playerTank.fire();
}
}
@Override
public void keyReleased(KeyEvent e) {
keys[e.getKeyCode()] = false;
}
@Override
public void keyTyped(KeyEvent e) {
}
@Override
public void run() {
while (true) {
playerTank.move(keys);
for (EnemyTank enemyTank : enemyTanks) {
enemyTank.move();
}
for (int i = 0; i < playerTank.getMissiles().size(); i++) {
Missile missile = playerTank.getMissiles().get(i);
missile.move();
if (missile.isOutOfBound()) {
playerTank.getMissiles().remove(missile);
i--;
} else {
for (EnemyTank enemyTank : enemyTanks) {
if (missile.hit(enemyTank)) {
playerTank.getMissiles().remove(missile);
i--;
explosions.add(new Explosion(enemyTank.getX(), enemyTank.getY()));
enemyTanks.remove(enemyTank);
break;
}
}
}
}
for (int i = 0; i < explosions.size(); i++) {
Explosion explosion = explosions.get(i);
if (explosion.isOver()) {
explosions.remove(explosion);
i--;
}
}
for (EnemyTank enemyTank : enemyTanks) {
for (int i = 0; i < enemyTank.getMissiles().size(); i++) {
Missile missile = enemyTank.getMissiles().get(i);
missile.move();
if (missile.isOutOfBound()) {
enemyTank.getMissiles().remove(missile);
i--;
} else {
if (missile.hit(playerTank)) {
enemyTank.getMissiles().remove(missile);
i--;
explosions.add(new Explosion(playerTank.getX(), playerTank.getY()));
playerTank.setAlive(false);
break;
}
}
}
if (playerTank.isAlive() && enemyTank.getRectangle().intersects(playerTank.getRectangle())) {
explosions.add(new Explosion(playerTank.getX(), playerTank.getY()));
playerTank.setAlive(false);
break;
}
}
if (enemyTanks.isEmpty()) {
System.out.println("You win!");
System.exit(0);
}
if (!playerTank.isAlive()) {
System.out.println("Game over!");
System.exit(0);
}
repaint();
try {
Thread.sleep(50);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
new TankWar();
}
private class Tank {
private int x;
private int y;
private Direction direction;
private boolean alive;
private Image[] images;
private int currentImageIndex;
private ArrayList<Missile> missiles;
public Tank(int x, int y, Direction direction, boolean alive) {
this.x = x;
this.y = y;
this.direction = direction;
this.alive = alive;
images = new Image[8];
for (int i = 0; i < 8; i++) {
images[i] = TankWarUtils.getImage("tank" + (i + 1) + ".png");
}
currentImageIndex = 0;
missiles = new ArrayList<Missile>();
}
public void draw(Graphics g) {
if (alive) {
g.drawImage(images[currentImageIndex], x, y, null);
} else {
g.drawImage(TankWarUtils.getImage("explosion.png"), x, y, null);
}
for (Missile missile : missiles) {
missile.draw(g);
}
}
public void move(boolean[] keys) {
int moveLength = 5;
if (keys[KeyEvent.VK_UP]) {
direction = Direction.UP;
y -= moveLength;
}
if (keys[KeyEvent.VK_DOWN]) {
direction = Direction.DOWN;
y += moveLength;
}
if (keys[KeyEvent.VK_LEFT]) {
direction = Direction.LEFT;
x -= moveLength;
}
if (keys[KeyEvent.VK_RIGHT]) {
direction = Direction.RIGHT;
x += moveLength;
}
if (x < 0) {
x = 0;
}
if (x > 750) {
x = 750;
}
if (y < 0) {
y = 0;
}
if (y > 550) {
y = 550;
}
currentImageIndex++;
if (currentImageIndex >= 8) {
currentImageIndex = 0;
}
}
public void fire() {
missiles.add(new Missile(x + 20, y + 20, direction));
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public Rectangle getRectangle() {
return new Rectangle(x, y, 60, 60);
}
public ArrayList<Missile> getMissiles() {
return missiles;
}
public boolean isAlive() {
return alive;
}
public void setAlive(boolean alive) {
this.alive = alive;
}
public enum Direction {
UP, DOWN, LEFT, RIGHT
}
}
private class EnemyTank {
private int x;
private int y;
private Direction direction;
private Image[] images;
private int currentImageIndex;
private ArrayList<Missile> missiles;
public EnemyTank(int x, int y, Direction direction) {
this.x = x;
this.y = y;
this.direction = direction;
images = new Image[8];
for (int i = 0; i < 8; i++) {
images[i] = TankWarUtils.getImage("enemy" + (i + 1) + ".png");
}
currentImageIndex = 0;
missiles = new ArrayList<Missile>();
}
public void draw(Graphics g) {
g.drawImage(images[currentImageIndex], x, y, null);
for (Missile missile : missiles) {
missile.draw(g);
}
}
public void move() {
int moveLength = 3;
if (Math.random() < 0.01) {
fire();
}
if (Math.random() < 0.05) {
int r = (int) (Math.random() * 4);
if (r == 0) {
direction = Direction.UP;
} else if (r == 1) {
direction = Direction.DOWN;
} else if (r == 2) {
direction = Direction.LEFT;
} else if (r == 3) {
direction = Direction.RIGHT;
}
}
if (direction == Direction.UP) {
y -= moveLength;
} else if (direction == Direction.DOWN) {
y += moveLength;
} else if (direction == Direction.LEFT) {
x -= moveLength;
} else if (direction == Direction.RIGHT) {
x += moveLength;
}
if (x < 0) {
x = 0;
}
if (x > 750) {
x = 750;
}
if (y < 0) {
y = 0;
}
if (y > 550) {
y = 550;
}
currentImageIndex++;
if (currentImageIndex >= 8) {
currentImageIndex = 0;
}
}
public void fire() {
missiles.add(new Missile(x + 20, y + 20, direction));
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public Rectangle getRectangle() {
return new Rectangle(x, y, 60, 60);
}
public ArrayList<Missile> getMissiles() {
return missiles;
}
public enum Direction {
UP, DOWN, LEFT, RIGHT
}
}
private class Missile {
private int x;
private int y;
private Direction direction;
private Image image;
public Missile(int x, int y, Direction direction) {
this.x = x;
this.y = y;
this.direction = direction;
image = TankWarUtils.getImage("missile.png");
}
public void draw(Graphics g) {
g.drawImage(image, x, y, null);
}
public void move() {
int moveLength = 8;
if (direction == Direction.UP) {
y -= moveLength;
} else if (direction == Direction.DOWN) {
y += moveLength;
} else if (direction == Direction.LEFT) {
x -= moveLength;
} else if (direction == Direction.RIGHT) {
x += moveLength;
}
}
public boolean isOutOfBound() {
return x < 0 || x > 800 || y < 0 || y > 600;
}
public boolean hit(Tank tank) {
return getRectangle().intersects(tank.getRectangle());
}
public boolean hit(EnemyTank enemyTank) {
return getRectangle().intersects(enemyTank.getRectangle());
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public Rectangle getRectangle() {
return new Rectangle(x, y, 10, 10);
}
public enum Direction {
UP, DOWN, LEFT, RIGHT
}
}
private class Explosion {
private int x;
private int y;
private Image[] images;
private int currentImageIndex;
public Explosion(int x, int y) {
this.x = x;
this.y = y;
images = new Image[11];
for (int i = 0; i < 11; i++) {
images[i] = TankWarUtils.getImage("explosion" + (i + 1) + ".png");
}
currentImageIndex = 0;
}
public void draw(Graphics g) {
g.drawImage(images[currentImageIndex], x, y, null);
currentImageIndex++;
if (currentImageIndex >= 11) {
currentImageIndex = 0;
}
}
public boolean isOver() {
return currentImageIndex == 0;
}
}
private static class TankWarUtils {
public static Image getImage(String fileName) {
return new ImageIcon(TankWarUtils.class.getResource("/images/" + fileName)).getImage();
}
}
}
```
相关推荐
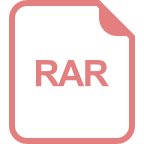
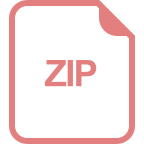
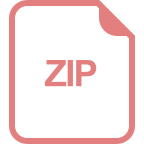
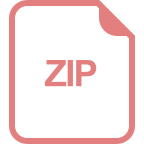











