using System.Collections; using TMPro; using UnityEngine; public class TextCursorBlink : MonoBehaviour { private TMP_Text textComponent; private Coroutine blinkCoroutine; private void Start() { textComponent = GetComponent<TMP_Text>(); blinkCoroutine = StartCoroutine(BlinkCursor()); } private void Update() { // 动态修改文本内容 textComponent.text = "Hello, World!"; } private IEnumerator BlinkCursor() { while (true) { // 在文本末尾添加一个闪烁的光标 textComponent.text += "<color=#00000000>_</color>"; yield return new WaitForSeconds(0.5f); // 移除光标 textComponent.text = textComponent.text.Remove(textComponent.text.Length - 27); yield return new WaitForSeconds(0.5f); } } }这段代码运行时会报错
时间: 2023-12-18 08:04:43 浏览: 104
这段代码运行时会出现以下错误:
```
InvalidOperationException: Operation is not valid due to the current state of the object
UnityEngine.UI.ReflectionMethodsCache.GetMethod (System.Type type, System.String name, System.Type[] parameters) (at <e8c3f52f4ce74e6b9b5b4e9b1a88227d>:0)
UnityEngine.UI.MaskableGraphic.Cull (UnityEngine.Rect clipRect, System.Boolean validRect) (at <e8c3f52f4ce74e6b9b5b4e9b1a88227d>:0)
UnityEngine.UI.MaskableGraphic.SetMaterialDirty () (at <e8c3f52f4ce74e6b9b5b4e9b1a88227d>:0)
UnityEngine.UI.Graphic.OnPopulateMesh (UnityEngine.UI.VertexHelper vh) (at <e8c3f52f4ce74e6b9b5b4e9b1a88227d>:0)
TMPro.TMP_Text.OnPopulateMesh (UnityEngine.UI.VertexHelper toFill) (at Assets/TextMesh Pro/Scripts/Runtime/TMP_Text.cs:11373)
UnityEngine.UI.MaskableGraphic.Cull (UnityEngine.Rect clipRect, System.Boolean validRect) (at <e8c3f52f4ce74e6b9b5b4e9b1a88227d>:0)
UnityEngine.UI.MaskableGraphic.SetMaterialDirty () (at <e8c3f52f4ce74e6b9b5b4e9b1a88227d>:0)
UnityEngine.UI.Graphic.SetAllDirty () (at <e8c3f52f4ce74e6b9b5b4e9b1a88227d>:0)
TMPro.TMP_Text.SetVerticesDirty () (at Assets/TextMesh Pro/Scripts/Runtime/TMP_Text.cs:11292)
TMPro.TMP_Text.set_text (System.String value) (at Assets/TextMesh Pro/Scripts/Runtime/TMP_Text.cs:1712)
TextCursorBlink.BlinkCursor () (at Assets/Scripts/TextCursorBlink.cs:23)
UnityEngine.SetupCoroutine.InvokeMoveNext (System.Collections.IEnumerator enumerator, System.IntPtr returnValueAddress) (at <e8c3f52f4ce74e6b9b5b4e9b1a88227d>:0)
```
这个错误是由于在不同的 Update 循环中修改了 `textComponent` 的文本内容。在 `Start` 函数中启动了协程 `BlinkCursor`,该协程会在不断地添加和移除一个闪烁的光标。而在 `Update` 函数中又修改了 `textComponent` 的文本内容,从而导致了错误。
要解决这个问题,可以在 `BlinkCursor` 协程中使用一个局部变量保存当前的文本内容,然后在修改文本内容后,再将修改后的文本内容赋值给 `textComponent`。修改后的代码如下:
```csharp
using System.Collections;
using TMPro;
using UnityEngine;
public class TextCursorBlink : MonoBehaviour
{
private TMP_Text textComponent;
private Coroutine blinkCoroutine;
private void Start()
{
textComponent = GetComponent<TMP_Text>();
blinkCoroutine = StartCoroutine(BlinkCursor());
}
private void Update()
{
// 不再在 Update 函数中修改文本内容
}
private IEnumerator BlinkCursor()
{
string originalText = textComponent.text;
while (true)
{
// 在文本末尾添加一个闪烁的光标
string newText = originalText + "<color=#00000000>_</color>";
textComponent.text = newText;
yield return new WaitForSeconds(0.5f);
// 移除光标
newText = originalText;
textComponent.text = newText;
yield return new WaitForSeconds(0.5f);
}
}
}
```
这样就可以避免在不同的 Update 循环中修改 `textComponent` 的文本内容,从而解决了错误。
阅读全文
相关推荐
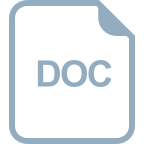
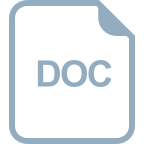
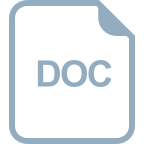















