IEnumerator DownloadImage() { UnityWebRequest request = UnityWebRequestTexture.GetTexture("https://www.example.com/image.png"); yield return request.SendWebRequest(); if (request.result == UnityWebRequest.Result.Success) { Texture2D texture = ((DownloadHandlerTexture)request.downloadHandler).texture; image.texture = texture; Resources.UnloadUnusedAssets(); // 清除内存 } } 如果多次调用,会存在资源堆积吗
时间: 2024-01-24 16:19:59 浏览: 197
是的,如果多次调用这个方法,会导致资源堆积。原因是每次调用该方法时,都会创建一个新的 UnityWebRequest 对象,并且下载的纹理也会被缓存起来。如果不手动释放这些对象和纹理,它们就会一直占用内存,最终导致内存溢出。
为了避免资源堆积,可以考虑在每次调用该方法前,先检查是否已经存在一个正在运行的下载任务,如果有,则等待其完成再开始新的下载。此外,在下载完成后,需要手动释放掉 UnityWebRequest 对象和纹理,可以使用 Destroy 或 Resources.UnloadAsset 方法来实现。
相关问题
using UnityEngine; using UnityEngine.Networking; using System.Collections; public class HttpDemo : MonoBehaviour { void Start() { StartCoroutine(GetData()); } IEnumerator GetData() { UnityWebRequest request = UnityWebRequest.Get("http://localhost:8080/data"); yield return request.SendWebRequest(); if (request.result == UnityWebRequest.Result.Success) { // 解析接口数据 string data = request.downloadHandler.text; Debug.Log("Data: " + data); } else { Debug.LogError("Http request error: " + request.error); } } }
这段代码使用了UnityWebRequest类来发送HTTP请求并获取数据。在Start()方法中,通过调用StartCoroutine()方法来执行GetData()协程。在GetData()协程中,首先创建了一个UnityWebRequest对象,并通过Get()方法设置请求的URL。然后,使用yield return语句来等待请求的响应。在请求响应后,可以通过result属性来检查请求是否成功,并通过downloadHandler.text属性获取响应数据。如果请求失败,可以通过error属性获取失败原因。
需要注意的是,使用UnityWebRequest类需要引用UnityEngine.Networking命名空间。另外,在使用协程时需要使用yield return语句来等待异步操作的完成。
用unityC#加载网页(https://remote.ruifbl.com/document/8608chanpin/room.fbx)的模型资源的代码
在Unity C#中加载外部网络上的FBX模型资源,你需要使用`WWW`类来异步下载文件,然后用`AssetDatabase.ImportAsset`导入到项目中。以下是一个简单的示例代码:
```csharp
using UnityEngine;
using System.IO;
using System.Collections.Generic;
public class LoadWebModel : MonoBehaviour
{
public string url = "https://remote.ruifbl.com/document/8608chanpin/room.fbx"; // 替换为你需要的URL
void Start()
{
StartCoroutine(LoadModelAsync());
}
IEnumerator.LoadSceneAsync(string sceneName)
{
WWW www = new WWW(url);
yield return www;
if (www.error == null)
{
// 异步下载完成后
Debug.Log("Model downloaded successfully.");
// 导入FBX到场景
string path = Application.dataPath + "/StreamingAssets/" + Path.GetFileName(www.assetUrl);
AssetDatabase.ImportAsset(path, ImportOptions.ForceUpdate);
// 加载FBX到游戏对象
GameObject modelObject = Instantiate(Resources.Load<GameObject>("YourPrefabName"));
// 或者直接加载FBX内容创建对象
// GameObject modelObject = Instantiate(AssetDatabase.LoadAssetAtPath(path, typeof(GameObject)));
// 将下载的模型添加到场景中
transform.parent = GameObject.Find("YourParentGameObject"); // 替换为你的目标父物体
}
else
{
Debug.LogError("Failed to load model: " + www.error);
}
}
}
```
在这个例子中,你需要确保Unity已经启用了StreamingAssets目录,并将"YourPrefabName"替换为FBX文件对应的预制体名称。如果FBX文件中有多个对象,记得在你的场景中有一个合适的父物体来容纳它们。
阅读全文
相关推荐
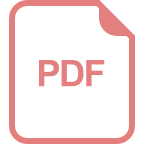
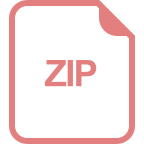
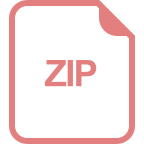













