public class CameraManager : MonoBehaviour { public GameObject target; public float height; public float distance; Vector3 pos; bool isShaking = false; public static CameraManager instance; // Use this for initialization void Start () { pos = transform.position; instance = this; } // Update is called once per frame void Update () { } public void CameraShake() { if(!isShaking) StartCoroutine(ShakeCoroutine()); } IEnumerator ShakeCoroutine() { isShaking = true; float time = 0.5f; while (time > 0) { transform.position = new Vector3(target.transform.position.x+Random.Range(-0.1f,0.1f), target.transform.position.y + height, target.transform.position.z - distance); time -= Time.deltaTime; yield return null; } isShaking = false; }
时间: 2024-02-10 11:19:23 浏览: 27
这是一个用于摄像机管理的 C# 脚本,它包含了一些摄像机的属性和操作。其中,target 是摄像机跟随的目标物体,height 是摄像机距离目标物体的高度,distance 是摄像机距离目标物体的距离,pos 是摄像机的初始位置,isShaking 表示摄像机是否正在震动,instance 是单例模式的实例。在 Start 函数中,将 pos 设置为当前摄像机的位置,将 instance 设置为当前实例。在 Update 函数中没有实现任何逻辑操作。CameraShake 函数用于触发摄像机震动效果,如果摄像机没有在震动,则启动协程函数 ShakeCoroutine。ShakeCoroutine 函数实现了摄像机的震动效果,通过不断改变摄像机的位置来模拟震动效果,最后将 isShaking 设为 false。
相关问题
public class ScrollableBackground : MonoBehaviour { [SerializeField] private float m_ScrollSpeed = -0.25f;
你好!对于这个脚本中的 `ScrollableBackground` 类,`m_ScrollSpeed` 是一个用来控制背景滚动速度的私有浮点型变量。它使用 `[SerializeField]` 属性将其标记为可以在编辑器中进行序列化和调整。默认情况下,滚动速度为 -0.25f。这意味着背景会以每帧向左滚动 0.25 个单位的速度。你还有其他问题吗?
public class PipeSpawner : MonoBehaviour { public GameObject pipePrefab; public float spawnDelay = 2f; public float minSpawnHeight = -2f; public float maxSpawnHeight = 2f; private void Start() { InvokeRepeating("SpawnPipe", 0f, spawnDelay); } private void SpawnPipe() { float randomHeight = Random.Range(minSpawnHeight, maxSpawnHeight); Vector2 spawnPosition = new Vector2(transform.position.x, randomHeight); Instantiate(pipePrefab, spawnPosition, Quaternion.identity); } } 解释这几行代码的意思
这段代码是一个管道生成器的脚本,用于在Unity游戏中生成管道。
1. `public class PipeSpawner : MonoBehaviour`:定义了一个名为PipeSpawner的公共类,并继承自MonoBehaviour类,表示这是一个组件脚本。
2. `public GameObject pipePrefab;`:定义了一个公共的GameObject类型的变量pipePrefab,用于存储预制体(管道)的引用。
3. `public float spawnDelay = 2f;`:定义了一个公共的浮点型变量spawnDelay,表示生成管道的延迟时间,默认为2秒。
4. `public float minSpawnHeight = -2f;`:定义了一个公共的浮点型变量minSpawnHeight,表示生成管道的最小高度,默认为-2。
5. `public float maxSpawnHeight = 2f;`:定义了一个公共的浮点型变量maxSpawnHeight,表示生成管道的最大高度,默认为2。
6. `private void Start()`:开始方法,在脚本启动时调用。
7. `InvokeRepeating("SpawnPipe", 0f, spawnDelay);`:使用InvokeRepeating函数,以spawnDelay为间隔时间重复调用SpawnPipe方法。即每隔spawnDelay秒调用一次SpawnPipe方法。
8. `private void SpawnPipe()`:生成管道的方法。
9. `float randomHeight = Random.Range(minSpawnHeight, maxSpawnHeight);`:在minSpawnHeight和maxSpawnHeight之间生成一个随机高度。
10. `Vector2 spawnPosition = new Vector2(transform.position.x, randomHeight);`:根据生成器的位置和随机高度,创建一个二维向量spawnPosition,表示生成管道的位置。
11. `Instantiate(pipePrefab, spawnPosition, Quaternion.identity);`:通过实例化pipePrefab预制体,在spawnPosition位置生成一个新的管道,Quaternion.identity表示不进行旋转。
相关推荐
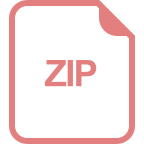
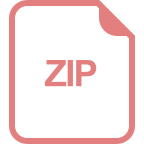
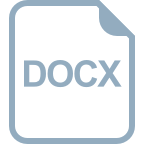













