a*算法八数码问题python
时间: 2023-07-28 20:21:19 浏览: 54
好的,以下是使用A*算法解决八数码问题的Python代码示例。
```python
from queue import PriorityQueue
# 定义状态类
class State:
def __init__(self, board, moves, manhattan):
self.board = board
self.moves = moves
self.manhattan = manhattan
# 定义优先级函数
def __lt__(self, other):
return self.manhattan + self.moves < other.manhattan + other.moves
# 判断是否为目标状态
def is_goal(self):
return self.board == [[0, 1, 2], [3, 4, 5], [6, 7, 8]]
# 获取所有可能的下一步状态
def get_neighbors(self):
neighbors = []
row, col = self.find(0)
if row > 0:
board_copy = self.copy()
board_copy[row][col], board_copy[row-1][col] = board_copy[row-1][col], board_copy[row][col]
neighbor = State(board_copy, self.moves+1, self.manhattan_distance())
neighbors.append(neighbor)
if row < 2:
board_copy = self.copy()
board_copy[row][col], board_copy[row+1][col] = board_copy[row+1][col], board_copy[row][col]
neighbor = State(board_copy, self.moves+1, self.manhattan_distance())
neighbors.append(neighbor)
if col > 0:
board_copy = self.copy()
board_copy[row][col], board_copy[row][col-1] = board_copy[row][col-1], board_copy[row][col]
neighbor = State(board_copy, self.moves+1, self.manhattan_distance())
neighbors.append(neighbor)
if col < 2:
board_copy = self.copy()
board_copy[row][col], board_copy[row][col+1] = board_copy[row][col+1], board_copy[row][col]
neighbor = State(board_copy, self.moves+1, self.manhattan_distance())
neighbors.append(neighbor)
return neighbors
# 计算曼哈顿距离
def manhattan_distance(self):
distance = 0
for i in range(3):
for j in range(3):
if self.board[i][j] == 0:
continue
row, col = self.find(self.board[i][j])
distance += abs(row-i) + abs(col-j)
return distance
# 查找指定数字的位置
def find(self, num):
for i in range(3):
for j in range(3):
if self.board[i][j] == num:
return i, j
# 复制状态
def copy(self):
board_copy = []
for i in range(3):
row = []
for j in range(3):
row.append(self.board[i][j])
board_copy.append(row)
return board_copy
# A*算法求解八数码问题
def solve(start):
start_state = State(start, 0, 0)
if start_state.is_goal():
return start_state.moves, start_state.board
frontier = PriorityQueue()
frontier.put(start_state)
explored = set()
while not frontier.empty():
state = frontier.get()
if state.is_goal():
return state.moves, state.board
explored.add(str(state.board))
for neighbor in state.get_neighbors():
if str(neighbor.board) in explored:
continue
frontier.put(neighbor)
return None
# 测试
start = [[1, 2, 3], [4, 0, 5], [6, 7, 8]]
moves, board = solve(start)
print("Minimum number of moves:", moves)
print("Final board:")
for row in board:
print(row)
```
这段代码中,我们定义了一个`State`类来表示八数码问题中的状态,其中包括当前的棋盘状态、已经移动的步数以及曼哈顿距离。我们还定义了优先级函数,用于在优先队列中按照优先级排序。在`get_neighbors`方法中,我们获取当前状态的所有可能的下一步状态,并计算曼哈顿距离。在`solve`函数中,我们使用A*算法搜索最优解,并返回最少移动步数和最终的棋盘状态。最后,我们测试了一组初始状态,求解八数码问题并输出结果。
注意,这段代码中的`queue.PriorityQueue`是Python中的优先队列实现,使用方法类似于普通队列。如果你对优先队列不太熟悉,可以参考Python文档中的说明。
相关推荐









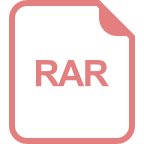
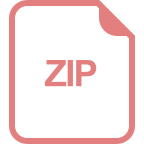
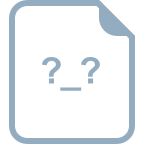
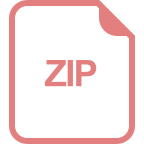