CANopennode的canopen.h文件在哪
时间: 2023-08-18 10:04:06 浏览: 86
CANopennode的canopen.h文件一般位于CANopennode库的include目录下。在Linux系统中,可以使用以下命令查找canopen.h文件的位置:
```
sudo find / -name canopen.h
```
在Windows系统中,可以在CANopennode库的安装目录下查找canopen.h文件。
如果你使用的是CANopennode的源码,可以在源码目录下的include目录中找到canopen.h文件。如果你使用的是预编译的库文件,则需要将库文件添加到Qt项目中,并在.pro文件中添加相关配置,例如:
```
INCLUDEPATH += /path/to/canopennode/include
LIBS += /path/to/canopennode/libcanopennode.a
```
相关问题
CANopennode的CANopen.h文件
以下是CANopennode中CANopen.h文件的代码,仅供参考:
```c
/**
* @file CANopen.h
* @author CANopenNode, LLC
* @version 4.0.0
* @date 25 Feb 2021
* @brief Header file for CANopenNode stack.
*
* This file contains all definitions and declarations for the CANopenNode
* stack that are relevant for the application. The data types and function
* prototypes defined here must be used in the user application code.
*
* @copyright Copyright (c) CANopenNode, LLC
* @defgroup CO_CANopen CANopen
* @{
*/
#ifndef CO_CANOPEN_H
#define CO_CANOPEN_H
#ifdef __cplusplus
extern "C" {
#endif
/* Includes ------------------------------------------------------------------*/
#include <stdint.h>
#include <stdbool.h>
#include <stddef.h>
/* Exported defines ----------------------------------------------------------*/
/**
* Macros for standard data types.
*
* Macros for data types defined in stdint.h and stdbool.h. These macros should
* be used in the application code instead of direct use of the types.
*
* @ingroup CO_CANopen_301
* @{
*/
#ifndef NULL
#define NULL ((void*) 0)
#endif
#define int8_t int8_t
#define uint8_t uint8_t
#define int16_t int16_t
#define uint16_t uint16_t
#define int32_t int32_t
#define uint32_t uint32_t
#define bool_t bool
#define true true
#define false false
/** @} */
/* Exported types ------------------------------------------------------------*/
/** CAN message structure as in CAN hardware. */
typedef struct {
uint16_t ident; /**< 11-bit identifier, bits 10..0 are used. */
uint8_t DLC; /**< Data length code: 0..8. */
uint8_t data[8];/**< Data field. Bytes 0..7 are used. */
} CO_CANrxMsg_t;
/** CAN transmit message structure. */
typedef struct {
uint16_t ident; /**< 11-bit identifier, bits 10..0 are used. */
bool_t rtr; /**< RTR (Remote transmission request). */
bool_t ext; /**< EXT (Extended identifier, 29-bit). */
uint8_t DLC; /**< Data length code: 0..8. */
uint8_t data[8];/**< Data field. Bytes 0..7 are used. */
} CO_CANtx_t;
/**
* CAN message reception callback function.
*
* Function is called, when new message is received and passed to the CANopenNode.
*
* @param[in] msg Received message with necessary informations.
*/
typedef void (*CO_CANrx_callback_t)(const CO_CANrxMsg_t *msg);
/**
* CANopen receive message structure.
*
* Object is storage for received CAN message and additional informations.
*/
typedef struct {
const uint16_t ident; /**< Standard CAN Identifier or Extended CAN Identifier. */
const uint16_t mask; /**< Mask, to determine which bits of the identifier are significant. */
CO_CANrx_callback_t pCANrx_callback; /**< Pointer to function, which will be called, when CAN message with specified identifier will be received. */
} CO_CANrx_t;
/** CANopen object with static configuration. */
typedef const struct {
const uint16_t index; /**< Index of object in Object Dictionary. */
const uint8_t subIndex;/**< Subindex of object in Object Dictionary. */
const uint8_t attribute;/**< Attribute of Object Dictionary entry. */
const uint32_t length; /**< Data length in bytes. */
void* const pData; /**< Pointer to data. */
const CO_CANrx_callback_t pFunct; /**< Pointer to function, which will be called on RPDO reception. */
} CO_ObjDict_t;
/* Exported variables --------------------------------------------------------*/
/* Exported functions ------------------------------------------------------- */
/**
* Initialize CANopenNode stack.
*
* Function must be called in the communication reset section.
*
* Function configures:
* - data storage for CANopen objects
* - LSS slave
* - LSS master
* - SDO server
* - SDO client
* - Emergency object
* - Heartbeat consumer
* - NMT object
* - Time object
* - SYNC objects
* - RPDO objects and corresponding CAN reception filters
* - TPDO objects and corresponding CAN transmission functions
* - SDO server objects and corresponding CAN reception filters and CAN transmission functions
*
* Function must be called before any other CANopenNode function.
*
* @param[out] ppData Pointer to pointer to data memory for CANopen objects.
* If *ppData==NULL, memory for CANopen objects will be
* allocated. If *ppData!=NULL, memory must be
* statically allocated by application and available
* during whole lifetime of the CANopenNode.
* Pointer ppData is pointing to the first free byte
* after the allocated memory.
* @param[in] nodeId CANopen Node ID.
* @param[in] bitRate CAN bit-rate.
* @param[in] CANrx_callback Pointer to function, which will be called, when CAN
* message with appropriate identifier is received.
* It can be NULL.
*
* @return 0: Operation completed successfully.
* @return -1: Error in function parameters.
* @return -2: Error: CO_LSSslave_init() failed.
* @return -3: Error: CO_SDOserver_init() failed.
* @return -4: Error: CO_EM_init() failed.
* @return -5: Error: CO_NMT_init() failed.
* @return -6: Error: CO_HBconsumer_init() failed.
* @return -7: Error: CO_TIME_init() failed.
* @return -8: Error: CO_SYNC_init() failed.
* @return -9: Error: CO_RPDO_init() failed.
* @return -10: Error: CO_TPDO_init() failed.
*/
int16_t CO_init(
uint8_t** const ppData,
const uint8_t nodeId,
const uint16_t bitRate,
CO_CANrx_callback_t CANrx_callback);
/**
* CAN receive function.
*
* Function must be called, when CAN message is received.
* For every received message, function will try to find appropriate
* CO_CANrx_t object (with CO_CANrx_t.ident equal to CAN identifier (msg->ident & CO_CANrx_t.mask).
*
* For more information see file CO_CAN.c
*
* @param[in] CANrxMsg Pointer to received message.
* @return 0: Operation completed successfully.
* @return -1: Error: Received message is NULL.
* @return -2: Error: No message received.
* @return -3: Error: Message received, but not processed.
*/
int16_t CO_CANrxBufferProcess(CO_CANrxMsg_t* const CANrxMsg);
/**
* CAN send function.
*
* For more information see file CO_CAN.c
*
* @param[in] COB_ID CAN identifier.
* @param[in] len Length of CAN message in bytes (0 to 8).
* @param[in] data Pointer to CAN message data bytes.
* @param[in] rtr Request for transmission. If true, then this is only request for transmission,
* no data are sent (length and data pointer are ignored).
* @return 0: Operation completed successfully.
* @return -1: Error: Wrong arguments.
* @return -2: Error: Previous message is still waiting for buffer.
* @return -3: Error: Timeout in transmission of CAN message.
*/
int16_t CO_CANsend(
const uint16_t COB_ID,
const uint8_t len,
const uint8_t* const data,
const bool_t rtr);
/**
* Calculate CAN bit-timing values from register values.
*
* Function calculates values for CAN bit timing register and optionally for
* CAN controller bit rate prescaler.
*
* For more information see file CO_driver.c
*
* @param[in] brp CAN controller bit rate prescaler.
* @param[in] tseg1 Time segment 1 (0 to 15).
* @param[in] tseg2 Time segment 2 (0 to 7).
* @param[in] sjw Resynchronization jump width (0 to 3).
* @param[out] pSyncTimeMicroseconds Synchronization time in micro seconds.
* @param[out] pBitRatePrescaler Bit rate prescaler.
* @return 0: Operation completed successfully.
* @return -1: Error: Wrong arguments.
*/
int16_t CO_CANbitRateCalc(
const uint16_t brp,
const uint8_t tseg1,
const uint8_t tseg2,
const uint8_t sjw,
uint32_t * const pSyncTimeMicroseconds,
uint16_t * const pBitRatePrescaler);
#ifdef __cplusplus
}
#endif /* __cplusplus */
#endif /* CO_CANOPEN_H */
/**
* @}
*/
/* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ */
/* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ */
/* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ */
/**
* @defgroup CO_CANopen_301 CANopen 3.0.1 stack implementation
*
* CANopen 3.0.1 stack implementation. File CO_ODinterface.h is not included
* here and must be included separately.
*
* @todo CO_CANrxMsg_t is not used anymore. Remove it in future.
*
* @defgroup CO_CANopen_301_Macros Macros
* @ingroup CO_CANopen_301
* @{
* CANopen error codes
* -------------------
* 0x00000000 - no error
* 0x05030000 - toggle bit not alternated
* 0x05040000 - SDO protocol timed out
* 0x05040001 - SDO protocol bad initial value
* 0x05040002 - SDO protocol bad object dictionary address
* 0x05040003 - SDO protocol bad data type
* 0x05040004 - SDO protocol bad data size
* 0x05040005 - SDO protocol bad data value
* 0x06010000 - Object dictionary not found
* 0x06010001 - Object cannot be mapped to the PDO
* 0x06010002 - PDO length exceeded
* 0x06020000 - Object does not exist in the object dictionary
* 0x06040041 - Object cannot be mapped to the PDO
* 0x06040042 - the number and length of the objects to be mapped would exceed PDO length
* 0x06060000 - Access failed due to an hardware error
* 0x06070010 - Data type does not match, length of service parameter does not match
* 0x06070012 - Data type does not match, length of service parameter too high
* 0x06070013 - Data type does not match, length of service parameter too low
* 0x06090011 - Subindex does not exist
* 0x06090030 - Value range of parameter exceeded (only for write access)
* 0x06090031 - Value of parameter written too high
* 0x06090032 - Value of parameter written too low
* 0x06090036 - Maximum value is less than minimum value
* 0x08000000 - General error
* 0x08000020 - Data cannot be transferred or stored to the application
* 0x08000021 - Data cannot be transferred or stored to the application because of local control
* 0x08000022 - Data cannot be transferred or stored to the application because of the present device state
* 0x08000023 - Object dictionary dynamic generation fails or no object dictionary is present (e.g. object dictionary is generated from file and generation fails because of an file error)
* 0x08000024 - No data available
* 0x08000025 - General error in the device
* 0x08000026 - Data cannot be transferred or stored to the application because of some dependency
* 0x08000027 - General error reason in the device
* 0x08000028 - Service is not available
* 0x08000029 - Attribute not supported
* 0x0800002A - The value of the parameter written exceeds the range of values that can be written
* 0x0800002B - Data cannot be transferred or stored to the application because of local control, specific application needed
* 0x0800002C - Data cannot be transferred or stored to the application because of the present device state, specific application needed
* 0x0800002D - Object dictionary not present, specific application needed
* 0x0800002E - No data available, specific application needed
* 0x0800002F - Data cannot be transferred or stored to the application because of some dependency, specific application needed
* 0x08000030 - Service is not available, specific application needed
* 0x08000031 - Attribute not supported, specific application needed
* 0x0F004000 - Unexpected SYNC data length
* 0x0F004001 - SYNC timeout
* 0x0F004002 - Unexpected SYNC data
* 0x0F004003 - Heartbeat consumer timeout
* 0x0F004004 - PDO not processed due to length error
* 0x0F004005 - PDO length exceeded
* 0x0F004006 - DAM MPDO not processed, destination object not available
* 0x0F004007 - Unexpected emergency object
* 0x0F004008 - Error in error register
* 0x0F004009 - Additional functions not available
* 0x0F00400A - Parameter incompatibility (configuration or mode)
* 0x0F00400B - CANopen service not supported
* 0x0F00400C - CANopen invalid state transition
* 0x0F00400D - CAN frame received is wrong
* 0x0F00400E - PDO not processed, communication object not available
* 0x0F00400F - PDO length exceeded
* 0x0F004010 - Data type of service parameter does not match
* 0x0F004011 - Data type of service parameter is not implemented
CANopennode的CANopen.h文件怎么用
CANopennode是一个用于实现CANopen协议的开源软件库。CANopen.h是CANopennode库的头文件,其中包含了CANopen协议栈的各种数据结构和函数声明。要使用CANopen.h文件,你需要按照以下步骤进行操作:
1. 下载并安装CANopennode库。你可以从CANopennode的官方网站 (http://www.canopennode.org/) 下载最新版本的CANopennode库。
2. 将CANopen.h文件包含到你的代码中。在你的代码中添加以下语句:
```
#include "CANopen.h"
```
3. 初始化CANopen协议栈。在你的代码中调用以下函数:
```
CO_ReturnError_t err = CO_init();
if(err != CO_ERROR_NO){
//错误处理代码
}
```
4. 使用CANopen协议栈。通过调用CANopen.h文件中定义的函数,可以实现CANopen协议栈的各种功能。例如:
```
CO_NMT_sendCommand(CO->NMT, CO_NMT_ENTER_PRE_OPERATIONAL);
```
以上是使用CANopen.h文件的基本步骤,具体使用方法可以参考CANopennode库的官方文档。
阅读全文
相关推荐


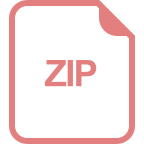
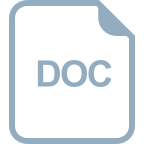
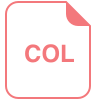








