triomino拼图问题c语言
时间: 2023-11-26 09:04:08 浏览: 115
Triomino拼图问题是一个经典的计算机科学问题,它可以通过C语言来实现。简单来说,Triomino拼图问题是将一个2^n * 2^n的正方形分成L形态的三格块,然后用特定的方式填充这些块,使得整个正方形没有留下任何空白。以下是一个简单的C语言程序,用于解决Triomino拼图问题。
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define EMPTY -1
#define BLOCK 0
#define L_BLOCK 1
void triomino(int **board, int size, int row, int col, int missing_row, int missing_col, int type);
int main() {
int size, row, col;
printf("Enter the size of the board: ");
scanf("%d", &size);
printf("Enter the row position of the missing square: ");
scanf("%d", &row);
printf("Enter the column position of the missing square: ");
scanf("%d", &col);
int **board = (int **)malloc(size * sizeof(int *));
for (int i = 0; i < size; i++)
board[i] = (int *)malloc(size * sizeof(int));
// 初始化棋盘
for (int i = 0; i < size; i++)
for (int j = 0; j < size; j++)
board[i][j] = EMPTY;
// 放置缺失的方块
board[row][col] = BLOCK;
// 解决问题
triomino(board, size, 0, 0, row, col, L_BLOCK);
// 打印解决方案
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++)
printf("%2d ", board[i][j]);
printf("\n");
}
// 释放内存
for (int i = 0; i < size; i++)
free(board[i]);
free(board);
return 0;
}
void triomino(int **board, int size, int row, int col, int missing_row, int missing_col, int type) {
if (size == 1)
return;
int half_size = size / 2;
int center_row = row + half_size - 1;
int center_col = col + half_size - 1;
// 放置L形块
int block_type = type;
if (missing_row < center_row) {
if (missing_col < center_col) {
board[center_row][center_col + 1] = block_type;
board[center_row + 1][center_col] = block_type;
}
else {
board[center_row][center_col] = block_type;
board[center_row + 1][center_col] = block_type;
}
}
else {
if (missing_col < center_col) {
board[center_row][center_col + 1] = block_type;
board[center_row][center_col] = block_type;
}
else {
board[center_row + 1][center_col] = block_type;
board[center_row][center_col] = block_type;
}
}
// 递归解决四个子问题
triomino(board, half_size, row, col, center_row, center_col, type);
triomino(board, half_size, row, center_col + 1, center_row, center_col + 1, type);
triomino(board, half_size, center_row + 1, col, center_row + 1, center_col, type);
triomino(board, half_size, center_row + 1, center_col + 1, center_row + 1, center_col + 1, type);
// 处理缺失的方块
if (missing_row <= center_row && missing_col <= center_col)
triomino(board, half_size, row, col, missing_row, missing_col, L_BLOCK);
else if (missing_row <= center_row && missing_col > center_col)
triomino(board, half_size, row, center_col + 1, missing_row, missing_col, L_BLOCK);
else if (missing_row > center_row && missing_col <= center_col)
triomino(board, half_size, center_row + 1, col, missing_row, missing_col, L_BLOCK);
else
triomino(board, half_size, center_row + 1, center_col + 1, missing_row, missing_col, L_BLOCK);
}
```
该程序使用递归算法来解决Triomino拼图问题。它首先初始化棋盘,并将缺失的方块放置在适当的位置。然后,它使用triomino函数来递归解决问题。该函数将棋盘分成四个子问题,并在中心处放置L形块。然后,它递归地解决四个子问题,并在必要时继续处理缺失的方块。最后,该程序打印出解决方案,并释放内存。
阅读全文
相关推荐


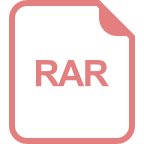










