linux系统编写C程序,使用Linux中的IPC机制,完成 “石头、剪子、布”的游戏。写一下实验步骤
时间: 2024-03-09 13:44:58 浏览: 385
好的,以下是实验步骤:
1. 创建两个进程,分别作为游戏的双方参与者,可以使用fork()函数创建进程。
2. 使用管道(pipe)或消息队列(message queue)作为进程间通信的方式,传递游戏信息。
3. 让每个进程随机生成“石头、剪子、布”中的一个,通过IPC机制将自己选择的对象发送给对手。
4. 对手接收到对方的选择后,进行比较,输出胜负结果。
5. 游戏结束后,关闭管道或消息队列,退出进程。
实现具体细节可以参考以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <time.h>
#define MSG_SIZE 64
#define PLAYER1 1
#define PLAYER2 2
#define GAME_OVER -1
struct message {
long msg_type;
char msg_text[MSG_SIZE];
};
// 生成随机数,表示玩家的选择
int generate_choice() {
srand(time(NULL));
int choice = rand() % 3;
return choice;
}
// 判断胜负
int judge_winner(int choice1, int choice2) {
if (choice1 == choice2) {
return 0;
}
if ((choice1 == 0 && choice2 == 1) || (choice1 == 1 && choice2 == 2) || (choice1 == 2 && choice2 == 0)) {
return PLAYER1;
} else {
return PLAYER2;
}
}
int main() {
int msgid;
struct message msg;
int choice1, choice2;
int winner;
// 创建消息队列
msgid = msgget(IPC_PRIVATE, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget error");
exit(EXIT_FAILURE);
}
// 创建子进程
pid_t pid = fork();
if (pid < 0) {
perror("fork error");
exit(EXIT_FAILURE);
}
if (pid == 0) {
// 子进程作为玩家1
while (1) {
printf("Please choose your object(0 for rock, 1 for scissors, 2 for paper): ");
scanf("%d", &choice1);
if (choice1 < 0 || choice1 > 2) {
printf("Invalid choice, please choose again.\n");
continue;
}
// 将选择的对象发送给玩家2
msg.msg_type = PLAYER2;
sprintf(msg.msg_text, "%d", choice1);
msgsnd(msgid, &msg, sizeof(msg.msg_text), 0);
// 接收玩家2的选择
msgrcv(msgid, &msg, sizeof(msg.msg_text), PLAYER1, 0);
choice2 = atoi(msg.msg_text);
// 判断胜负
winner = judge_winner(choice1, choice2);
if (winner == PLAYER1) {
printf("Congratulation! You win!\n");
} else if (winner == PLAYER2) {
printf("Sorry, you lose.\n");
} else {
printf("Draw.\n");
}
// 判断游戏是否结束
if (winner == GAME_OVER) {
printf("Game over.\n");
break;
}
}
} else {
// 父进程作为玩家2
while (1) {
// 接收玩家1的选择
msgrcv(msgid, &msg, sizeof(msg.msg_text), PLAYER2, 0);
choice1 = atoi(msg.msg_text);
// 生成自己的选择
choice2 = generate_choice();
printf("I choose %d.\n", choice2);
// 将选择的对象发送给玩家1
msg.msg_type = PLAYER1;
sprintf(msg.msg_text, "%d", choice2);
msgsnd(msgid, &msg, sizeof(msg.msg_text), 0);
// 判断胜负
winner = judge_winner(choice1, choice2);
if (winner == PLAYER2) {
printf("Congratulation! You win!\n");
} else if (winner == PLAYER1) {
printf("Sorry, you lose.\n");
} else {
printf("Draw.\n");
}
// 判断游戏是否结束
if (winner == GAME_OVER) {
printf("Game over.\n");
break;
}
}
}
// 删除消息队列
msgctl(msgid, IPC_RMID, NULL);
return 0;
}
```
希望能帮到你。
阅读全文
相关推荐

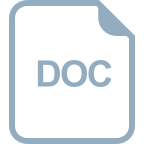
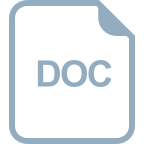










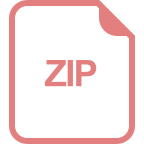
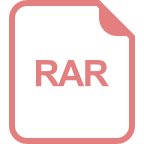

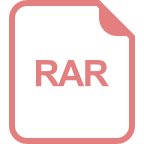

