)编写C程序,使用Linux中的IPC机制,完成 “石头、剪子、布”的游戏。
时间: 2024-11-30 22:23:05 浏览: 38
在Linux系统中,我们可以使用Inter-Process Communication (IPC)机制,如管道(pipe)、消息队列(msg_queue)、信号量(semaphore)或共享内存(shared memory),来创建一个进程间的游戏应用,比如“石头、剪刀、布”。以下是简化的步骤:
1. **创建服务器进程**:
- 使用socket通信或者共享内存来接收客户端的消息。
- 设计游戏规则逻辑,包括接受玩家输入(石头、剪刀、布),判断胜负并返回结果。
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/socket.h>
#include <sys/types.h>
#include <string.h>
// 定义游戏逻辑函数
int compare_moves(char server_move, char client_move);
int main() {
// ... (初始化socket或共享内存)
// 接收客户端连接并处理请求
while (true) {
// 读取客户端输入
// 调用比较函数判断结果
// 返回结果给客户端
}
return 0;
}
char compare_moves(char server_move, char client_move) {
// 石头对剪刀赢,剪刀对布赢,布对石头赢...
}
```
2. **创建客户端进程**:
- 发送请求(玩家选择)到服务器进程,并等待响应。
- 更新UI显示比赛结果。
```c
#include <unistd.h>
#include <sys/socket.h>
#include <arpa/inet.h>
void send_request(int sock, char choice) {
// ... (发送选择到服务器)
}
int main() {
int sock = socket(AF_INET, SOCK_STREAM, 0);
// ... (连接到服务器)
char player_choice;
// ... (获取用户输入)
send_request(sock, player_choice);
// ... (接收服务器回复并显示结果)
close(sock);
return 0;
}
```
阅读全文
相关推荐

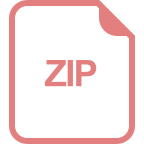















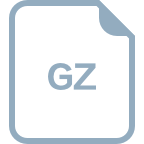