编写C程序,使用Linux中的IPC机制,完成 “石头、剪子、布”的游戏。
时间: 2023-06-12 12:04:58 浏览: 266
这里提供一种使用共享内存和信号量实现的方法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <sys/sem.h>
#include <time.h>
#define SHM_KEY 0x1234
#define SEM_KEY 0x5678
#define ROCK 0
#define SCISSORS 1
#define PAPER 2
#define WIN 0
#define LOSE 1
#define DRAW 2
typedef struct {
int player_choice;
int computer_choice;
int result;
} GameData;
void set_semvalue(int sem_id, int value) {
union semun {
int val;
struct semid_ds *buf;
unsigned short *array;
} sem_union;
sem_union.val = value;
if (semctl(sem_id, 0, SETVAL, sem_union) == -1) {
perror("semctl");
exit(EXIT_FAILURE);
}
}
void sem_wait(int sem_id) {
struct sembuf sem_b;
sem_b.sem_num = 0;
sem_b.sem_op = -1;
sem_b.sem_flg = SEM_UNDO;
if (semop(sem_id, &sem_b, 1) == -1) {
perror("semop");
exit(EXIT_FAILURE);
}
}
void sem_signal(int sem_id) {
struct sembuf sem_b;
sem_b.sem_num = 0;
sem_b.sem_op = 1;
sem_b.sem_flg = SEM_UNDO;
if (semop(sem_id, &sem_b, 1) == -1) {
perror("semop");
exit(EXIT_FAILURE);
}
}
int main() {
srand(time(NULL));
int shm_id = shmget(SHM_KEY, sizeof(GameData), IPC_CREAT | 0666);
if (shm_id == -1) {
perror("shmget");
exit(EXIT_FAILURE);
}
GameData *game_data = shmat(shm_id, NULL, 0);
if (game_data == (GameData *) -1) {
perror("shmat");
exit(EXIT_FAILURE);
}
int sem_id = semget(SEM_KEY, 1, IPC_CREAT | 0666);
if (sem_id == -1) {
perror("semget");
exit(EXIT_FAILURE);
}
set_semvalue(sem_id, 0);
while (1) {
printf("Please input your choice (0 for rock, 1 for scissors, 2 for paper): ");
scanf("%d", &(game_data->player_choice));
game_data->computer_choice = rand() % 3;
printf("You chose %d, computer chose %d\n", game_data->player_choice, game_data->computer_choice);
if (game_data->player_choice == ROCK) {
if (game_data->computer_choice == ROCK) {
game_data->result = DRAW;
} else if (game_data->computer_choice == SCISSORS) {
game_data->result = WIN;
} else if (game_data->computer_choice == PAPER) {
game_data->result = LOSE;
}
} else if (game_data->player_choice == SCISSORS) {
if (game_data->computer_choice == ROCK) {
game_data->result = LOSE;
} else if (game_data->computer_choice == SCISSORS) {
game_data->result = DRAW;
} else if (game_data->computer_choice == PAPER) {
game_data->result = WIN;
}
} else if (game_data->player_choice == PAPER) {
if (game_data->computer_choice == ROCK) {
game_data->result = WIN;
} else if (game_data->computer_choice == SCISSORS) {
game_data->result = LOSE;
} else if (game_data->computer_choice == PAPER) {
game_data->result = DRAW;
}
}
sem_signal(sem_id);
sem_wait(sem_id);
if (game_data->result == WIN) {
printf("You win!\n");
} else if (game_data->result == LOSE) {
printf("You lose!\n");
} else {
printf("It's a draw!\n");
}
}
if (shmdt(game_data) == -1) {
perror("shmdt");
exit(EXIT_FAILURE);
}
if (shmctl(shm_id, IPC_RMID, 0) == -1) {
perror("shmctl");
exit(EXIT_FAILURE);
}
if (semctl(sem_id, 0, IPC_RMID) == -1) {
perror("semctl");
exit(EXIT_FAILURE);
}
return 0;
}
```
在该程序中,使用了共享内存存储游戏数据,使用信号量实现了进程间同步。在主循环中,用户输入自己的选择后,程序随机生成计算机的选择,并根据规则判断胜负,并将结果存储在共享内存中。然后使用信号量通知另一个进程(如果有的话)可以读取共享内存中的结果了,自己则使用信号量等待结果的到来。最后根据结果输出胜负信息。
阅读全文
相关推荐
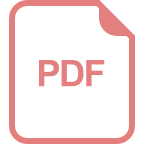













