编写C程序,使用Linux中的消息队列机制,完成 “石头、剪子、布”的游戏。要求: 回合数:接收用户输入 打印结果: 每回合的结果,最终的结果
时间: 2024-02-26 08:58:58 浏览: 110
好的,以下是使用 Linux 消息队列机制编写的石头剪子布游戏的 C 语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <errno.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <sys/types.h>
#define MAX_ROUNDS 10 // 最大回合数
#define TYPE_PLAYER 1 // 玩家消息类型
#define TYPE_COMPUTER 2 // 电脑消息类型
struct msgbuf {
long mtype; // 消息类型
int gesture; // 手势
};
char *get_gesture(int gesture) {
switch (gesture) {
case 1:
return "石头";
case 2:
return "剪刀";
case 3:
return "布";
default:
return NULL;
}
}
int main() {
int player_score = 0, computer_score = 0; // 玩家和电脑的得分
int rounds = 0; // 当前回合数
int msgid; // 消息队列 ID
key_t key = ftok(".", 'a'); // 生成消息队列的键值
// 创建消息队列
if ((msgid = msgget(key, IPC_CREAT | 0666)) == -1) {
perror("msgget error");
exit(EXIT_FAILURE);
}
// 游戏开始提示
printf("欢迎来玩石头剪刀布游戏!\n");
// 循环进行游戏
while (rounds < MAX_ROUNDS) {
struct msgbuf player_msg, computer_msg;
// 玩家出手势并发送消息
printf("第 %d 回合,请出手势(1.石头 2.剪刀 3.布):", rounds + 1);
scanf("%d", &player_msg.gesture);
player_msg.mtype = TYPE_PLAYER;
if (msgsnd(msgid, &player_msg, sizeof(player_msg.gesture), 0) == -1) {
perror("msgsnd error");
exit(EXIT_FAILURE);
}
// 接收电脑的手势并比较胜负
if (msgrcv(msgid, &computer_msg, sizeof(computer_msg.gesture), TYPE_COMPUTER, 0) == -1) {
perror("msgrcv error");
exit(EXIT_FAILURE);
}
printf("电脑出了:%s,", get_gesture(computer_msg.gesture));
if (player_msg.gesture == computer_msg.gesture) {
printf("平局!\n");
} else if ((player_msg.gesture == 1 && computer_msg.gesture == 2) ||
(player_msg.gesture == 2 && computer_msg.gesture == 3) ||
(player_msg.gesture == 3 && computer_msg.gesture == 1)) {
printf("你赢了!\n");
player_score++;
} else {
printf("你输了!\n");
computer_score++;
}
rounds++; // 回合数加一
}
// 输出最终结果
printf("游戏结束!你的得分:%d,电脑的得分:%d。\n", player_score, computer_score);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl error");
exit(EXIT_FAILURE);
}
return 0;
}
```
在上述代码中,我们使用了 `msgget` 创建消息队列,使用 `msgsnd` 发送消息,使用 `msgrcv` 接收消息,并使用 `msgctl` 删除消息队列。程序中定义了两种消息类型,一种是玩家消息类型,一种是电脑消息类型。每回合玩家出手势后,会将手势封装成玩家消息并发送到消息队列中,然后等待接收电脑的手势,将电脑的手势封装成电脑消息并发送到消息队列中,最后比较胜负并输出结果。最后,游戏结束后会删除消息队列。
希望这个程序能够带给你一些帮助!
阅读全文
相关推荐
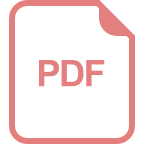






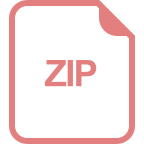
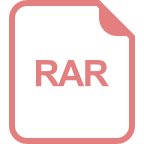
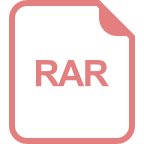
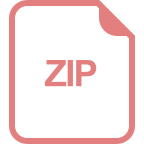
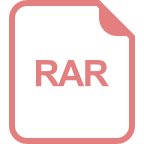
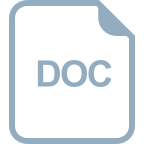
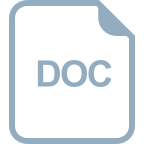
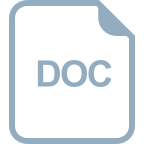