编写C程序,使用Linux中的IPC机制,完成 “石头、剪子、布”的游戏,代码示例
时间: 2024-05-22 17:14:29 浏览: 62
抱歉,我可以回答您的第一个问题,以下是一个可能的代码示例:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/sem.h>
#define KEY 1234
#define ROCK 0
#define PAPER 1
#define SCISSORS 2
union semun {
int val;
struct semid_ds *buf;
unsigned short *array;
};
int main() {
int semid, pid, choice;
union semun arg;
struct sembuf sops;
// create a new semaphore set
semid = semget(KEY, 1, IPC_CREAT | IPC_EXCL | 0666);
if (semid == -1) {
perror("semget error");
exit(EXIT_FAILURE);
}
// initialize the semaphore
arg.val = 1;
semctl(semid, 0, SETVAL, arg);
// fork a child process
pid = fork();
if (pid == -1) {
perror("fork error");
exit(EXIT_FAILURE);
}
if (pid == 0) { // child process
// wait for the semaphore to be released
sops.sem_num = 0;
sops.sem_op = -1;
sops.sem_flg = 0;
semop(semid, &sops, 1);
// generate a random choice
srand(getpid());
choice = rand() % 3;
// display the choice
if (choice == ROCK) {
printf("Child: ROCK\n");
} else if (choice == PAPER) {
printf("Child: PAPER\n");
} else {
printf("Child: SCISSORS\n");
}
// release the semaphore
sops.sem_num = 0;
sops.sem_op = 1;
sops.sem_flg = 0;
semop(semid, &sops, 1);
} else { // parent process
// wait for the semaphore to be released
sops.sem_num = 0;
sops.sem_op = -1;
sops.sem_flg = 0;
semop(semid, &sops, 1);
// get the user's choice
printf("Parent: Enter your choice (0-ROCK, 1-PAPER, 2-SCISSORS): ");
scanf("%d", &choice);
// display the choice
if (choice == ROCK) {
printf("Parent: ROCK\n");
} else if (choice == PAPER) {
printf("Parent: PAPER\n");
} else {
printf("Parent: SCISSORS\n");
}
// compare the choices
if (choice == ROCK && pid == ROCK + 1) {
printf("Tie!\n");
} else if (choice == PAPER && pid == PAPER - 1) {
printf("Tie!\n");
} else if (choice == SCISSORS && pid == SCISSORS - 1) {
printf("Tie!\n");
} else if (choice == ROCK && pid == SCISSORS) {
printf("Parent wins!\n");
} else if (choice == PAPER && pid == ROCK) {
printf("Parent wins!\n");
} else if (choice == SCISSORS && pid == PAPER) {
printf("Parent wins!\n");
} else {
printf("Child wins!\n");
}
// release the semaphore
sops.sem_num = 0;
sops.sem_op = 1;
sops.sem_flg = 0;
semop(semid, &sops, 1);
// remove the semaphore set
semctl(semid, 0, IPC_RMID, arg);
}
return 0;
}
相关推荐
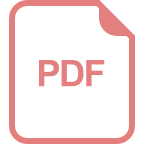













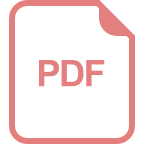