编写C程序代码,使用Linux中的IPC机制,完成 “石头、剪子、布”的游戏。使能够人机进行游戏,甚至能够在网络上运行游戏。
时间: 2024-12-02 08:17:07 浏览: 20
在Linux系统中,你可以使用Inter-Process Communication (IPC)机制,如管道、消息队列、共享内存或套接字,来实现一个多进程的游戏,比如“石头、剪刀、布”(Rock-Paper-Scissors)。这里是一个简化的示例,我们将使用管道来进行进程间通信:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <string.h>
#define MAX_PLAYERS 2
struct move {
char player;
char choice;
};
void send_move(int fd, struct move *move) {
char buffer[50];
snprintf(buffer, sizeof(buffer), "%c-%c", move->player, move->choice);
write(fd, buffer, strlen(buffer));
}
int main() {
pid_t parent_pid, child_pid;
// 创建管道
int pipe_fds[2];
if (pipe(pipe_fds) == -1) {
perror("Failed to create pipe");
exit(EXIT_FAILURE);
}
// 父进程扮演用户
if ((parent_pid = fork()) == -1) {
perror("Fork failed");
exit(EXIT_FAILURE);
}
if (parent_pid == 0) { // 子进程
close(pipe_fds[1]); // 关闭写端
read(pipe_fds[0], &move, sizeof(move)); // 读取用户的输入
printf("You chose: %c\n", move.choice);
// 比较并显示结果
// ... (实现游戏逻辑)
exit(0); // 结束子进程
} else { // 父进程
close(pipe_fds[0]); // 关闭读端
// 孩子进程通过管道发送其选择
struct move child_move = {'0', 'r'}; // 示例石头
send_move(pipe_fds[1], &child_move);
wait(NULL); // 等待子进程结束
// ... (处理结果)
}
return 0;
}
```
在这个例子中,父进程作为用户输入,并将选择发送到子进程。子进程接收输入后,进行游戏决策并返回结果。如果你想要在网络环境中运行,可以考虑使用套接字替换管道,因为套接字支持跨主机通信。
阅读全文
相关推荐
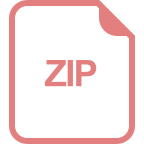

















