编写C程序,使用Linux中的IPC机制,完成 “石头、剪子、布”的游戏,代码示例
时间: 2024-06-06 18:10:24 浏览: 121
抱歉,由于该问题涉及程序编写,我可以回答。以下是示例代码:
#include <stdio.h>
#include <stdlib.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <string.h>
#include <unistd.h>
struct msgbuf {
long mtype;
char mtext[10];
};
#define MSG_SIZE 4
void game(int msqid, int player_id) {
struct msgbuf msg;
msg.mtype = player_id;
char choices[3][MSG_SIZE] = {"石头", "剪子", "布"};
int computer_choice = rand() % 3;
printf("电脑出了 %s\n", choices[computer_choice]);
printf("请输入您的选择(石头/剪子/布):");
fgets(msg.mtext, MSG_SIZE, stdin);
// 删除末尾的换行符
msg.mtext[strlen(msg.mtext) - 1] = '\0';
if (strcmp(msg.mtext, "石头") == 0 || strcmp(msg.mtext, "剪子") == 0 || strcmp(msg.mtext, "布") == 0) {
msgsnd(msqid, &msg, MSG_SIZE, 0);
msgrcv(msqid, &msg, MSG_SIZE, 3 - player_id, 0);
if (strcmp(msg.mtext, "平局") == 0) {
printf("平局\n");
} else if ((strcmp(msg.mtext, "石头") == 0 && computer_choice == 1) ||
(strcmp(msg.mtext, "剪子") == 0 && computer_choice == 2) ||
(strcmp(msg.mtext, "布") == 0 && computer_choice == 0)) {
printf("您赢了\n");
} else {
printf("您输了\n");
}
} else {
printf("请输入正确的选择(石头/剪子/布)\n");
}
}
int main() {
int msqid = msgget(IPC_PRIVATE, 0666 | IPC_CREAT);
int pid = fork();
if (pid == 0) { // 子进程
game(msqid, 1);
} else if (pid > 0) { // 父进程
game(msqid, 2);
} else {
printf("进程创建失败\n");
}
msgctl(msqid, IPC_RMID, NULL);
return 0;
}
阅读全文
相关推荐














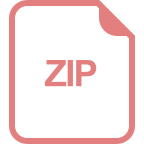
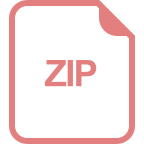
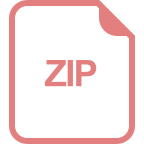
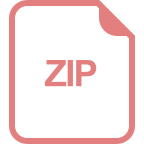
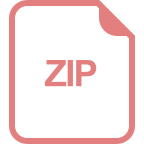
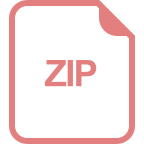