class MyItem : public QGraphicsItem { public: MyItem(QGraphicsItem *parent = nullptr) : QGraphicsItem(parent) { // 设置图形的初始位置和大小 setPos(0, 0); m_width = 100; m_height = 100; } QRectF boundingRect() const override { return QRectF(0, 0, m_width, m_height); } void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) override { // 绘制图形 painter->drawRect(boundingRect()); } void mouseMoveEvent(QGraphicsSceneMouseEvent *event) override { // 获取当前鼠标位置和上一次鼠标位置之间的差值 QPointF delta = event->pos() - event->lastPos(); // 只允许沿着 X 轴方向移动图形 QPointF newPos = pos() + QPointF(delta.x(), 0); // 限制图形的移动范围 if (newPos.x() < 0) newPos.setX(0); if (newPos.x() + m_width > scene()->width()) newPos.setX(scene()->width() - m_width); // 更新图形的位置 setPos(newPos); } private: qreal m_width; qreal m_height; };这段代码图形不能沿x轴负方向移动
时间: 2024-02-14 13:14:56 浏览: 64
这段代码中的 `mouseMoveEvent` 函数实现了鼠标拖拽移动图形的功能,并且只允许沿着 X 轴正方向移动图形。如果想要允许沿着 X 轴负方向移动图形,可以将代码中的以下部分:
```
if (newPos.x() < 0)
newPos.setX(0);
```
改为:
```
if (newPos.x() < scene()->width() - m_width)
newPos.setX(scene()->width() - m_width);
```
这样就可以实现沿着 X 轴负方向移动图形的功能了。同时,`boundingRect` 函数实现了图形的边界框,可以根据实际需求进行修改。
相关问题
error: 'MyItem::MyItem(QGraphicsItem*)' is private within this context MyItem* item = new MyItem; ^~~~~~
这个错误通常是因为在程序中试图访问MyItem类的私有构造函数。私有构造函数是类中特殊的成员函数,只能在类内部被访问,不能被类的外部访问。在你的代码中,你试图使用一个不带参数的构造函数创建MyItem类的对象。然而,你的MyItem类定义了一个私有构造函数,这个构造函数只能在类内部被访问,因此编译器无法创建这个对象。
为了解决这个问题,你需要确保在类定义中提供一个公共的构造函数,以便在类外部创建对象。例如:
```
class MyItem : public QGraphicsItem {
public:
MyItem(QGraphicsItem* parent = nullptr) : QGraphicsItem(parent) {
// 构造函数的实现
}
// 其他成员函数和成员变量的定义
};
```
在这个例子中,我们提供了一个公共的构造函数,它接受一个QGraphicsItem类型的参数,并将其传递给基类的构造函数。这个公共构造函数可以在类的外部被访问,以便创建MyItem类的对象。
#include <QtWidgets>class MyItem : public QGraphicsObject{public: MyItem(int pinCount, QColor color, QSizeF size, QGraphicsItem* parent = nullptr) : QGraphicsObject(parent) , m_pinCount(pinCount) , m_color(color) , m_size(size) {} QRectF boundingRect() const override { return QRectF(QPointF(0, 0), m_size); } QPainterPath shape() const override { QPainterPath path; path.addRect(QRectF(QPointF(0, 0), m_size)); return path; } void paint(QPainter* painter, const QStyleOptionGraphicsItem* option, QWidget* widget) override { Q_UNUSED(option) Q_UNUSED(widget) painter->setPen(Qt::NoPen); painter->setBrush(m_color); painter->drawRect(boundingRect()); painter->setPen(Qt::black); painter->setFont(QFont("Arial", 10)); painter->drawText(boundingRect(), Qt::AlignCenter, QString("%1 pins").arg(m_pinCount)); } void mousePressEvent(QGraphicsSceneMouseEvent* event) override { if (event->button() == Qt::LeftButton) { setCursor(Qt::ClosedHandCursor); m_lastPos = event->scenePos(); } } void mouseMoveEvent(QGraphicsSceneMouseEvent* event) override { if (QLineF(event->screenPos(), m_lastPos).length() < QApplication::startDragDistance()) return; QGraphicsObject::mouseMoveEvent(event); setPos(pos() + event->scenePos() - m_lastPos); m_lastPos = event->scenePos(); } void mouseReleaseEvent(QGraphicsSceneMouseEvent* event) override { if (event->button() == Qt::LeftButton) { setCursor(Qt::ArrowCursor); } }private: int m_pinCount; QColor m_color; QSizeF m_size; QPointF m_lastPos;};int main(int argc, char* argv[]){ QApplication app(argc, argv); QGraphicsScene scene; scene.setSceneRect(0, 0, 800, 600); MyItem* item1 = new MyItem(8, Qt::red, QSizeF(80, 60)); item1->setPos(100, 100); scene.addItem(item1); MyItem* item2 = new MyItem(16, Qt::green, QSizeF(120, 80)); item2->setPos(300, 200); scene.addItem(item2); QGraphicsView view(&scene); view.setRenderHint(QPainter::Antialiasing); view.setDragMode(QGraphicsView::RubberBandDrag); view.setViewportUpdateMode(QGraphicsView::FullViewportUpdate); view.show(); return app.exec();}在这个代码中,如何使用TextOut去给矩形框中添加文本
这个代码是使用Qt的绘图框架来实现的,所以不能直接使用TextOut函数。可以在MyItem类的paint函数中使用QPainter的函数来绘制文本,如下所示:
```
void paint(QPainter* painter, const QStyleOptionGraphicsItem* option, QWidget* widget) override
{
Q_UNUSED(option)
Q_UNUSED(widget)
painter->setPen(Qt::NoPen);
painter->setBrush(m_color);
painter->drawRect(boundingRect());
painter->setPen(Qt::black);
painter->setFont(QFont("Arial", 10));
painter->drawText(boundingRect(), Qt::AlignCenter, QString("%1 pins").arg(m_pinCount));
}
```
这段代码中,我们使用了QPainter的setFont函数来设置字体,使用drawText函数来绘制文本。其中,boundingRect()函数返回了该图形项的边界矩形,Qt::AlignCenter表示文本将会居中对齐。这样,每个矩形框中就会显示相应的文本。
阅读全文
相关推荐
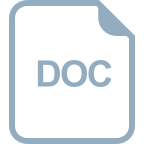
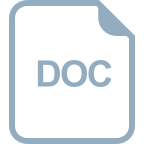
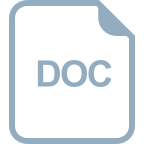













