解释代码 #include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <sys/wait.h> main() { pid_t pidA, pidB; int status; pidA = fork(); if (pidA < 0) { perror("fork error"); exit(1); } else if (pidA == 0) { // 子 A if (execl("/bin/ls", "ls", "-l", NULL) == -1) { perror("execl"); exit(1); } exit(0); } else { pidB = fork(); if (pidB < 0) { perror("fork error"); exit(1); } else if (pidB == 0) { // 子 B if (execl("/bin/ps", "ps", "-a", NULL) == -1) { perror("execl"); exit(1); } exit(0); } else { // 父 wait(&status); // 等待子 A结束 printf("Son A exited with status %d\n", WEXITSTATUS(status)); wait(&status); // 等待子 B结束 printf("Son B exited with status %d\n", WEXITSTATUS(status)); } } }
时间: 2024-02-15 10:28:26 浏览: 26
这段代码是一个使用 fork 和 exec 函数创建进程的示例。首先创建了两个子进程 pidA 和 pidB,然后在子进程中分别调用 execl 函数执行 /bin/ls 和 /bin/ps 命令。最后在父进程中使用 wait 函数等待子进程执行完毕,然后输出子进程的退出状态。其中,WEXITSTATUS(status) 函数可以获取子进程的退出状态。整个程序的功能是在终端上执行 ls -l 和 ps -a 命令。
相关问题
/* Socket head file */ #include<sys/types.h> #include<sys/socket.h> #include<netinet/in.h>/*sockaddr_in{}*/ #include<arpa/inet.h> /* Unix standard head file */ #include<stdio.h> #include<stdlib.h> #include<string.h> #include<unistd.h> #include <errno.h>/* strerror(),perror(),errno head file*/ #include <sys/time.h> #include <sys/wait.h> #define MAXBUFF 1024 #define PORT 9999怎么改成windows系统
在Windows系统中,网络编程需要使用Winsock API,因此需要引入`<winsock2.h>`头文件。此外,需要在程序中调用`WSAStartup()`函数和`WSACleanup()`函数来初始化和释放Winsock库。
下面是在Windows系统下进行网络编程的头文件和常量定义示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <winsock2.h>
#include <ws2tcpip.h>
#pragma comment(lib, "Ws2_32.lib")
#define MAXBUFF 1024
#define PORT 9999
```
其中,`<ws2tcpip.h>`头文件包含了一些网络编程相关的函数和结构体定义。`#pragma comment(lib, "Ws2_32.lib")`语句用于链接Winsock库。
需要注意的是,在Windows系统中,端口号的类型为`unsigned short`,而不是`int`。因此,定义端口号常量时应该使用`USHORT`类型:
```c
#define PORT (USHORT)9999
```
include <unistd.h> #include <stdio.h> #include <stdlib.h> #include <sys/types.h> #include <sys/wait.h> int main(void){ pid_t a; a = fork(); if (a < 0) { perror("fork failed"); exit(1); } if (a == 0) { execlp ("ps" ,"ps",NULL); execlp ("ls" ,"ls","-al","/etc/passwd ",NULL); return 1; } else { wait(NULL); printf("father exit\n");} return 0; }改正以上代码
根据你提供的代码,我们可以看出,子进程中有两个 `execlp` 函数调用,这意味着子进程将执行两个程序,而不是一个程序。此外,在第二个 `execlp` 函数调用中,你在 `/etc/passwd` 后面添加了一个空格,这可能会导致程序无法正常运行。
下面是改正后的代码:
```c
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/wait.h>
int main(void) {
pid_t pid;
pid = fork();
if (pid < 0) {
perror("fork failed");
exit(1);
} else if (pid == 0) {
execlp("ps", "ps", NULL);
perror("execlp failed");
exit(1);
} else {
wait(NULL);
printf("child process finished\n");
}
return 0;
}
```
在上面的代码中,我们只调用了一个 `execlp` 函数,并且没有在 `/etc/passwd` 后面添加空格。如果 `execlp` 调用失败,我们使用 `perror` 函数输出错误信息。此外,我们还将 `printf` 语句修改为 "child process finished",以更清晰地表达程序的意图。
相关推荐
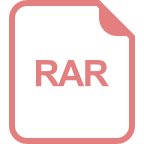
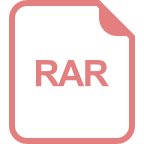
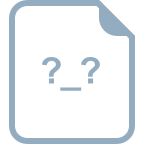













