#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <sys/wait.h> int main( ) { int fd[2]; char buf[30], s[30]; pipe(fd); //创建管道 while((fork()) == -1); //创建子程序失败时,循环 if(fork() == 0) //子进程返回 { sprintf(buf, "This is an example\n"); //将 buf 中字符写入管道 write(fd[1], buf, sizeof(buf)); //将 buf 中字符写入管道 } else //父进程返回 { wait(0); //等待子程序结束 read(fd[0], s, sizeof(s)); //父进程读管道中字符 printf("%s", s); //输出读取到的字符 } return 0; }改良以上程序
时间: 2024-03-25 11:38:03 浏览: 20
以上程序是一个简单的管道通信例子,可以通过管道将一个字符串从子进程传递给父进程并输出。以下是对程序的改良:
1. 错误处理:以上程序没有进行错误处理,可以在创建管道和创建子进程时添加错误处理语句。
2. 参数传递:以上程序只传递了一个字符串,可以通过参数传递实现更加灵活的通信。
3. 程序退出:以上程序没有使用 exit() 函数退出程序,可以添加退出语句以保证程序正常结束。
改良后的程序如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main(int argc, char *argv[]) {
int fd[2];
char buf[30], s[30];
if (pipe(fd) == -1) { //创建管道失败
perror("pipe");
exit(EXIT_FAILURE);
}
pid_t pid = fork();
if (pid == -1) { //创建子进程失败
perror("fork");
exit(EXIT_FAILURE);
}
else if (pid == 0) { //子进程返回
if (close(fd[0]) == -1) { //关闭管道读端
perror("close");
exit(EXIT_FAILURE);
}
sprintf(buf, "%s\n", argv[1]); //将参数写入管道
if (write(fd[1], buf, sizeof(buf)) == -1) { //将 buf 中字符写入管道
perror("write");
exit(EXIT_FAILURE);
}
if (close(fd[1]) == -1) { //关闭管道写端
perror("close");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
else { //父进程返回
if (close(fd[1]) == -1) { //关闭管道写端
perror("close");
exit(EXIT_FAILURE);
}
if (read(fd[0], s, sizeof(s)) == -1) { //父进程读管道中字符
perror("read");
exit(EXIT_FAILURE);
}
printf("%s", s); //输出读取到的字符
if (close(fd[0]) == -1) { //关闭管道读端
perror("close");
exit(EXIT_FAILURE);
}
wait(NULL); //等待子程序结束
exit(EXIT_SUCCESS);
}
return 0;
}
```
改良后的程序增加了错误处理和参数传递功能,程序退出时使用了 exit() 函数。
相关推荐
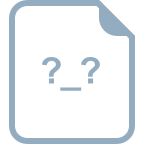
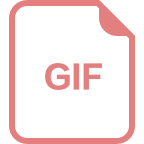
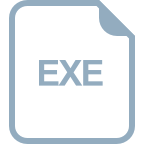















