openGL+open3d+python 鼠标拖动画矩形框选出点云数据的感兴趣区域代码
时间: 2023-07-14 15:12:25 浏览: 85
以下是一个简单的示例代码,演示如何使用OpenGL和Open3D库在Python中拖动矩形框选出点云数据的感兴趣区域:
```python
import numpy as np
import open3d as o3d
from OpenGL.GL import *
from OpenGL.GLU import *
from PyQt5.QtCore import *
from PyQt5.QtGui import *
from PyQt5.QtOpenGL import *
class GLWidget(QGLWidget):
def __init__(self, parent=None):
super(GLWidget, self).__init__(parent)
self.width = 640
self.height = 480
self.x0, self.y0 = 0, 0
self.x1, self.y1 = 0, 0
self.points = None
def initializeGL(self):
glClearColor(1.0, 1.0, 1.0, 1.0)
glEnable(GL_DEPTH_TEST)
def resizeGL(self, w, h):
glViewport(0, 0, w, h)
glMatrixMode(GL_PROJECTION)
glLoadIdentity()
gluPerspective(45.0, float(w)/float(h), 0.1, 100.0)
glMatrixMode(GL_MODELVIEW)
def paintGL(self):
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
glLoadIdentity()
gluLookAt(0, 0, 5, 0, 0, 0, 0, 1, 0)
glPointSize(5)
glColor3f(1.0, 0.0, 0.0)
glBegin(GL_POINTS)
if self.points is not None:
for p in self.points:
glVertex3f(p[0], p[1], p[2])
glEnd()
glColor3f(0.0, 0.0, 1.0)
glLineWidth(2)
glBegin(GL_LINE_LOOP)
glVertex2f(self.x0, self.y0)
glVertex2f(self.x1, self.y0)
glVertex2f(self.x1, self.y1)
glVertex2f(self.x0, self.y1)
glEnd()
def mousePressEvent(self, event):
self.x0, self.y0 = event.x(), event.y()
self.x1, self.y1 = event.x(), event.y()
def mouseMoveEvent(self, event):
self.x1, self.y1 = event.x(), event.y()
self.update()
def mouseReleaseEvent(self, event):
self.x1, self.y1 = event.x(), event.y()
self.update()
# 获取矩形框选范围内的点云数据
w, h = abs(self.x1 - self.x0), abs(self.y1 - self.y0)
x, y = min(self.x0, self.x1), min(self.y0, self.y1)
data = glReadPixels(x, self.height-y-h, w, h, GL_RGB, GL_UNSIGNED_BYTE)
image = QImage(data, w, h, QImage.Format_RGB888)
image = image.mirrored()
pcd = o3d.geometry.PointCloud.create_from_qt_image(image, 100.0, self.width, self.height)
# 显示矩形框选范围内的点云数据
self.points = np.asarray(pcd.points)
self.update()
if __name__ == '__main__':
app = QApplication([])
widget = GLWidget()
widget.setMinimumSize(640, 480)
widget.show()
app.exec_()
```
在这个示例中,我们使用了PyQt5库和OpenGL来创建一个Qt窗口,并在窗口中展示点云数据。我们可以用鼠标拖动矩形框选出感兴趣区域,并获取该区域内的点云数据。具体实现过程如下:
1. 在`initializeGL`函数中,我们设置了OpenGL的清除颜色和深度缓冲,并启用了深度测试。
2. 在`resizeGL`函数中,我们设置了OpenGL的视口和投影矩阵,以便在窗口大小变化时正确显示点云数据。
3. 在`paintGL`函数中,我们首先清除颜色和深度缓冲,并设置视图矩阵。然后,我们使用红色点绘制点云数据,并使用蓝色线绘制矩形框选范围。
4. 在`mousePressEvent`、`mouseMoveEvent`和`mouseReleaseEvent`函数中,我们响应鼠标事件,并更新矩形框选范围。
5. 在`mouseReleaseEvent`函数中,我们通过OpenGL的`glReadPixels`函数获取矩形框选范围内的像素数据,并将其转换为Qt中的QImage对象。然后,我们使用Open3D库提供的`create_from_qt_image`函数将QImage对象转换为点云数据,并将其显示在窗口中。
这个示例仅为一个简单的演示,具体实现方式可能因应用场景和需要而有所不同。
相关推荐
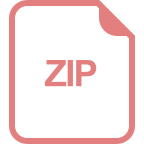













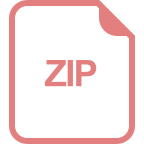
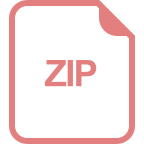