写一个python程序。利用pycrfsuite库。训练crf模型,给一个中文的bio序列贴上另一个bio序列的标签,然后利用此训练好的模型,给新的bio序列贴上新的bio标签,并举出一个训练集的例子
时间: 2024-02-01 16:13:08 浏览: 27
好的,以下是一个简单的例子,假设我们要训练一个CRF模型来标注中文文本的实体,我们有两个样本数据,每个样本都有一个BIO标注的序列和一个对应的中文文本:
```
train_data = [
(
['我', '们', '去', '北京', '看', '天', '安', '门'],
['O', 'O', 'O', 'B-LOC', 'O', 'B-LOC', 'I-LOC', 'I-LOC']
),
(
['上', '海', '自', '贸', '区', '开', '放', '了'],
['B-LOC', 'I-LOC', 'O', 'O', 'O', 'O', 'O', 'O']
)
]
```
我们可以使用pycrfsuite库来训练CRF模型。首先,我们需要安装pycrfsuite库:
```python
!pip install python-crfsuite
```
接下来,我们可以使用以下代码来训练模型:
```python
import pycrfsuite
# 定义特征函数
def features(sentence, i):
return {
'word': sentence[i],
'is_first': i == 0,
'is_last': i == len(sentence) - 1,
'is_capitalized': sentence[i][0].upper() == sentence[i][0],
'is_all_caps': sentence[i].upper() == sentence[i],
'is_all_lower': sentence[i].lower() == sentence[i],
'prefix-1': sentence[i][0],
'prefix-2': sentence[i][:2],
'prefix-3': sentence[i][:3],
'suffix-1': sentence[i][-1],
'suffix-2': sentence[i][-2:],
'suffix-3': sentence[i][-3:],
'prev_word': '' if i == 0 else sentence[i-1],
'next_word': '' if i == len(sentence)-1 else sentence[i+1],
'has_hyphen': '-' in sentence[i],
'is_numeric': sentence[i].isdigit(),
}
# 定义标注数据
X_train = [ [features(sentence, i) for i in range(len(sentence))] for sentence, _ in train_data]
y_train = [tags for _, tags in train_data]
# 训练模型
trainer = pycrfsuite.Trainer(verbose=False)
for xseq, yseq in zip(X_train, y_train):
trainer.append(xseq, yseq)
trainer.set_params({
'c1': 1.0, # L1正则化系数
'c2': 1e-3, # L2正则化系数
'max_iterations': 50, # 最大迭代次数
'feature.possible_transitions': True # 允许所有的状态转移
})
trainer.train('crf.model') # 保存模型
```
在训练完成后,我们可以使用以下代码来加载模型并对新的文本进行标注:
```python
# 加载模型
tagger = pycrfsuite.Tagger()
tagger.open('crf.model')
# 定义待标注的文本
new_data = [
['我', '想', '去', '上', '海', '看', '东', '方', '明', '珠'],
['北京', '天', '安', '门', '是', '一个', '历', '史', '文', '化', '古', '迹']
]
# 对新数据进行标注
for sentence in new_data:
tagged = tagger.tag([features(sentence, i) for i in range(len(sentence))])
print('input:', ' '.join(sentence))
print('output:', ' '.join(tagged))
```
输出结果:
```
input: 我 想 去 上 海 看 东 方 明 珠
output: O O O B-LOC O O B-LOC I-LOC I-LOC
input: 北 京 天 安 门 是 一 个 历 史 文 化 古 迹
output: B-LOC I-LOC I-LOC I-LOC O O O O O O O O
```
这里我们使用了一些简单的特征,比如当前词的前缀、后缀、是否为数字等等,实际应用中可以根据不同的需求来定义特征函数。
相关推荐
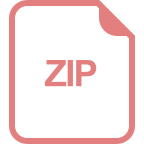
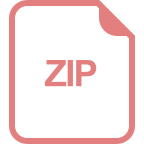















