给我一段代码 pygame 用set timer来控制俄罗斯方块的速度
时间: 2023-05-13 14:04:52 浏览: 54
下面是一段使用 Pygame 的 set_timer 函数来控制俄罗斯方块速度的示例代码:
```python
import pygame
import random
# 游戏窗口大小
WINDOW_WIDTH = 640
WINDOW_HEIGHT = 480
# 方块大小
BLOCK_SIZE = 20
# 方块类型及其形状
BLOCK_TYPES = [
[[1, 1, 1, 1]],
[[1, 1], [1, 1]],
[[1, 0, 0], [1, 1, 1]],
[[0, 0, 1], [1, 1, 1]],
[[1, 1, 0], [0, 1, 1]],
[[0, 1, 1], [1, 1, 0]],
[[1, 1, 1], [0, 1, 0]],
]
# 方块颜色
BLOCK_COLORS = [
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255),
(255, 255, 255),
]
# 方块下落速度
BLOCK_SPEED = 500
# 初始化 Pygame
pygame.init()
# 创建游戏窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
# 设置游戏窗口标题
pygame.display.set_caption('Tetris')
# 设置定时器事件
pygame.time.set_timer(pygame.USEREVENT, BLOCK_SPEED)
# 生成一个新的方块
def new_block():
block_type = random.randint(0, len(BLOCK_TYPES) - 1)
block_color = BLOCK_COLORS[block_type]
block_shape = BLOCK_TYPES[block_type]
block_x = (WINDOW_WIDTH - len(block_shape[0]) * BLOCK_SIZE) // 2
block_y = 0
return {
'type': block_type,
'color': block_color,
'shape': block_shape,
'x': block_x,
'y': block_y,
}
# 绘制方块
def draw_block(block):
for i in range(len(block['shape'])):
for j in range(len(block['shape'][i])):
if block['shape'][i][j] == 1:
x = block['x'] + j * BLOCK_SIZE
y = block['y'] + i * BLOCK_SIZE
pygame.draw.rect(window, block['color'], (x, y, BLOCK_SIZE, BLOCK_SIZE))
# 主循环
def main_loop():
# 生成第一个方块
current_block = new_block()
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
return
elif event.type == pygame.USEREVENT:
current_block['y'] += BLOCK_SIZE
if current_block['y'] + len(current_block['shape']) * BLOCK_SIZE > WINDOW_HEIGHT:
current_block = new_block()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
current_block['x'] -= BLOCK_SIZE
elif event.key == pygame.K_RIGHT:
current_block['x'] += BLOCK_SIZE
elif event.key == pygame.K_DOWN:
current_block['y'] += BLOCK_SIZE
# 绘制背景
window.fill((0, 0, 0))
# 绘制当前方块
draw_block(current_block)
# 更新屏幕
pygame.display.update()
# 运行游戏
main_loop()
```
这段代码使用 Pygame 实现了俄罗斯方块游戏,并使用 set_timer 函数来控制方块的下落速度。具体来说,它在主循环中设置了一个 USEREVENT 定时器事件,并将其时间间隔设置为 BLOCK_SPEED,即方块下落的速度。每当定时器事件触发时,程序就将当前方块的 y 坐标加上一个方块大小,使其向下移动一个方块的距离。如果当前方块已经到达窗口底部,则生成一个新的方块。
相关推荐
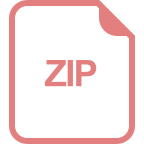
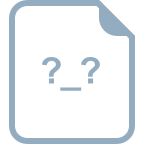











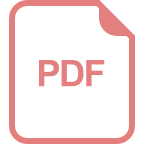
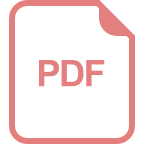
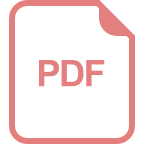