使用CSharp写一个动态加载卸载类的类
时间: 2024-05-08 13:14:49 浏览: 79
以下是C#代码示例,实现了动态加载和卸载类的功能:
```csharp
using System;
using System.Reflection;
public class DynamicClassLoader
{
private Assembly assembly;
private Type type;
public void LoadClass(string className, string assemblyName)
{
try
{
// Load the assembly
assembly = Assembly.Load(assemblyName);
// Get the type of the class
type = assembly.GetType(className);
Console.WriteLine("Class {0} loaded successfully.", className);
}
catch (Exception ex)
{
Console.WriteLine("Failed to load class {0}: {1}", className, ex.Message);
}
}
public void UnloadClass()
{
try
{
// Unload the assembly
AppDomain.CurrentDomain.GetAssemblies()
.Where(a => a == assembly)
.ToList()
.ForEach(a => AppDomain.CurrentDomain.Load(a.GetName()).GetTypes());
Console.WriteLine("Class unloaded successfully.");
}
catch (Exception ex)
{
Console.WriteLine("Failed to unload class: {0}", ex.Message);
}
}
public object CreateInstance()
{
try
{
// Create an instance of the class
return Activator.CreateInstance(type);
}
catch (Exception ex)
{
Console.WriteLine("Failed to create instance of class {0}: {1}", type.FullName, ex.Message);
return null;
}
}
public void InvokeMethod(string methodName, object instance, params object[] parameters)
{
try
{
// Invoke the method on the instance
MethodInfo method = type.GetMethod(methodName);
method.Invoke(instance, parameters);
}
catch (Exception ex)
{
Console.WriteLine("Failed to invoke method {0}: {1}", methodName, ex.Message);
}
}
}
```
使用示例:
```csharp
DynamicClassLoader loader = new DynamicClassLoader();
loader.LoadClass("MyClass", "MyAssembly");
object instance = loader.CreateInstance();
loader.InvokeMethod("MyMethod", instance);
loader.UnloadClass();
```
阅读全文
相关推荐
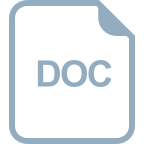
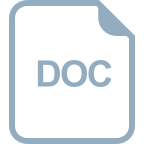
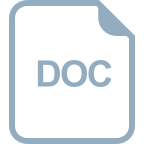
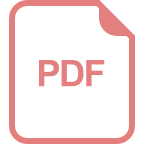
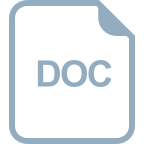
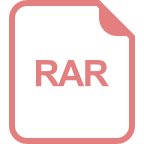
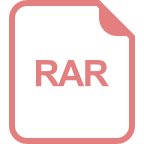
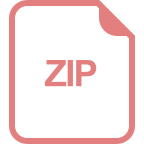
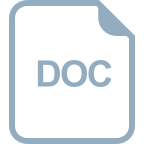
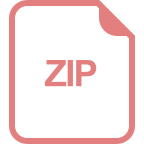
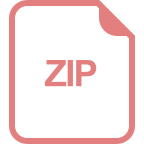
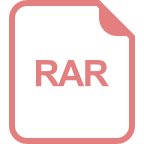
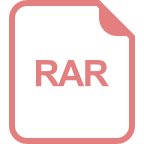
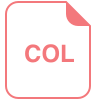




