Java方法实现求矩形 三角形 圆形面积系统
时间: 2024-02-24 15:58:16 浏览: 187
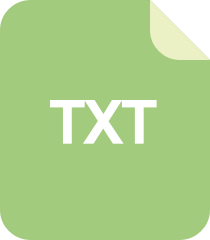
java程序代码实现矩形、三角形、圆的面积和周长计算

可以使用Java的面向对象编程思想,将矩形、三角形、圆形抽象成类,并在类中定义求面积的方法。
以矩形为例,可以定义一个矩形类(Rectangle),其中包含矩形的长和宽属性,以及求矩形面积的方法(getArea()):
```
public class Rectangle {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double getArea() {
return length * width;
}
}
```
同样的,可以定义一个三角形类(Triangle),其中包含三角形的底边和高属性,以及求三角形面积的方法(getArea()):
```
public class Triangle {
private double base;
private double height;
public Triangle(double base, double height) {
this.base = base;
this.height = height;
}
public double getArea() {
return base * height / 2;
}
}
```
还可以定义一个圆形类(Circle),其中包含圆形的半径属性,以及求圆形面积的方法(getArea()):
```
public class Circle {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
}
```
在主程序中,可以根据用户的输入创建不同的矩形、三角形、圆形对象,并调用它们的getArea()方法求面积:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请选择要计算的图形:");
System.out.println("1. 矩形");
System.out.println("2. 三角形");
System.out.println("3. 圆形");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.print("请输入矩形的长和宽:");
double length = scanner.nextDouble();
double width = scanner.nextDouble();
Rectangle rectangle = new Rectangle(length, width);
System.out.println("矩形的面积为:" + rectangle.getArea());
break;
case 2:
System.out.print("请输入三角形的底边和高:");
double base = scanner.nextDouble();
double height = scanner.nextDouble();
Triangle triangle = new Triangle(base, height);
System.out.println("三角形的面积为:" + triangle.getArea());
break;
case 3:
System.out.print("请输入圆形的半径:");
double radius = scanner.nextDouble();
Circle circle = new Circle(radius);
System.out.println("圆形的面积为:" + circle.getArea());
break;
default:
System.out.println("输入有误!");
break;
}
}
}
```
这样就可以实现一个简单的求矩形、三角形、圆形面积的系统了。
阅读全文
相关推荐
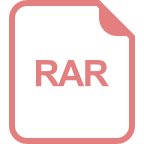




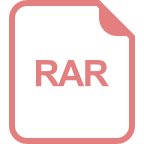








