fit_plane_3d_ransac具体的函数代码及函数编写思路
时间: 2024-02-12 20:04:29 浏览: 126
fit_plane_3d_ransac函数是一个基于RANSAC算法来拟合3D平面的函数,其具体的函数代码及函数编写思路如下:
函数代码:
```python
def fit_plane_3d_ransac(points, max_iterations=100, distance_threshold=0.01):
"""
:param points: List of 3D points in the format [(x1, y1, z1), (x2, y2, z2), ...]
:param max_iterations: Maximum number of iterations to perform
:param distance_threshold: Distance threshold to determine inliers
:return: A tuple of the form (a, b, c, d), representing the plane equation ax + by + cz + d = 0
"""
best_inliers = []
best_plane = None
for i in range(max_iterations):
# Choose 3 random points
random_points = random.sample(points, 3)
# Compute the plane equation for these 3 points
plane = compute_plane_equation(random_points)
# Compute the distance between all points and the plane
distances = [compute_distance_to_plane(point, plane) for point in points]
# Determine the inliers based on the distance threshold
inliers = [point for j, point in enumerate(points) if distances[j] < distance_threshold]
# If the number of inliers is greater than the current best, update the best plane and inliers
if len(inliers) > len(best_inliers):
best_inliers = inliers
best_plane = compute_plane_equation(best_inliers)
return best_plane
```
函数编写思路:
1. 首先定义一个函数fit_plane_3d_ransac,该函数接收一个包含3D点的列表,最大迭代次数和距离阈值作为输入参数。
2. 在函数中,定义一个变量best_inliers,用于存储当前拟合的平面中的内点,同时定义一个变量best_plane,用于存储当前最佳平面的方程。
3. 在循环中,执行max_iterations次以下操作:
- 随机选择三个点。
- 计算这三个点所在的平面方程。
- 计算所有点到该平面的距离。
- 根据距离阈值确定内点。
- 如果内点数目大于当前最佳平面的内点数目,则更新最佳平面和内点。
4. 返回最佳平面的方程。
总的来说,该函数采用随机抽样一致性算法(RANSAC)来拟合3D平面,可以在存在噪声和离群值的情况下得到可靠的结果。
阅读全文
相关推荐


















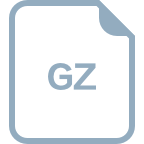