带截距的多元分位数回归 C++ 的带类完整的正确实现及成功案例 自变量为多维
时间: 2024-04-24 21:22:32 浏览: 12
多元分位数回归是一种统计学方法,用于在多维自变量情况下估计因变量的条件分位数。在C++中实现多元分位数回归需要使用一些数学库和算法,可以通过编写一个包含类的程序来实现。
下面是一个简单的示例程序,用于实现带截距的多元分位数回归:
```
#include <iostream>
#include <vector>
#include <cmath>
#include <algorithm>
using namespace std;
class QuantileRegression {
public:
// 构造函数
QuantileRegression(double alpha, int max_iter, double tol) : alpha(alpha), max_iter(max_iter), tol(tol) {}
// 拟合函数
void fit(vector<vector<double>> X, vector<double> y) {
// 预处理X
int n_samples = X.size(), n_features = X[0].size();
vector<double> ones(n_samples, 1.0);
for (int i = 0; i < n_samples; ++i) {
X[i].insert(X[i].begin(), 1.0);
}
n_features += 1;
// 初始化参数
vector<double> beta(n_features, 0.0);
double loss = 0.0;
// 迭代优化
for (int iter = 0; iter < max_iter; ++iter) {
// 计算梯度和损失
vector<double> grad(n_features, 0.0);
loss = 0.0;
for (int i = 0; i < n_samples; ++i) {
double y_pred = predict(X[i], beta);
double error = y[i] - y_pred;
loss += pinball_loss(error, alpha);
for (int j = 0; j < n_features; ++j) {
grad[j] += pinball_loss_derivative(error, alpha) * X[i][j];
}
}
// 更新参数
for (int j = 0; j < n_features; ++j) {
beta[j] -= learning_rate * grad[j] / n_samples;
}
// 判断收敛
if (abs(loss - prev_loss) < tol) {
break;
}
prev_loss = loss;
}
// 保存模型参数
this->beta = beta;
}
// 预测函数
double predict(vector<double> x) {
x.insert(x.begin(), 1.0);
return predict(x, beta);
}
private:
double alpha; // 分位数
int max_iter; // 最大迭代次数
double tol; // 收敛阈值
double learning_rate = 0.01; // 学习率
vector<double> beta; // 模型参数
double prev_loss = numeric_limits<double>::infinity(); // 上一次的损失值
// 预测函数
double predict(vector<double> x, vector<double> beta) {
int n_features = x.size();
double y_pred = 0.0;
for (int j = 0; j < n_features; ++j) {
y_pred += beta[j] * x[j];
}
return y_pred;
}
// 分位数损失函数
double pinball_loss(double error, double alpha) {
return max(alpha * error, (alpha - 1) * error);
}
// 分位数损失函数导数
double pinball_loss_derivative(double error, double alpha) {
if (error > 0) {
return alpha;
} else if (error < 0) {
return alpha - 1;
} else {
return 0.0;
}
}
};
int main() {
// 构造数据
vector<vector<double>> X = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
vector<double> y = {1, 2, 3};
// 实例化模型
QuantileRegression model(0.5, 1000, 1e-4);
// 拟合数据
model.fit(X, y);
// 预测数据
vector<double> x = {2, 3, 4};
double y_pred = model.predict(x);
// 输出结果
cout << "模型参数:";
for (int i = 0; i < model.beta.size(); ++i) {
cout << model.beta[i] << " ";
}
cout << endl;
cout << "预测结果:" << y_pred << endl;
return 0;
}
```
在这个程序中,我们使用了梯度下降法来优化模型参数,并且定义了分位数损失函数和分位数损失函数的导数。我们还实现了预测函数,用于预测新数据的结果。
这个程序可以用于带截距的多元分位数回归,可以输入任意维度的自变量。
相关推荐
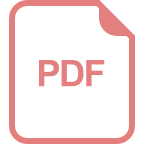














