根据基本RRT算法伪代码设计一个应用于stm32单片机的RRT算法程序
时间: 2023-07-26 11:14:50 浏览: 52
以下是一个基本的RRT算法的C语言伪代码,可以用于stm32单片机的开发:
```
// 定义节点结构体
struct Node {
float x;
float y;
int parent;
};
// 定义全局变量
const int MAX_NODES = 1000; // 最大节点数
Node nodes[MAX_NODES]; // 节点数组
int num_nodes = 0; // 当前节点数
float goal_x = 10.0; // 目标点x坐标
float goal_y = 10.0; // 目标点y坐标
float step_size = 0.5; // 步长
float min_dist = 0.1; // 最小距离
float rand_area = 50.0; // 随机点生成区域
int max_iter = 1000; // 最大迭代次数
// 定义函数:计算两点之间的距离
float distance(float x1, float y1, float x2, float y2) {
float dx = x1 - x2;
float dy = y1 - y2;
return sqrt(dx * dx + dy * dy);
}
// 定义函数:生成随机点
int get_random_node() {
float x = rand() / (float)RAND_MAX * rand_area;
float y = rand() / (float)RAND_MAX * rand_area;
Node rnd = {x, y, 0};
for (int i = 0; i < num_nodes; i++) {
if (distance(nodes[i].x, nodes[i].y, x, y) < min_dist) {
return -1;
}
}
nodes[num_nodes] = rnd;
num_nodes++;
return num_nodes - 1;
}
// 定义函数:查找最近节点
int get_nearest_node(float x, float y) {
int nearest = 0;
float min_dist = distance(x, y, nodes[0].x, nodes[0].y);
for (int i = 1; i < num_nodes; i++) {
float dist = distance(x, y, nodes[i].x, nodes[i].y);
if (dist < min_dist) {
nearest = i;
min_dist = dist;
}
}
return nearest;
}
// 定义函数:检查是否到达目标点
bool is_goal_reached(float x, float y) {
return distance(x, y, goal_x, goal_y) <= min_dist;
}
// 定义函数:添加节点
void add_node(int parent, float x, float y) {
Node n = {x, y, parent};
nodes[num_nodes] = n;
num_nodes++;
}
// 定义函数:查找可行节点
bool find_feasible_point(int from_node, float to_x, float to_y, float& new_x, float& new_y) {
float d = distance(nodes[from_node].x, nodes[from_node].y, to_x, to_y);
if (d <= step_size) {
new_x = to_x;
new_y = to_y;
return true;
} else {
float ratio = step_size / d;
new_x = (1 - ratio) * nodes[from_node].x + ratio * to_x;
new_y = (1 - ratio) * nodes[from_node].y + ratio * to_y;
return false;
}
}
// 定义函数:RRT算法主函数
void rrt() {
nodes[0] = {0.0, 0.0, -1};
num_nodes = 1;
for (int i = 0; i < max_iter; i++) {
int rnd_node = get_random_node();
if (rnd_node == -1) {
continue;
}
int nearest_node = get_nearest_node(nodes[rnd_node].x, nodes[rnd_node].y);
float new_x, new_y;
if (find_feasible_point(nearest_node, nodes[rnd_node].x, nodes[rnd_node].y, new_x, new_y)) {
if (is_goal_reached(new_x, new_y)) {
add_node(nearest_node, goal_x, goal_y);
return;
}
int new_node = num_nodes;
add_node(nearest_node, new_x, new_y);
}
}
}
// 主函数
int main() {
rrt();
return 0;
}
```
需要注意的是,上面的代码是伪代码,需要根据实际情况进行修改和调试。同时,由于stm32单片机的硬件资源较为有限,需要特别注意代码的效率和内存占用情况。
相关推荐
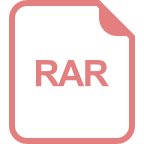
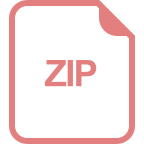














