生成一个matlab中三位路径规划的rrt算法代码
时间: 2024-04-25 10:06:21 浏览: 149
以下是一个简单的 MATLAB RRT 算法代码,用于三维路径规划:
```matlab
% RRT Path Planning Algorithm for 3D space
% Author: [your name and email]
clear all
close all
clc
% Define the start and goal positions
start = [0,0,0];
goal = [10,10,10];
% Define the boundary of the workspace
xmin = 0;
xmax = 20;
ymin = 0;
ymax = 20;
zmin = 0;
zmax = 20;
% Define the parameters of the RRT algorithm
max_iter = 1000; % maximum number of iterations
step_size = 1; % step size of each iteration
goal_tol = 0.1; % tolerance for reaching the goal
obstacles = [5,5,5,2; 15,15,15,2]; % define the obstacles in the workspace
% Initialize the tree and add the start node
tree = [start, 0];
plot3(start(1), start(2), start(3), 'go', 'MarkerSize', 10);
hold on;
for i = 1:max_iter
% Generate a random point in the workspace
rand_point = [randi([xmin,xmax]), randi([ymin,ymax]), randi([zmin,zmax])];
% Find the nearest node in the tree
distances = sqrt(sum((tree(:,1:3) - rand_point).^2, 2));
[min_dist, nearest_node] = min(distances);
nearest_point = tree(nearest_node, 1:3);
% Calculate the new point
new_point = nearest_point + step_size * (rand_point - nearest_point) / min_dist;
% Check if the new point is within the workspace boundaries
if new_point(1) < xmin || new_point(1) > xmax || new_point(2) < ymin || new_point(2) > ymax || new_point(3) < zmin || new_point(3) > zmax
continue;
end
% Check if the new point is in collision with any obstacles
in_collision = 0;
for j = 1:size(obstacles, 1)
if norm(new_point - obstacles(j,1:3)) < obstacles(j,4)
in_collision = 1;
break;
end
end
if in_collision
continue;
end
% Add the new point to the tree
new_node = [new_point, nearest_node];
tree = [tree; new_node];
plot3(new_point(1), new_point(2), new_point(3), 'ro', 'MarkerSize', 5);
hold on;
line([nearest_point(1), new_point(1)], [nearest_point(2), new_point(2)], [nearest_point(3), new_point(3)], 'Color', 'b', 'LineWidth', 1);
drawnow;
% Check if the goal is reached
if norm(new_point - goal) < goal_tol
goal_node = size(tree, 1);
break;
end
end
% Generate the path from the goal to the start
path = [goal_node];
current_node = goal_node;
while current_node ~= 1
current_node = tree(current_node, 4);
path = [current_node, path];
end
% Plot the final path
for i = 1:length(path)-1
start_point = tree(path(i), 1:3);
end_point = tree(path(i+1), 1:3);
line([start_point(1), end_point(1)], [start_point(2), end_point(2)], [start_point(3), end_point(3)], 'Color', 'r', 'LineWidth', 2);
end
```
该代码使用基本的 RRT 算法生成路径,其中包括随机采样、查找最近节点、计算新节点、检查碰撞等步骤。在算法结束时,它将生成从起点到终点的最优路径,并将其绘制出来。请注意,此代码仅用于学习和演示目的,并不适用于实际应用。
阅读全文
相关推荐
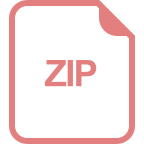
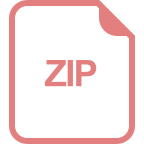















