用python写一个基于栅格地图的a*算法
时间: 2023-05-16 12:05:12 浏览: 190
好的,以下是一个基于栅格地图的A*算法的Python代码示例:
```python
import heapq
class Grid:
def __init__(self, width, height):
self.width = width
self.height = height
self.walls = set()
def in_bounds(self, pos):
x, y = pos
return 0 <= x < self.width and 0 <= y < self.height
def passable(self, pos):
return pos not in self.walls
def neighbors(self, pos):
x, y = pos
results = [(x+1, y), (x, y-1), (x-1, y), (x, y+1)]
if (x + y) % 2 == 0: results.reverse() # aesthetics
results = filter(self.in_bounds, results)
results = filter(self.passable, results)
return results
def cost(self, current, next):
return 1
def heuristic(a, b):
(x1, y1) = a
(x2, y2) = b
return abs(x1 - x2) + abs(y1 - y2)
def a_star_search(graph, start, goal):
frontier = PriorityQueue()
frontier.put(start, 0)
came_from = {}
cost_so_far = {}
came_from[start] = None
cost_so_far[start] = 0
while not frontier.empty():
current = frontier.get()
if current == goal:
break
for next in graph.neighbors(current):
new_cost = cost_so_far[current] + graph.cost(current, next)
if next not in cost_so_far or new_cost < cost_so_far[next]:
cost_so_far[next] = new_cost
priority = new_cost + heuristic(goal, next)
frontier.put(next, priority)
came_from[next] = current
return came_from, cost_so_far
class PriorityQueue:
def __init__(self):
self.elements = []
def empty(self):
return len(self.elements) == 0
def put(self, item, priority):
heapq.heappush(self.elements, (priority, item))
def get(self):
return heapq.heappop(self.elements)[1]
# Example usage:
# g = Grid(10, 10)
# g.walls = [(1, 1), (2, 1), (3, 1), (3, 2), (3, 3), (2, 3)]
# came_from, cost_so_far = a_star_search(g, (1, 2), (7, 8))
# print(came_from)
# print(cost_so_far)
```
这个代码示例实现了一个基于栅格地图的A*算法,可以用于寻找两个点之间的最短路径。你可以根据自己的需要修改代码来适应不同的场景。
相关推荐
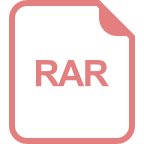














