用python实现一个基于栅格地图的astar
时间: 2023-05-16 16:04:48 浏览: 235
算法,你能给出代码示例吗?
当然可以,以下是一个基于栅格地图的A*算法的Python代码示例:
```python
import heapq
class Grid:
def __init__(self, width, height):
self.width = width
self.height = height
self.walls = set()
def in_bounds(self, pos):
x, y = pos
return 0 <= x < self.width and 0 <= y < self.height
def passable(self, pos):
return pos not in self.walls
def neighbors(self, pos):
x, y = pos
results = [(x+1, y), (x, y-1), (x-1, y), (x, y+1)]
if (x + y) % 2 == 0: results.reverse() # aesthetics
results = filter(self.in_bounds, results)
results = filter(self.passable, results)
return results
def cost(self, current, next):
return 1
def heuristic(a, b):
return abs(a[0] - b[0]) + abs(a[1] - b[1])
def astar(graph, start, goal):
frontier = []
heapq.heappush(frontier, (0, start))
came_from = {}
cost_so_far = {}
came_from[start] = None
cost_so_far[start] = 0
while frontier:
current = heapq.heappop(frontier)[1]
if current == goal:
break
for next in graph.neighbors(current):
new_cost = cost_so_far[current] + graph.cost(current, next)
if next not in cost_so_far or new_cost < cost_so_far[next]:
cost_so_far[next] = new_cost
priority = new_cost + heuristic(goal, next)
heapq.heappush(frontier, (priority, next))
came_from[next] = current
return came_from, cost_so_far
```
这个代码示例实现了一个基于栅格地图的A*算法,其中Grid类表示地图,heuristic函数表示启发式函数,astar函数表示A*算法的实现。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
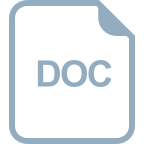
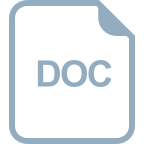
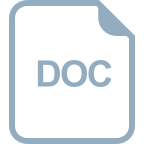




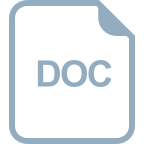
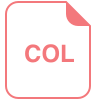
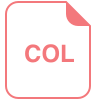








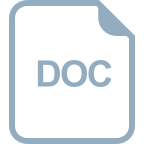