N = len(x) V = np.zeros(N)
时间: 2023-11-24 07:05:19 浏览: 78
这是一段Python代码,它的作用是创建一个长度为N的一维数组V,并且将数组中的所有元素初始化为0。其中,N是变量x的长度。该代码需要使用NumPy库,因此需要事先导入该库,例如:
```python
import numpy as np
x = [1, 2, 3, 4, 5] # 自定义变量x
N = len(x) # 获取x的长度
V = np.zeros(N) # 创建长度为N的数组V,并将其中所有元素初始化为0
print(V) # 输出数组V的值
```
在上面的代码中,我们首先定义了一个变量x,用于存储一些自定义的数值。然后,我们使用len()函数计算变量x的长度N。接着,使用np.zeros()函数创建一个长度为N的一维数组V,并将其中所有元素初始化为0。最后,我们输出数组V的值,即可看到所有元素都为0的数组。
相关问题
def out_to_rgb(out,PALETTE,CLASSES): palette = np.array(PALETTE) assert palette.shape[0] == len(CLASSES) assert palette.shape[1] == 3 assert len(palette.shape) == 2 color_seg = np.zeros((out.shape[0], out.shape[1], 3), dtype=np.uint8) for label, color in enumerate(palette): color_seg[out == label, :] = color tran=transforms.ToTensor() color_seg=tran(color_seg) return color_seg def out_to_rgb_np(out,PALETTE,CLASSES): palette = np.array(PALETTE) assert palette.shape[0] == len(CLASSES) assert palette.shape[1] == 3 assert len(palette.shape) == 2 color_seg = np.zeros((out.shape[0], out.shape[1], 3), dtype=np.uint8) for label, color in enumerate(palette): color_seg[out == label, :] = color return color_seg逐句解释
这段代码定义了两个函数,都是用于将一个模型输出的标签图像转换为 RGB 彩色图像。
- `def out_to_rgb(out,PALETTE,CLASSES):`:定义了一个名为 out_to_rgb 的函数,接受三个参数:out 表示模型输出的标签图像,PALETTE 表示颜色调色板,CLASSES 表示类别列表。
- `palette = np.array(PALETTE)`:将 PALETTE 转换为 numpy 数组,并将其赋值给变量 palette。
- `assert palette.shape[0] == len(CLASSES)`:断言 PALETTE 中的行数与 CLASSES 的长度相等,即每个类别对应了一种颜色。
- `assert palette.shape[1] == 3`:断言 PALETTE 中每个颜色由三个通道组成,即为 RGB 格式。
- `assert len(palette.shape) == 2`:断言 PALETTE 是一个二维数组。
- `color_seg = np.zeros((out.shape[0], out.shape[1], 3), dtype=np.uint8)`:创建一个 shape 为 (out.shape[0], out.shape[1], 3) 的全 0 numpy 数组,用于存储转换后的 RGB 彩色图像。
- `for label, color in enumerate(palette):`:遍历颜色调色板 PALETTE,获取每种颜色以及其对应的标签值。
- `color_seg[out == label, :] = color`:将标签图像中值为 label 的像素的 RGB 值赋为 color。
- `tran=transforms.ToTensor()`:创建一个 torchvision.transforms.ToTensor() 的实例,用于将 numpy 数组转换为 torch.Tensor。
- `color_seg=tran(color_seg)`:将经过转换后的 numpy 数组 color_seg 转换为 torch.Tensor,并将其赋值给变量 color_seg。
- `return color_seg`:返回转换后的 RGB 彩色图像,类型为 torch.Tensor。
- `def out_to_rgb_np(out,PALETTE,CLASSES):`:定义了一个名为 out_to_rgb_np 的函数,与 out_to_rgb 函数的实现基本相同,只是最后直接返回 numpy 数组类型的 RGB 彩色图像。
import numpy as np import sys from torchvision import transforms sys.path.append("..") def out_to_rgb(out,PALETTE,CLASSES): palette = np.array(PALETTE) assert palette.shape[0] == len(CLASSES) assert palette.shape[1] == 3 assert len(palette.shape) == 2 color_seg = np.zeros((out.shape[0], out.shape[1], 3), dtype=np.uint8) for label, color in enumerate(palette): color_seg[out == label, :] = color tran=transforms.ToTensor() color_seg=tran(color_seg) return color_seg def out_to_rgb_np(out,PALETTE,CLASSES): palette = np.array(PALETTE) assert palette.shape[0] == len(CLASSES) assert palette.shape[1] == 3 assert len(palette.shape) == 2 color_seg = np.zeros((out.shape[0], out.shape[1], 3), dtype=np.uint8) for label, color in enumerate(palette): color_seg[out == label, :] = color return color_seg
这段代码看起来是一个图像分割的函数,输入是一个经过模型预测得到的标签图像 out,以及 PALETTE 和 CLASSES 两个参数,PALETTE 是一个颜色调色板,每个类别都对应一种颜色;CLASSES 是一个类别列表,每个元素对应一个类别。输出是一个 RGB 图像,其中每个像素的颜色根据其对应的类别在 PALETTE 中取得。
其中 out_to_rgb_np 函数是不使用 torchvision.transforms.ToTensor 转换的版本,返回的是 numpy 数组类型的 RGB 图像。而 out_to_rgb 函数则是使用了 ToTensor 转换,返回的是 torch.Tensor 类型的 RGB 图像。
阅读全文
相关推荐
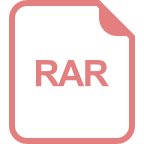
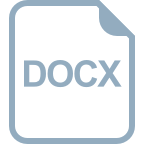
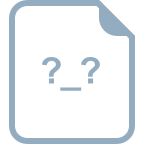













