编写C语言试在邻接组阵存储结构上实现图的基本操作: Insert Vex ( G , v) , Insert Arc (G,v,w), DeleteVex(G,v )和 DeleteArc(G ,v ,w )。
时间: 2023-10-10 17:14:56 浏览: 36
好的,我来为您解答这个问题。
在邻接矩阵存储结构上实现图的基本操作,可以使用以下结构体表示:
```c
#define MAX_VERTEX_NUM 20
typedef char VexType;
typedef int ArcType;
typedef struct {
VexType vexs[MAX_VERTEX_NUM]; // 存储顶点信息
ArcType arcs[MAX_VERTEX_NUM][MAX_VERTEX_NUM]; // 存储边的信息
int vexnum, arcnum; // 存储顶点数和边数
} AdjMatrix;
```
其中,顶点信息使用`VexType`类型表示,边的信息使用`ArcType`类型表示。`vexnum`和`arcnum`分别表示图中顶点数和边数。
接下来,我们就可以实现四种基本操作:
1. Insert Vex ( G , v)
```c
void InsertVex(AdjMatrix *G, VexType v) {
if (G->vexnum >= MAX_VERTEX_NUM) {
printf("Error: The graph is full!\n");
return;
}
G->vexs[G->vexnum] = v;
for (int i = 0; i < G->vexnum; i++) {
G->arcs[i][G->vexnum] = 0;
G->arcs[G->vexnum][i] = 0;
}
G->vexnum++;
}
```
2. Insert Arc (G,v,w)
```c
void InsertArc(AdjMatrix *G, VexType v, VexType w) {
int vpos = -1, wpos = -1;
for (int i = 0; i < G->vexnum; i++) {
if (G->vexs[i] == v) {
vpos = i;
}
if (G->vexs[i] == w) {
wpos = i;
}
if (vpos != -1 && wpos != -1) {
break;
}
}
if (vpos == -1 || wpos == -1) {
printf("Error: The vertex does not exist!\n");
return;
}
G->arcs[vpos][wpos] = 1;
G->arcnum++;
}
```
3. DeleteVex(G,v)
```c
void DeleteVex(AdjMatrix *G, VexType v) {
int vpos = -1;
for (int i = 0; i < G->vexnum; i++) {
if (G->vexs[i] == v) {
vpos = i;
break;
}
}
if (vpos == -1) {
printf("Error: The vertex does not exist!\n");
return;
}
for (int i = 0; i < G->vexnum; i++) {
if (i != vpos) {
G->arcs[i][vpos] = 0;
G->arcs[vpos][i] = 0;
}
}
for (int i = vpos; i < G->vexnum - 1; i++) {
G->vexs[i] = G->vexs[i+1];
}
G->vexnum--;
for (int i = 0; i < G->arcnum; i++) {
if (G->arcs[i][vpos] == 1 || G->arcs[vpos][i] == 1) {
for (int j = i; j < G->arcnum - 1; j++) {
G->arcs[j][vpos] = G->arcs[j+1][vpos];
G->arcs[vpos][j] = G->arcs[vpos][j+1];
}
G->arcnum--;
i--;
}
}
}
```
4. DeleteArc(G,v,w)
```c
void DeleteArc(AdjMatrix *G, VexType v, VexType w) {
int vpos = -1, wpos = -1;
for (int i = 0; i < G->vexnum; i++) {
if (G->vexs[i] == v) {
vpos = i;
}
if (G->vexs[i] == w) {
wpos = i;
}
if (vpos != -1 && wpos != -1) {
break;
}
}
if (vpos == -1 || wpos == -1) {
printf("Error: The vertex does not exist!\n");
return;
}
if (G->arcs[vpos][wpos] == 0) {
printf("Error: The arc does not exist!\n");
return;
}
G->arcs[vpos][wpos] = 0;
G->arcnum--;
}
```
以上代码为邻接矩阵存储结构上实现图的基本操作,其中`MAX_VERTEX_NUM`为最大顶点数,可以根据需要修改。
相关推荐
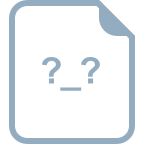













