tensorflow实现高斯模糊
时间: 2023-10-29 21:32:01 浏览: 236
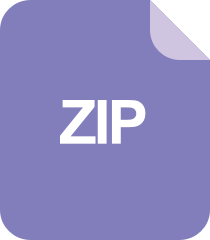
图像融合 图像处理(卷积、高斯模糊)
以下是使用TensorFlow实现高斯模糊的示例代码:
```python
import tensorflow as tf
import numpy as np
def gaussian_kernel(size, sigma):
x, y = np.meshgrid(np.linspace(-1,1,size), np.linspace(-1,1,size))
d = np.sqrt(x*x+y*y)
kernel = np.exp(-((d**2)/(2.0*sigma**2)))
kernel = kernel / np.sum(kernel)
return kernel
def apply_gaussian_blur(image, kernel_size, sigma):
# Create Gaussian kernel
kernel = gaussian_kernel(kernel_size, sigma)
# Reshape the kernel to match the expected shape of tf.nn.conv2d
kernel = kernel.reshape([kernel_size, kernel_size, 1, 1])
# Create a TensorFlow graph
graph = tf.Graph()
with graph.as_default():
# Create placeholders for the input and output images
input_image = tf.placeholder(tf.float32, shape=image.shape, name='input_image')
output_image = tf.placeholder(tf.float32, shape=image.shape, name='output_image')
# Create a convolutional layer with the Gaussian kernel
conv_layer = tf.nn.conv2d(input_image, kernel, strides=[1, 1, 1, 1], padding='SAME')
# Define the loss function as the mean squared error between the output image and the blurred image
loss = tf.reduce_mean(tf.square(output_image - conv_layer))
# Create an optimizer to minimize the loss
optimizer = tf.train.AdamOptimizer(learning_rate=0.01).minimize(loss)
# Initialize all variables
init = tf.global_variables_initializer()
# Run the TensorFlow graph
with tf.Session(graph=graph) as session:
# Initialize variables
session.run(init)
# Train the network for 1000 iterations
for i in range(1000):
_, l = session.run([optimizer, loss], feed_dict={input_image: image, output_image: image})
if i % 100 == 0:
print('Iteration %d, loss = %f' % (i, l))
# Apply the Gaussian blur to the input image
blurred_image = session.run(conv_layer, feed_dict={input_image: image})
return blurred_image
# Load an example image
image = tf.keras.utils.get_file('elephant.jpg', 'https://storage.googleapis.com/download.tensorflow.org/example_images/elephant.jpg')
image = tf.keras.preprocessing.image.load_img(image)
image = tf.keras.preprocessing.image.img_to_array(image)
# Apply Gaussian blur to the image
blurred_image = apply_gaussian_blur(image, kernel_size=5, sigma=1.5)
# Display the original and blurred images
import matplotlib.pyplot as plt
plt.subplot(1, 2, 1)
plt.imshow(image.astype('uint8'))
plt.title('Original Image')
plt.subplot(1, 2, 2)
plt.imshow(blurred_image.astype('uint8'))
plt.title('Blurred Image')
plt.show()
```
该代码使用`gaussian_kernel`函数创建高斯卷积核,并使用`apply_gaussian_blur`函数将该卷积核应用于输入图像。在TensorFlow图中,使用`tf.nn.conv2d`函数创建一个卷积层,并使用均方误差作为损失函数。使用Adam优化器最小化损失函数,并通过运行图来应用高斯模糊。
在示例中,我们使用了一个名为“elephant.jpg”的示例图像,并将其应用于内核大小为5和标准差为1.5的高斯模糊。结果显示了原始图像和模糊图像。
请注意,该示例仅用于演示目的,实际应用中可能需要对内核大小和标准差进行调整以获得最佳结果。
阅读全文
相关推荐
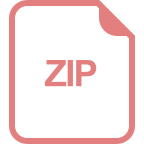
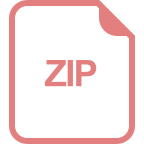















