matlab俄罗斯方块代码
时间: 2023-08-31 19:05:22 浏览: 68
以下是一个基于 MATLAB 的俄罗斯方块游戏的示例代码:
```matlab
% 俄罗斯方块游戏
clc;
clear all;
close all;
% 初始化游戏界面
board_width = 10;
board_height = 20;
board = zeros(board_height, board_width);
% 定义俄罗斯方块形状
shapes = {
[1 1 1 1], % I形状
[1 1 0; 0 1 1], % Z形状
[0 1 1; 1 1 0], % 反Z形状
[1 0 0; 1 1 1], % L形状
[0 0 1; 1 1 1], % 反L形状
[1 1; 1 1], % 方块形状
[0 1 0; 1 1 1] % T形状
};
% 随机选择一个形状
current_shape = shapes{randi(length(shapes))};
% 初始化形状位置
row = 1;
col = ceil(board_width / 2) - ceil(size(current_shape, 2) / 2);
while true % 游戏循环
% 显示游戏界面
display_board(board, current_shape, row, col);
% 等待用户输入
input_char = getkey;
% 处理用户输入
switch input_char
case 'leftarrow' % 左移
if is_valid_move(board, current_shape, row, col - 1)
col = col - 1;
end
case 'rightarrow' % 右移
if is_valid_move(board, current_shape, row, col + 1)
col = col + 1;
end
case 'downarrow' % 下移
if is_valid_move(board, current_shape, row + 1, col)
row = row + 1;
else
% 将形状放置到游戏界面中
board = place_shape(board, current_shape, row, col);
% 生成新的形状
current_shape = shapes{randi(length(shapes))};
% 初始化形状位置
row = 1;
col = ceil(board_width / 2) - ceil(size(current_shape, 2) / 2);
% 检查是否有可消除的行
board = clear_lines(board);
% 如果游戏结束,退出游戏循环
if is_game_over(board)
break;
end
end
case 'uparrow' % 旋转
rotated_shape = rot90(current_shape);
if is_valid_move(board, rotated_shape, row, col)
current_shape = rotated_shape;
end
case 'escape' % 退出游戏
break;
end
end
% 显示游戏结束信息
disp('Game Over!');
% 以下是一些辅助函数
function display_board(board, current_shape, row, col)
% 显示游戏界面
display_str = '';
for i = 1:size(board, 1)
for j = 1:size(board, 2)
if board(i, j) == 0
display_str = [display_str ' '];
else
display_str = [display_str '#'];
end
end
display_str = [display_str '\n'];
end
% 显示当前形状
for i = 1:size(current_shape, 1)
for j = 1:size(current_shape, 2)
if current_shape(i, j) == 1
display_str((row + i - 2) * (size(board, 2) + 1) + col + j - 1) = '#';
end
end
end
% 显示游戏界面和当前形状
clc;
fprintf(display_str);
end
function is_valid = is_valid_move(board, shape, row, col)
% 判断形状是否可以移动到指定位置
is_valid = true;
for i = 1:size(shape, 1)
for j = 1:size(shape, 2)
if shape(i, j) == 1
if row + i - 1 > size(board, 1) || col + j - 1 < 1 || col + j - 1 > size(board, 2) || board(row + i - 1, col + j - 1) == 1
is_valid = false;
return;
end
end
end
end
end
function new_board = place_shape(board, shape, row, col)
% 将形状放置到游戏界面中
new_board = board;
for i = 1:size(shape, 1)
for j = 1:size(shape, 2)
if shape(i, j) == 1
new_board(row + i - 1, col + j - 1) = 1;
end
end
end
end
function new_board = clear_lines(board)
% 检查是否有可消除的行
lines_to_clear = [];
for i = 1:size(board, 1)
if all(board(i, :) == 1)
lines_to_clear(end+1) = i;
end
end
% 消除行并将上面的行下移
new_board = board;
for i = lines_to_clear
new_board(i, :) = 0;
new_board(2:i, :) = new_board(1:i-1, :);
end
end
function is_game_over = is_game_over(board)
% 判断游戏是否结束
is_game_over = any(board(1, :) == 1);
end
```
这个示例代码可能有一些不完善的地方,但应该足够给你提供一个基本的思路。你可以根据自己的需要进行修改和扩展。
相关推荐
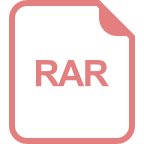









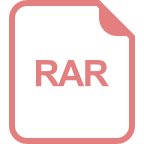