first集和follow集怎么求
时间: 2024-06-12 13:11:37 浏览: 38
在编译原理中,First集和Follow集是用于构造语法分析表的两个重要概念。
First集:对于文法中的每个非终结符,求出它能推导出的所有字符串的首符号集合,这个集合就是该非终结符的First集。
Follow集:对于文法中的每个非终结符,求出它在所有产生式右部中紧随其后的符号的集合,这个集合就是该非终结符的Follow集。
求解First集和Follow集的方法如下:
1. 对于每个终结符,它的First集就是它本身。
2. 对于每个非终结符,如果它可以推导出空串,则将空串加入它的First集。
3. 对于每个产生式A->α,将α的首符号加入A的First集。
4. 对于每个非终结符A,将所有能推导出A的产生式右部的Follow集加入A的Follow集。
5. 对于每个产生式A->αBβ,将B的First集加入A->αBβ的Follow集。如果B可以推导出空串,则将A的Follow集加入A->αBβ的Follow集。
相关问题
求first集和follow集c
关于求first集和follow集,需要先确定文法的产生式规则。以下是求解过程:
假设文法的产生式规则为:
S -> AB | bB
A -> aA | ε
B -> cB | d
首先求解非终结符A的first集:
- A -> aA | ε
- first(aA) = {a}
- 如果A可以推导出ε,则将ε加入first(A)中
- first(A) = {a, ε}
然后求解非终结符B的first集:
- B -> cB | d
- first(cB) = {c}
- first(d) = {d}
- first(B) = {c, d}
接下来求解非终结符S的first集:
- S -> AB | bB
- 如果S可以推导出A,则将first(A)中除ε以外的所有元素加入first(S)中
- first(A)中除ε以外的所有元素为{a}
- first(S) = {a}
- 如果S可以推导出B,则将first(B)中除ε以外的所有元素加入first(S)中
- first(B)中除ε以外的所有元素为{c, d}
- first(S) = {a, c, d}
最后求解非终结符A和B的follow集:
- S是开始符号,将$加入follow(S)中
- follow(S) = {$}
- 对于产生式A -> aA | ε,将follow(A)加入到aA的后面
- follow(A) = {b, c, d, $}
- 如果A可以推导出ε,则将follow(S)加入到follow(A)中
- follow(A) = {b, c, d, $}
- 对于产生式B -> cB | d,将follow(B)加入到cB和d的后面
- follow(B) = {b}
- 如果B可以推导出ε,则将follow(A)加入到follow(B)中
- follow(B) = {b, b, c, d, $}
因此,求解得到的first集和follow集如下:
- first(S) = {a, c, d}
- first(A) = {a, ε}
- first(B) = {c, d}
- follow(S) = {$}
- follow(A) = {b, c, d, $}
- follow(B) = {b, b, c, d, $}
求first集和follow集c语言
### 回答1:
First集和Follow集是语法分析中的概念,是用于判断一个文法是否为LL(1)文法的重要工具。
First集是指一个非终结符号能推导出的所有符号的首字母集合。
Follow集是指在文法中,在一个非终结符号之后的符号集合。
对于C语言来说,要计算First集和Follow集,需要对C语言的语法进行分析,计算每一个非终结符号的First集和Follow集。
### 回答2:
First集是指一个文法中每个非终结符对应的产生式右侧的第一个符号的集合。在C语言中,可以通过以下步骤求出每个非终结符的First集合:
1. 如果一个非终结符的产生式右侧第一个符号是终结符,则该终结符就是该非终结符的First集合中的元素。
2. 如果一个非终结符的产生式右侧第一个符号是另一个非终结符,则将该非终结符的First集合中的元素添加到当前非终结符的First集合中。
3. 如果一个非终结符的产生式右侧第一个符号可以推导出空串,则需要考虑后面的符号。如果后面的符号是终结符,则将该终结符加入该非终结符的First集合中;如果后面的符号是非终结符,则将该非终结符的First集合中去掉空串的元素添加到当前非终结符的First集合中。
Follow集是指文法中每个非终结符在任意一个派生式中“后面”可能出现的终结符的集合。在C语言中,可以通过以下步骤求出每个非终结符的Follow集合:
1. 对于文法起始符号S,将“$”加入S的Follow集中。
2. 对于每一个非终结符A,遍历文法中每个产生式,将“后面”可能出现在A之后的符号(包括终结符和非终结符)的First集合中除去空串的元素加入A的Follow集中。
3. 如果一个非终结符B可以推导出空串,即存在一个产生式右侧只包含空串的产生式,那么将B的Follow集加入至B所在产生式左侧非终结符的Follow集中。
通过求解First和Follow集合,可以完成C语言语法分析的相关操作。
### 回答3:
首先,需要先了解什么是first集和follow集。
first集:给定一个文法的某个非终结符,它所有能够推导出来的字符串中的第一个终结符集合,即每个产生式右边的终结符或空串的集合。
follow集:给定一个文法的某个非终结符,所有可能出现在它后面的终结符集合,即考虑这个非终结符在右侧是否还有其他非终结符或者是这个非终结符后面是否还能够推导出来空串。
接下来,我们以以下的C语言的产生式为例,来看如何求解first集和follow集。
S → F;
S → if(E)S;
S → if(E)S else S;
F → id=E;
F → ε;
E → EEQ
E → int
E → id
首先,我们来求解S的first集:
- S → F;,F是一个非终结符,在F的first集中,包含了终结符id和空串ε,然后将继续对;进行计算。
- S → if(E)S;,if是终结符,而E的first集中包含int和id,所以if的first集中也包含int和id。然后继续对(E)S进行计算,(和E相同,仅包含int和id,)的first集包含int和id,S的first集是它的first集和else的first集之和,由于S可以推导出空串,所以在这里也会包含空串ε。
- S → if(E)S else S;,if的first集中包含int和id,E计算出来也是int和id,)\E的first集是int和id,S的first集是它的第一个产生式的F的first集和else的first集之和。
- F → id=E;,id的first集是它本身,而E的first集中包含int和id,=的first集是它本身,F的first集为id。
- F → ε,F的first集是空集合。
然后,我们来求解S的follow集:
- S → F;,S的follow集包含文法中结束符$。
- S → if(E)S,假设A是它的follow集,则E的follow集是A,S的follow集是它后面的一个终结符else的first集和A的并集。由于S可以推导出空串,所以还需要加上它的前面的非终结符的follow集,即F的follow集。所以这里S的follow集即为else和$。
- S → if(E)S else S,同上,A是它的follow集,则E的follow集为else和A的并集,第一个S的follow集即为A,第二个S的follow集则是它后面的终结符$。
- F → id=E;,同上,E的follow集即为;的first集,F的follow集即为S的follow集。
- F → ε,F的follow集即为S的follow集。
接下来,我们来求解E的first集和follow集:
- E → EEQ,E的first集为int和id。
- E → int,E的first集为int。
- E → id,E的first集为id。
- E的follow集即为它后面的终结符}和else的first集。由于E在这个文法中出现的时候都是在if语句中,所以也需要加上if的follow集,即else和$。
最后,我们来求解if的first集和follow集:
- if的first集为if。
- if的follow集为else和$。
综上所述,我们可以通过算法,计算出文法中每个非终结符的first集和follow集,这可以被用于语法分析、错误检查、文法的优化等方面。
相关推荐
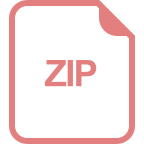
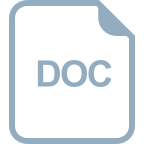
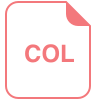











