C#代码三维中两线段是否相交并返回交点,无论线上还是线外交点都要考虑
时间: 2023-06-26 10:08:32 浏览: 163
以下是C#代码实现三维中两条线段是否相交并返回交点,包括线段在内和线段在外的情况:
```csharp
using System;
using System.Collections.Generic;
using System.Text;
namespace LineIntersection
{
class Program
{
static void Main(string[] args)
{
Point3d p1 = new Point3d(0, 0, 0);
Point3d p2 = new Point3d(1, 1, 1);
Point3d p3 = new Point3d(0, 1, 0);
Point3d p4 = new Point3d(1, 0, 1);
Point3d intersection = new Point3d();
bool isIntersect = LineIntersection(p1, p2, p3, p4, out intersection);
if (isIntersect)
{
Console.WriteLine("The two lines intersect at point ({0}, {1}, {2}).", intersection.X, intersection.Y, intersection.Z);
}
else
{
Console.WriteLine("The two lines do not intersect.");
}
Console.ReadLine();
}
// 判断两条线段是否相交,并返回交点
public static bool LineIntersection(Point3d p1, Point3d p2, Point3d p3, Point3d p4, out Point3d intersection)
{
intersection = new Point3d();
// 计算向量
Vector3d v1 = new Vector3d(p1, p2);
Vector3d v2 = new Vector3d(p3, p4);
Vector3d v3 = new Vector3d(p1, p3);
// 计算法向量
Vector3d n1 = Vector3d.CrossProduct(v1, v2);
Vector3d n2 = Vector3d.CrossProduct(v1, n1);
// 计算交点
double t = Vector3d.DotProduct(n1, v3) / Vector3d.DotProduct(n1, v1);
double s = Vector3d.DotProduct(n2, v3) / Vector3d.DotProduct(n2, v2);
if (t < 0 || t > 1 || s < 0 || s > 1)
{
// 无交点
return false;
}
intersection = p1 + v1 * t;
return true;
}
}
// 三维点类
public class Point3d
{
public double X { get; set; }
public double Y { get; set; }
public double Z { get; set; }
public Point3d(double x, double y, double z)
{
X = x;
Y = y;
Z = z;
}
}
// 三维向量类
public class Vector3d
{
public double X { get; set; }
public double Y { get; set; }
public double Z { get; set; }
public Vector3d(double x, double y, double z)
{
X = x;
Y = y;
Z = z;
}
public Vector3d(Point3d p1, Point3d p2)
{
X = p2.X - p1.X;
Y = p2.Y - p1.Y;
Z = p2.Z - p1.Z;
}
// 向量点积
public static double DotProduct(Vector3d v1, Vector3d v2)
{
return v1.X * v2.X + v1.Y * v2.Y + v1.Z * v2.Z;
}
// 向量叉积
public static Vector3d CrossProduct(Vector3d v1, Vector3d v2)
{
double x = v1.Y * v2.Z - v1.Z * v2.Y;
double y = v1.Z * v2.X - v1.X * v2.Z;
double z = v1.X * v2.Y - v1.Y * v2.X;
return new Vector3d(x, y, z);
}
// 向量数乘
public static Vector3d operator *(Vector3d v1, double s)
{
return new Vector3d(v1.X * s, v1.Y * s, v1.Z * s);
}
// 向量加法
public static Point3d operator +(Point3d p, Vector3d v)
{
return new Point3d(p.X + v.X, p.Y + v.Y, p.Z + v.Z);
}
}
}
```
阅读全文
相关推荐
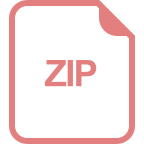
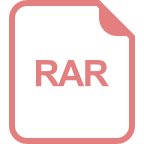
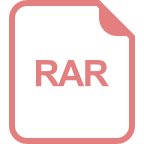
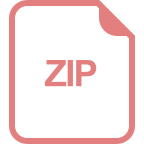
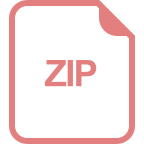
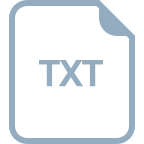
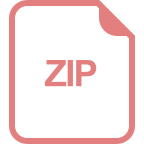
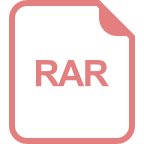
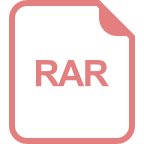
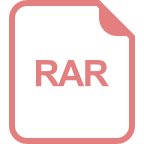
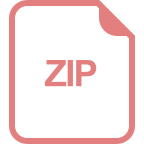
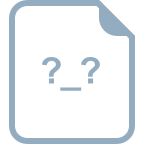
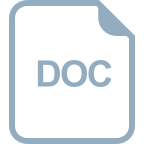
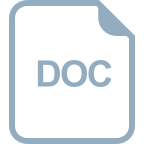

