unity里Dictionary<string, string> myDictionary = new Dictionary<string, string>(); myDictionary.Add("key1", "value1"); myDictionary.Add("key2", "value2"); myDictionary.Add("key3", "value3");如何获取字典里的内容?
时间: 2023-07-03 18:18:52 浏览: 36
要获取Dictionary中的内容,可以使用以下代码:
1. 获取单个元素:
```
string value = myDictionary["key1"];
```
2. 遍历所有元素:
```
foreach (KeyValuePair<string, string> kvp in myDictionary)
{
Debug.Log("Key = " + kvp.Key + ", Value = " + kvp.Value);
}
```
其中,kvp是一个KeyValuePair类型的变量,它包含了Dictionary中的键和值。在上述代码中,我们使用foreach循环遍历了所有的键值对,并打印输出了它们的键和值。
相关问题
unity这样public static Dictionary<string, string> tionary = new Dictionary<string, string>();定义了一个字典,并且在当前脚本赋值,在其他脚本引用却是空的,为什么?
如果在当前脚本中定义了一个 public static 字典,并在该脚本中赋值,但在其他脚本中引用该字典却为空,有可能是以下几个原因:
1. 赋值时机不对:如果在其他脚本中引用该字典的时候,当前脚本中的赋值操作还没有执行,那么字典就会为空。可以通过在 Awake 或 Start 方法中赋值来保证赋值时机的正确性。
2. 脚本执行顺序不对:Unity 中每个脚本都有执行的顺序,如果当前脚本的执行顺序在其他脚本之后,那么其他脚本在引用该字典时,当前脚本中的赋值还未执行,导致字典为空。可以通过编辑器中的“Script Execution Order”来调整脚本的执行顺序。
3. 字典被重置了:如果在其他脚本中对该字典进行了赋值或者清空操作,那么原本赋值的字典就会被重置为空。可以通过在当前脚本的 OnDisable 或 OnDestroy 方法中保存字典的状态,或者使用单例模式来保证字典的不被重置。
以下是使用单例模式共享字典对象的示例代码:
```csharp
// DictionaryManager.cs
using System.Collections.Generic;
using UnityEngine;
public class DictionaryManager : MonoBehaviour
{
public static DictionaryManager Instance { get; private set; }
public Dictionary<string, string> dict = new Dictionary<string, string>();
private void Awake()
{
// 实现单例模式
if (Instance == null)
{
Instance = this;
DontDestroyOnLoad(gameObject);
}
else
{
Destroy(gameObject);
}
// 初始化字典
dict.Add("apple", "red");
dict.Add("banana", "yellow");
dict.Add("orange", "orange");
}
}
// OtherScript.cs
using UnityEngine;
public class OtherScript : MonoBehaviour
{
private void Start()
{
// 获取字典
var dict = DictionaryManager.Instance.dict;
// 输出字典中的内容
foreach (var kvp in dict)
{
Debug.Log(kvp.Key + ": " + kvp.Value);
}
}
}
```
在上述代码中,定义了一个 DictionaryManager 类,其中包含一个名为 dict 的字典对象。在 Awake() 方法中,实现了单例模式,并且初始化了字典。在 OtherScript 类中,通过 DictionaryManager.Instance 获取单例对象,并访问其中的 dict 字典对象,最后输出了字典中的内容。这样就可以在多个脚本中共享同一个字典对象了。
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; using UnityEngine.Events; public class Inventory : MonoBehaviour { public Button btn_pickup; public GameObject item; public Sprite[] sprites; public static class UIBind{ public static void BindClick(Button btn,UnityAction onClick) { btn.onClick.AddListener(onClick); } } private GameObject content; private Dictionary<string, int> itemsDic = new Dictionary<string, int>(); private Dictionary<string, GameObject> objsDic = new Dictionary<string, GameObject>(); void Start() { content = GameObject.Find("Canvas/Inventory/Viewport/Content"); UIBind.BindClick(btn_pickup, OnClickPickUp); } void OnClickPickUp() { Sprite sprite = sprites[Random.Range(0,sprites.Length)]; if (itemsDic.ContainsKey(sprite.name)) { itemsDic[sprite.name]++; objsDic[sprite.name].transform.Find("num").GetComponent<Text>().text = "x" + itemsDic[sprite.name].ToString(); } else { itemsDic.Add(sprite.name, 1); GameObject obj = Instantiate(item, content.transform); Image image = obj.transform.Find("slot").GetComponent<Image>(); image.sprite = sprite; Text text = obj.transform.Find("num").GetComponent<Text>(); text.text = "x" + itemsDic[sprite.name].ToString(); ; obj.GetComponentInChildren<Button>().onClick.AddListener(delegate() { OnClickUse(obj, sprite.name, text); }); objsDic.Add(sprite.name, obj); } } void OnClickUse(GameObject obj,string name ,Text text) { itemsDic[name]--; if (itemsDic[name] <=0) { itemsDic.Remove(name); objsDic.Remove(name); Destroy(obj); } else { text.text = "x" + itemsDic[name].ToString(); } } }麻烦帮我注释并且修正下
下面是对代码的注释和修正:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.Events;
public class Inventory : MonoBehaviour
{
// 拾取按钮
public Button btn_pickup;
// 物品预制体
public GameObject item;
// 所有物品的图片
public Sprite[] sprites;
// 静态内部类,用于绑定按钮点击事件
public static class UIBind
{
public static void BindClick(Button btn, UnityAction onClick)
{
btn.onClick.AddListener(onClick);
}
}
// 物品栏的内容
private GameObject content;
// 存储物品数量的字典
private Dictionary<string, int> itemsDic = new Dictionary<string, int>();
// 存储物品对象的字典
private Dictionary<string, GameObject> objsDic = new Dictionary<string, GameObject>();
void Start()
{
// 获取物品栏的内容对象
content = GameObject.Find("Canvas/Inventory/Viewport/Content");
// 绑定拾取按钮的点击事件
UIBind.BindClick(btn_pickup, OnClickPickUp);
}
// 点击拾取按钮的事件处理函数
void OnClickPickUp()
{
// 随机选择一张物品图片
Sprite sprite = sprites[Random.Range(0, sprites.Length)];
if (itemsDic.ContainsKey(sprite.name))
{
// 如果已经有该物品,则数量加1
itemsDic[sprite.name]++;
objsDic[sprite.name].transform.Find("num").GetComponent<Text>().text = "x" + itemsDic[sprite.name].ToString();
}
else
{
// 如果没有该物品,则添加新物品
itemsDic.Add(sprite.name, 1);
// 创建新的物品对象
GameObject obj = Instantiate(item, content.transform);
// 设置物品图片
Image image = obj.transform.Find("slot").GetComponent<Image>();
image.sprite = sprite;
// 设置物品数量
Text text = obj.transform.Find("num").GetComponent<Text>();
text.text = "x" + itemsDic[sprite.name].ToString();
// 绑定物品使用按钮的点击事件
obj.GetComponentInChildren<Button>().onClick.AddListener(delegate ()
{
OnClickUse(obj, sprite.name, text);
});
objsDic.Add(sprite.name, obj);
}
}
// 点击物品使用按钮的事件处理函数
void OnClickUse(GameObject obj, string name, Text text)
{
// 物品数量减1
itemsDic[name]--;
if (itemsDic[name] <= 0)
{
// 如果数量为0,则从字典和界面上移除该物品
itemsDic.Remove(name);
objsDic.Remove(name);
Destroy(obj);
}
else
{
// 否则更新物品数量
text.text = "x" + itemsDic[name].ToString();
}
}
}
```
相关推荐
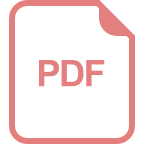
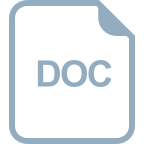












