写一个程序,功能是将一个文件copy给另一个文件,用标准CI/0实现
时间: 2024-10-16 07:20:24 浏览: 25
为了通过标准C++ I/O流实现简单的文件复制功能,你可以创建一个名为`CopyFile.cpp`的源文件,编写如下的代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
// 定义一个函数用于复制文件
void copy_file(const std::string& source_path, const std::string& destination_path) {
std::ifstream in(source_path, std::ios::binary); // 二进制模式打开读取
if (!in) {
std::cerr << "Error opening source file: " << source_path << std::endl;
return;
}
std::ofstream out(destination_path, std::ios::binary); // 二进制模式打开写入
if (!out) {
std::cerr << "Error opening destination file: " << destination_path << std::endl;
in.close(); // 关闭已打开的输入流
return;
}
char buffer[4096]; // 临时缓冲区大小
while (in.read(buffer, sizeof(buffer))) { // 逐块读取并写入
out.write(buffer, in.gcount()); // 写入到输出文件
}
// 检查是否已完全读取
if (in.gcount() != 0) {
std::cerr << "Error reading from source file." << std::endl;
in.clear(); // 清除错误状态
in.seekg(0, std::ios::beg); // 移动回文件开始位置
} else {
in.close();
}
out.close(); // 关闭输出流
}
int main() {
std::string source = "path_to_source.txt";
std::string destination = "path_to_destination.txt"; // 替换为你需要的实际路径
try {
copy_file(source, destination);
std::cout << "File copied successfully." << std::endl;
} catch (const std::exception &e) {
std::cerr << "An error occurred: " << e.what() << std::endl;
}
return 0;
}
```
在这个程序中,我们首先尝试打开源文件并读取,然后逐块写入目标文件。如果遇到任何错误,比如文件无法打开或读写过程中出现问题,我们会捕获异常并输出错误信息。
运行此程序前,请确保替换`source`和`destination`变量为你实际要操作的源文件和目标文件路径,并确保目标文件路径可以安全覆盖。
阅读全文
相关推荐
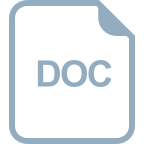
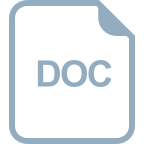
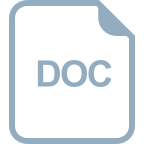

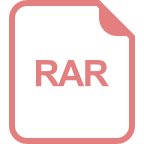













