设以带头结点的双向链表表示的线性表为L=(a1, a2,…, an),试设计一时间复杂度为O(n)的算法,将L改造为L=(a1,a3,…,an…, a4, a2)。C语言编程及算法流程图
时间: 2024-10-05 16:05:06 浏览: 4
为了将一个带头结点的双向链表 L = (a1, a2, ..., an) 改造为 L' = (a1, a3, ..., an, ..., a4, a2),我们可以采用以下步骤:
1. 创建一个新的头结点 new_head,并将其指向前一个列表的第一个元素 a1。
2. 初始化两个指针 current 和 previous,分别指向 L 的当前元素和上一个元素(如果有的话)。初始时,current 指向 a1,previous 指向 null。
3. 遍历链表,直到遇到最后一个元素。在遍历时:
a. 当 current 的下一个元素 next 满足条件(即 next 的值等于 current 的值加 2),则交换 current 和 next 的指针,将 current 移动到 next(因为我们要跳过当前位置的节点)。
b. 更新 current 为 current 的下一个节点(如果不是 last,则 next 永远不会满足条件,因此 current 自然会移到下一对相邻节点)。
c. 如果 current 到达了 end(即 next 为空),那么将其赋值给 previous 的下一个指针,然后 previous 赋值为 current。
4. 最后,返回新的头结点 new_head,它现在指向的是原链表的一个非连续序列。
以下是 C 语言的伪代码示例:
```c
typedef struct Node {
int data;
struct Node* prev;
struct Node* next;
} Node;
Node* reverseEven(Node* head) {
Node* new_head = new Node();
new_head->next = head;
Node* current = head;
Node* previous = NULL;
while (current != NULL && current->next != NULL) {
if (current->next->data == current->data + 2) {
// Swap the pointers
Node* temp = current->next;
current->next = temp->next;
temp->next = current;
if (previous != NULL)
previous->next = temp;
else
new_head = temp;
current = current->next;
} else {
previous = current;
current = current->next;
}
}
// If the last node is odd, connect it to the beginning
if (current != NULL)
current->prev->next = current;
return new_head;
}
```
相关推荐
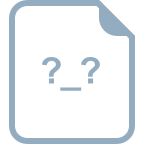
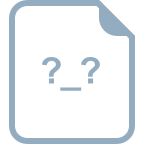
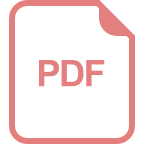






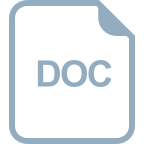
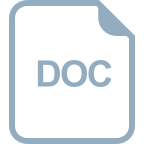
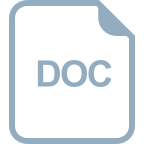
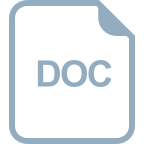


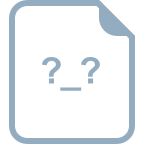
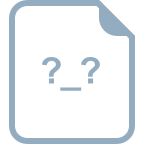