设以带头结点的双向循环链表表示的线性表L=(a1,a2,•••,an)。试写一时间复杂度为O(n)的算法,将L改造为L=(a1,a3,…,an,…, a 4, a2) .
时间: 2024-10-05 19:03:59 浏览: 42
这个算法的主要目标是将给定的双向循环链表`L`中的元素按照交替顺序排列,即奇数位置的节点保持不变,偶数位置的节点移动到前面。我们可以利用头结点来辅助操作,因为双向链表允许我们从头部和尾部同时遍历。
以下是步骤描述:
1. 初始化三个指针,`prev`指向头结点,`current`指向头结点的下一个节点,`next`指向当前节点的下一个节点。
2. 当`current`不是最后一个节点时,进入循环:
- 将`current`的下一个节点赋值给`temp`,这是为了临时保存`current`的下一个节点。
- 将`current`的下一个节点设置为`current`,使得链表的偶数位置节点链接在一起。
- 将`current`更新为其原本的下一个节点,即`temp`,继续处理下一个偶数位置节点。
- 如果`current`是头结点,则将`next`设回`current`,形成新的循环。
3. 循环结束后,`current`会停在新链表的最后,所以更新头结点为`current`。
时间复杂度为O(n),因为我们只遍历了一次链表。
```python
# Python伪代码
class Node:
def __init__(self, data):
self.data = data
self.prev = None
self.next = None
def alternate_order(head):
if head is None or head.next is None:
return head
current = head
next_node = current.next
temp = None
while True:
# Swap even position nodes
if temp is not None:
current.next = temp
temp.prev = current
else:
current.next = next_node
next_node.prev = current
# Move to the next odd position
current = next_node
if current == head:
break
next_node = current.next
# Update head for the new cycle
head.prev = head.next
head.next.prev = head
head.next = current
current.prev = head
return head
```
阅读全文
相关推荐
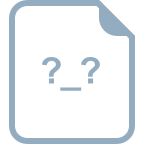
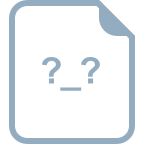
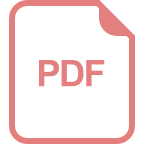









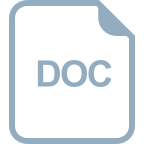


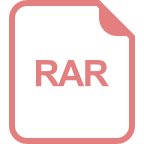
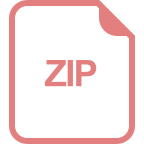
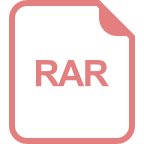
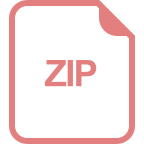